Javascript Calendar Example
Calendars are a very useful widget or part of an application, as we can see from the frequency in which we use them ourselves (at least for the forgetful people like me!). In fact they can be so useful that there are currently apps dedicated solely to this function in our smartphones and other gadgets. How can we create one ourselves using Javascript? Let’s see below!
1. Things to consider before creating a calendar
We decided to work on creating our own Javascript Calendar. But where exactly do we start? Let’s see!
What we need is a calendar that displays correctly the number of days in a month and also the weekday to which it corresponds, so the best view we could give it is the regular table, the classic one. For that we would need a piece of code that would do the correct rendering and presenting of our code.
Moreover we would need a correct Javascript function that displays the correct number of days for a month (remember that February is a leap year!), the correct weekday for it and also for the first day of the month.
Lastly, we would want our calendar to be presentable, since that is crucial to the accurate understanding of it. To fulfill all these requirements, we will divide our code into three parts: the HTML, the CSS and most importantly the Javascript. Let’s get to it!
2. The HTML Part
Firstly, we have to create an HTML document that will be the base of the calendar we’re building. As you can see in the code below, it will be very basic, containing only a div
element with the id calendar
, which we will be using later in other parts of our code. Take a look at the code below:
calendar.html
<!DOCTYPE html> <html> <head> <title>JavaScript calendar</title> <script src="calendar.js"></script> <link href="calendar.css" rel="stylesheet"/> </head> <body onload="displayCalendar()"> <div id="calendar"></div> </body> </html>
The only additions to the code that we didn’t already mention are the linking and scripting of the Javascript and CSS part of our code, as we decided to put them into separate files to make our code more easily understandable and less clustered, and also the function displayCalendar()
which we have put inside the body
tag. This way, whenever our document is loaded, through the onload
event, the function will be executed immediately and we will have our calendar displayed nicely in it. However, the HTML part is just this small thing. Impressive, right?
3. The Javascript Part
Admittedly, Javascript is the most important part in making an accurate and dynamic calendar. That doesn’t mean that it is an especially difficult part, though. Before we get to the code, we have to mention that we will base our work largely on a built in Javascript object, that is the Date
object.
3.1 Global Variables
This object parses a given date and gives us useful information about that date. If we leave the argument unspecified, it will accept the current date as one by default. However, the Date
object has it’s limitations, some of them being that it doesn’t know the names of the months or days of the week, the lengths of the months (and consequently the leap year limitations), or the day of the week in which the month starts.
These “flaws” of the Date
object will be compensated by the global variables that we will be using in our code. Let’s take a look at the code part by part.
calendar.js
var htmlContent =""; var FebNumberOfDays =""; var counter = 1;
We will have a number of global variables during the course of our code, but the ones above are the first ones, and they are used as simple helpers. The first one, htmlContent
is used for the HTML content we will generate later on, while FebNumberOfDays
is used to store the number of days that the second month of the year, February, is going to last. We need only the length of this month as a variable as it is the only one that changes from one year to the other. Next up is a counter that will help us later on. Now that we’re done with this, take a look at the code snippet below:
calendar.js
var dateNow = new Date(); var month = dateNow.getMonth(); var day = dateNow.getDate(); var year = dateNow.getFullYear();
3.2 Fixing the shortcomings of built-in features
As you see, here we have used the Date
object to get the current date and also relevant information about it such as the month, day and year to which it corresponds. Below you will find a code snippet that specifies the previous and next month, which will be useful later in order to determine the right weekday in which the month starts.
calendar.js
var nextMonth = month+1; var prevMonth = month-1;
Now let’s determine the number of days in February with the function shown below, which is also one of the easiest and most popular solutions online about this problem:
calendar.js
if (month == 1){ if ( (year%100!=0) && (year%4==0) || (year%400==0)){ FebNumberOfDays = 29; }else{ FebNumberOfDays = 28; } }
When we explained the Date
object, we mentioned that it had a few shortcomings that we would have to compensate for later. You might remember that some of them had to do with the labels for months and weekdays, and the lengths of each month. The code snippet below would solve these problems:
calendar.js
var monthNames = ["January","February","March","April","May","June","July","August","September","October","November", "December"]; var dayNames = ["Sunday","Monday","Tuesday","Wednesday","Thrusday","Friday", "Saturday"]; var dayPerMonth = ["31", ""+FebNumberOfDays+"","31","30","31","30","31","31","30","31","30","31"];
With the next part of code, we determine the days of the previous and next months and also the days of the week. The code would go like this:
calendar.js
var nextDate = new Date(nextMonth +' 1 ,'+year); var weekdays= nextDate.getDay(); var weekdays2 = weekdays; var numOfDays = dayPerMonth[month];
3.3 Displaying the Calendar
The loop shown in the code snippet below will make it possible for your calendar to have white spaces in the dates of the previous month:
calendar.js
while (weekdays>0){ htmlContent += "<td class='monthPre'></td>"; weekdays--; }
We wrote all the code for the logic behind our Calendar. What is left now is only building our calendar’s body using HTML. We will do that by using the code snippet below:
calendar.js
while (counter <= numOfDays){ if (weekdays2 > 6){ weekdays2 = 0; htmlContent += "</tr><tr>"; } if (counter == day){ htmlContent +="<td class='dayNow' onMouseOver='this.style.background=\"#FFFF00\"; this.style.color=\"#FFFFFF\"' "+ "onMouseOut='this.style.background=\"#FFFFFF\"; this.style.color=\"#00FF00\"'>"+counter+"</td>"; }else{ htmlContent +="<td class='monthNow' onMouseOver='this.style.background=\"#FFFF00\"'"+ " onMouseOut='this.style.background=\"#FFFFFF\"'>"+counter+"</td>"; } weekdays2++; counter++; }
Inside the while cycle you will see that the first conditional clause is the one that is used to determine when we will have a new line, while the rest of the code highlights the current date.
Let’s top it all off by creating the HTML body of the calendar using the code below:
calendar.js
var calendarBody = "<table class='calendar'> <tr class='monthNow'><th colspan='7'>" +monthNames[month]+" "+ year +"</th></tr>"; calendarBody +="<tr class='dayNames'> <td>Sun</td> <td>Mon</td> <td>Tues</td>"+ "<td>Wed</td> <td>Thurs</td> <td>Fri</td> <td>Sat</td> </tr>"; calendarBody += "<tr>"; calendarBody += htmlContent; calendarBody += "</tr></table>"; document.getElementById("calendar").innerHTML=calendarBody;
All this code will be placed inside a function that we named displayCalendar
, if you’ll remember it’s name from the HTML part in the first part of the article. With this, we’re done.
4. The CSS Part
Even though we are finished with the most part of our calendar, we have to put in a few touches of CSS lest it look completely and utterly ugly and difficult to understand too, for that matter. This is why, being the passionate people we are about style, we use the code snippet below to make our calendar look more presentable:
calendar.css
.monthPre{ color: gray; text-align: center; } .monthNow{ color: blue; text-align: center; } .dayNow{ border: 2px solid black; color: #FF0; text-align: center; } .calendar td{ htmlContent: 2px; width: 40px; } .monthNow th{ background-color: #000000; color: #FFFFFF; text-align: center; } .dayNames{ background: #0FF000; color: #FFFFFF; text-align: center; }
The calendar we built will look like below:
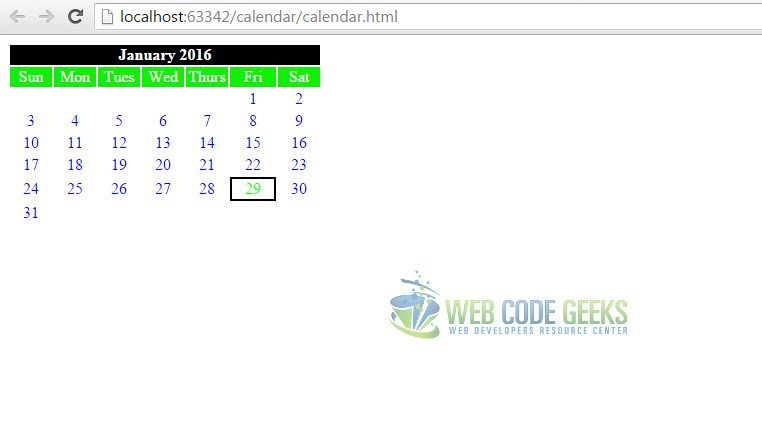
And with this, you now have you calendar, accurate and pretty. However, don’t refrain from experimenting by yourself!
5. Download the source code
This was an example of Calendars in Javascript.
You can download the full source code of this example here: calendar
how start from Monday
Hi there, nicely done! What about an ISO calendar with week number in column 1 and Modays in column 2?
It seems that the array DayNames is redundant.
Hello Era – I love your calendar! I have put together a concept for traverse-able event and booking calendar, loosely based on this calendar. I thought you might be interested to know :)
https://github.com/alanbickel/booking-calendar. Please feel free to email me if you’ve got any feedback or concerns :)
Nice article, thanks for sharing! I have build one, check it out: https://jsuites.net/v3/javascript-calendar