JavaScript Date toString() Example
Greetings readers, in this tutorial, we will show how to use the JavaScript scripting language and the Date toString() method.
1. Introduction
JavaScript is an object-oriented programming language that allows the client-side scripting to interact with a user and deliver the dynamic pages. Most web browsers including Google Chrome, Mozilla Firefox, Safari, Internet Explorer, Microsoft Edge, Opera, etc. support it. The JavaScript scripting language includes:
- Declaring variables
- Maintaining the retrieving values
- Defining and invoking functions
- Defining classes
- Load and use external modules
- Define event handlers
- And much more ….
1.1 Advantages of JavaScript Language
The pros of using the JavaScript scripting language are:
- JavaScript is easy to learn
- It executes on client’s browser, so eliminates the server-side processing and be executed on any OS
- JavaScript can be used with any type of web page e.g. PHP, ASP.NET, Perl etc
- Web-page performance increases due to client-side execution
- JavaScript code can be minified to decrease the loading time from the server
- Many JavaScript-based application frameworks are available in the market to create Single page web applications e.g. AngularJS, ReactJS etc.
1.2 Disadvantages of JavaScript Language
The cons of using the JavaScript scripting language are:
- No support for networking applications
- Does not have any multi-threading or multi-processing capabilities
- Does not allow the file reading and writing capabilities
1.3 JavaScript Date toString() method
- JavaScript provides the
Date
object to work with Date and Time including days, months, years, hours, seconds and milliseconds - The
toString()
is an inbuilt method that converts aDate
object to a String representation - The
toString()
method is automatically called,- When the
Date
is represented as a text value. For e.g.console.log(new Date());
- When the
Date
is used in string concatenation. For e.g.var today = 'Today is ' + new Date();
- When the
- The
toString()
method represents a generic method, it does not require that it’sthis
is aDate
instance - Syntax:
var demo = dateObj.toString();
2. JavaScript Date toString() Example
Here is a systematic guide for implementing this tutorial using the JavaScript language.
2.1 Tools Used
We are using Eclipse Kepler SR2, JDK 8 and Maven. Having said that, we have tested the code against JDK 1.7 and it works well.
2.2 Project Structure
Firstly, let us review the final project structure if you are confused about where you should create the corresponding files or folder later!
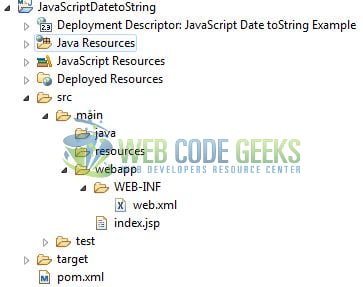
2.3 Project Creation
This section will show on how to create a Java-based Maven project with Eclipse. In Eclipse Ide, go to File -> New -> Maven Project
.
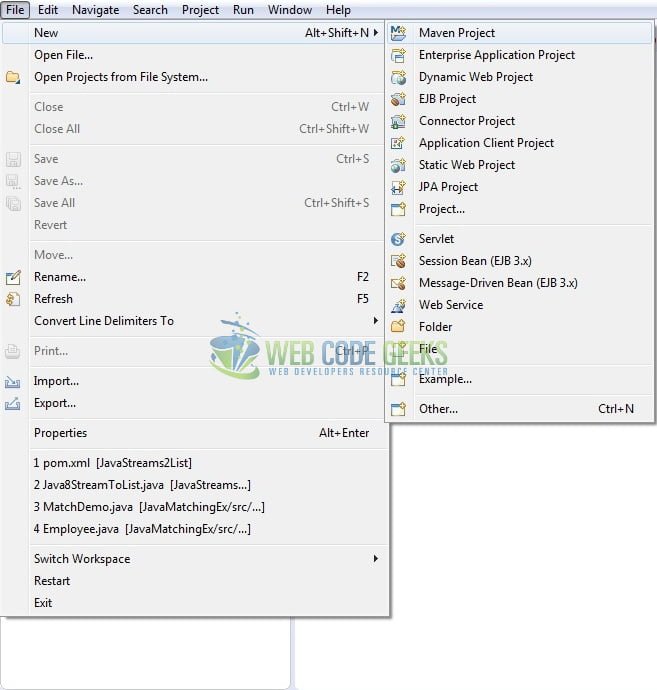
In the New Maven Project window, it will ask you to select the project location. By default, ‘Use default workspace location’ will be selected. Just click on the next button to proceed.
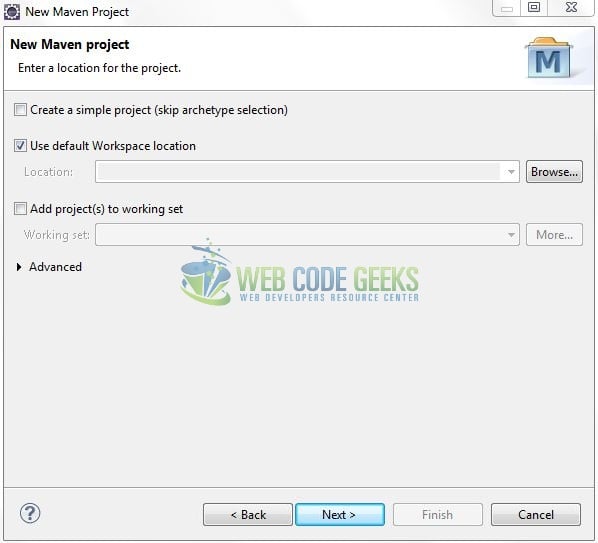
Select the ‘Maven Web App’ Archetype from the list of options and click next.
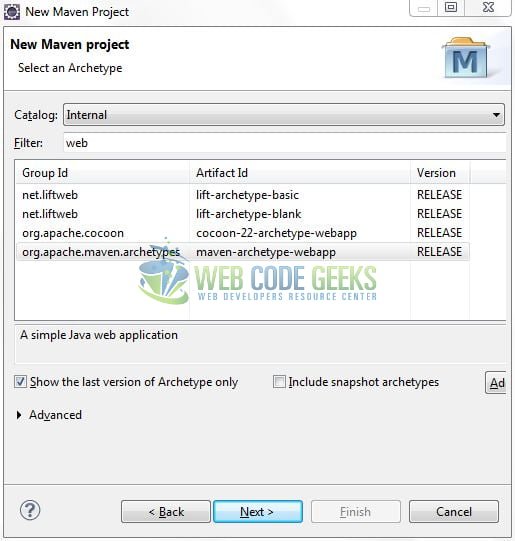
It will ask you to ‘Enter the group and the artifact id for the project’. We will input the details as shown in the below image. The version number will be by default: 0.0.1-SNAPSHOT
.
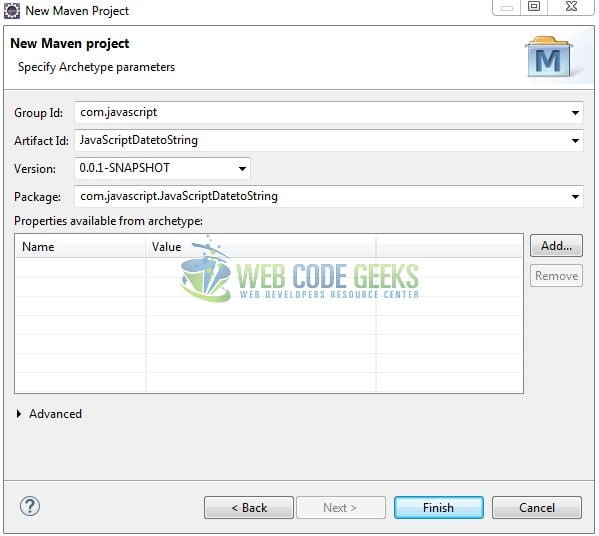
Click on Finish and the creation of a maven project is completed. If you see, it has downloaded the maven dependencies and a pom.xml
file will be created. It will have the following code:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.javascript</groupId> <artifactId>JavaScriptDatetoString</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> </project>
3. Application Building
Let us create an application to understand the basic building blocks of this tutorial.
3.1 Define the HTML
Let us write a simple index page in the JavaScriptDatetoString/src/main/webapp/
folder. Add the following code to it:
index.jsp
<!-- Date toString() function --> <div id="toString-info"> <h3 class="text-primary font-weight-bold">JavaScript Date</h3> <ol class="text-info line-hgt"> <li>JavaScript provides the <code>Date</code> object to work with <em>Date</em> and <em>Time</em> including days, months, years, hours, seconds and milliseconds.</li> <li>The <code>toString()</code> method converts a <code>Date</code> object to a String representation.</li> <li>The <code>toString()</code> method is automatically called, <ul> <li>When the <code>Date</code> is represented as a text-value. For e.g. <code>console.log(new Date());</code></li> <li>When the <code>Date</code> is used in string concatenation. For e.g. <code>var today = 'Today is ' + new Date();</code></li> </ul> </li> <li>The <code>toString()</code> method is a generic method, it does not require that it's <code>this</code> is a <code>Date</code> instance</li> <li>Syntax:<pre>var demo = dateObj.toString();</pre></li> </ol> </div> <div> </div> <!-- showing result --> <h5 id="result" class="text-success"></h5>
3.2 Define the JavaScript function
Let us write a simple javascript function that shows the execution of the toString()
function. Add the following code to it:
JavaScript function
var dateObj = new Date(); // Assigns a string value to 'today' in the same format as: Mon Sep 08 1998 14:36:22 GMT+0530 (India Standard Time). var today = dateObj.toString(); // assigning the output to the html. document.getElementById("result").innerHTML = "<strong>Today is</strong> " + today;
3.3 First application
Complete the above steps and save the file. Let us see the sample code snippet.
index.jsp
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Index page</title> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <link href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9zdGFja3BhdGguYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/4.1.3/css/bootstrap.min.css" rel="stylesheet"> <style> .line-hgt { line-height: 2.0; } </style> </head> <body> <div class="container"> <h2 align="center" class="text-danger">JavaScript Date <code>toString()</code> Example</h2> <hr /> <!-- Date toString() function --> <div id="toString-info"> <h3 class="text-primary font-weight-bold">JavaScript Date</h3> <ol class="text-info line-hgt"> <li>JavaScript provides the <code>Date</code> object to work with <em>Date</em> and <em>Time</em> including days, months, years, hours, seconds and milliseconds.</li> <li>The <code>toString()</code> method converts a <code>Date</code> object to a String representation.</li> <li>The <code>toString()</code> method is automatically called, <ul> <li>When the <code>Date</code> is represented as a text-value. For e.g. <code>console.log(new Date());</code></li> <li>When the <code>Date</code> is used in string concatenation. For e.g. <code>var today = 'Today is ' + new Date();</code></li> </ul> </li> <li>The <code>toString()</code> method is a generic method, it does not require that it's <code>this</code> is a <code>Date</code> instance</li> <li>Syntax:<pre>var demo = dateObj.toString();</pre></li> </ol> </div> <div> </div> <!-- showing result --> <h5 id="result" class="text-success"></h5> </div> </body> <script type="text/javascript"> var dateObj = new Date(); var today = dateObj.toString(); // Assigns a string value to 'today' in the same format as: Mon Sep 08 1998 14:36:22 GMT+0530 (India Standard Time). // Assigning the output to the html. document.getElementById("result").innerHTML = "<strong>Today is</strong> " + today; </script> </html>
4. Run the Application
As we are ready for all the changes, let us compile the project and deploy the application on the Tomcat7 server. To deploy the application on Tomat7, right-click on the project and navigate to Run as -> Run on Server
.
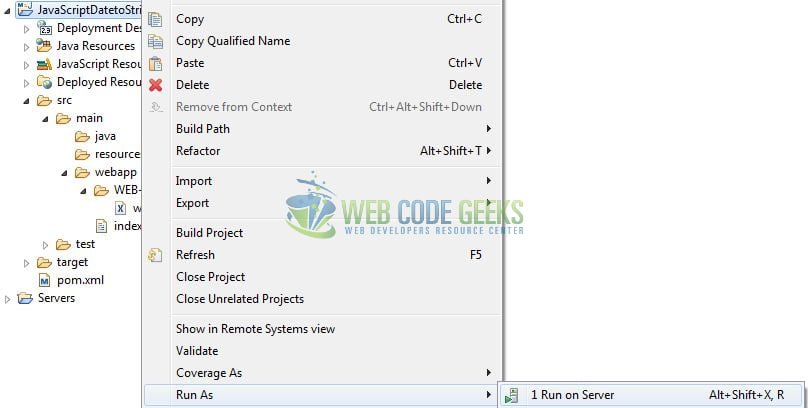
Tomcat will deploy the application in its web-apps folder and shall start its execution to deploy the project so that we can go ahead and test it in the browser.
5. Project Demo
Open your favorite browser and hit the following URL to display the application’s index page as shown in Fig. 7.
http://localhost:8082/JavaScriptDatetoString/
Server name (localhost) and port (8082) may vary as per your Tomcat configuration.
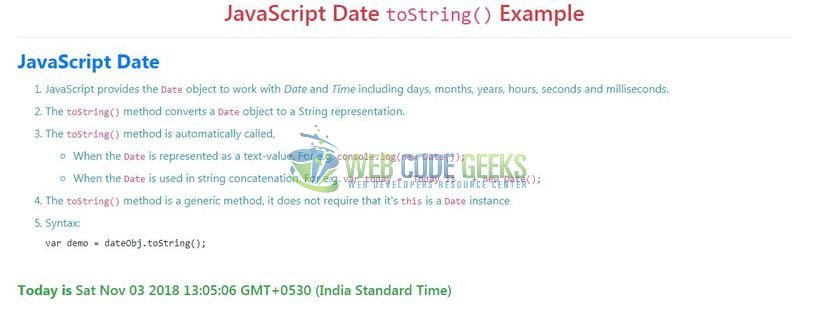
That is all for this tutorial and I hope the article served you whatever you were looking for. Happy Learning and do not forget to share!
6. Conclusion
In this section, developers learned how to create a simple application with the JavaScript language. Developers can download the sample application as an Eclipse project in the Downloads section.
7. Download the Eclipse Project
This was an example of JavaScript Date toString() function for the beginners.
You can download the full source code of this example here: JavaScriptDatetoString