Working with Oracle JET Chart Component
In this post, we’ll see how to use Oracle JET Chart (oj-chart) Component and its various properties. Here I am using Oracle JET with JDeveloper 12.1.3. To learn more about using JET with JDeveloper refer my previous post
Goto Oracle JET Cookbook and read the whole recipe of Bar Chart, Here you’ll learn about basic properties of a chart
Now open the dashboard.html file from your project in JDeveloper editor and paste this code
<div class="oj-hybrid-padding"> <h1>Dashboard Content Area</h1> <div> <oj-chart id="barChart" type="bar" orientation="[[orientationValue]]" stack="[[stackValue]]" series="[[barSeriesValue]]" groups="[[barGroupsValue]]" animation-on-display="auto" animation-on-data-change="auto" hover-behavior="dim" style="max-width:650px;width:100%;height:350px;"> </oj-chart> </div> </div>
Here you can see orientation, stack, series, groups values are referenced from different variables and these variables are used in the dashboard.js file to supply values to chart component.
/* * Your dashboard ViewModel code goes here */ define(['ojs/ojcore', 'knockout', 'jquery', 'ojs/ojchart'], function (oj, ko, $) { function DashboardViewModel() { var self = this; /*Chart Properties*/ self.stackValue = ko.observable('off'); self.orientationValue = ko.observable('vertical'); /* chart data */ var barSeries = [ {name : "Finance", items : [42000, 55000]}, {name : "Purchase", items : [55000, 70000]}, {name : "Service", items : [36000, 50000]}, {name : "Administration", items : [28000, 65000]}, {name : "HR", items : [25000, 60000]} ]; var barGroups = ["Average Salary", "Max Salary"]; self.barSeriesValue = ko.observableArray(barSeries); self.barGroupsValue = ko.observableArray(barGroups); . . .
Here you can see that an array is used to populate data in the bar chart and all variables are defined with appropriate values, You can see that we have two groups here for each Department and chart orientation is vertical.
Now run and check how it looks on the browser
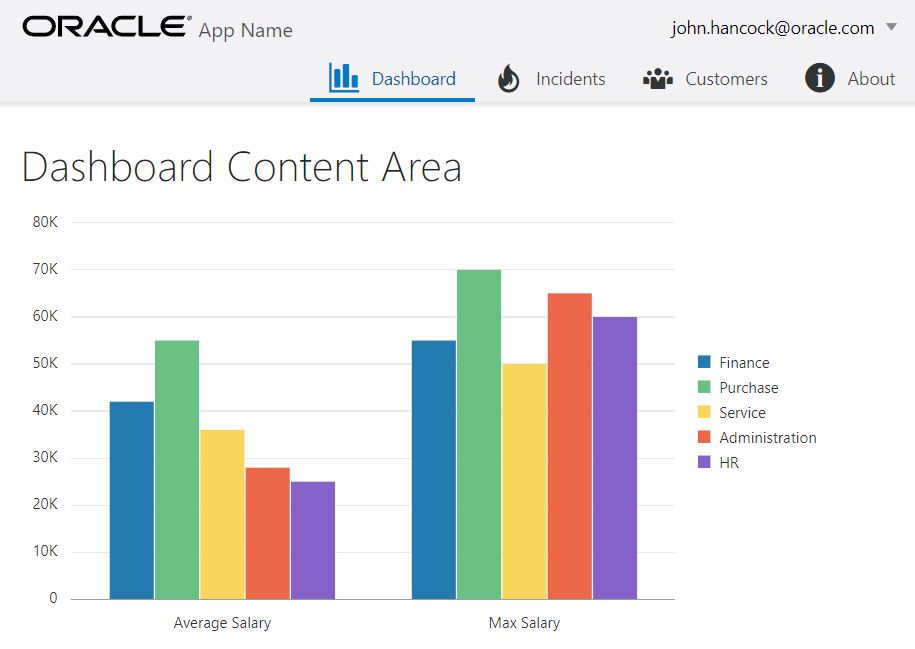
Try changing stackValue and orientationValue attribute and check what happens?
/*Chart Properties*/ self.stackValue = ko.observable('on'); self.orientationValue = ko.observable('horizontal');
and bar chart looks like this
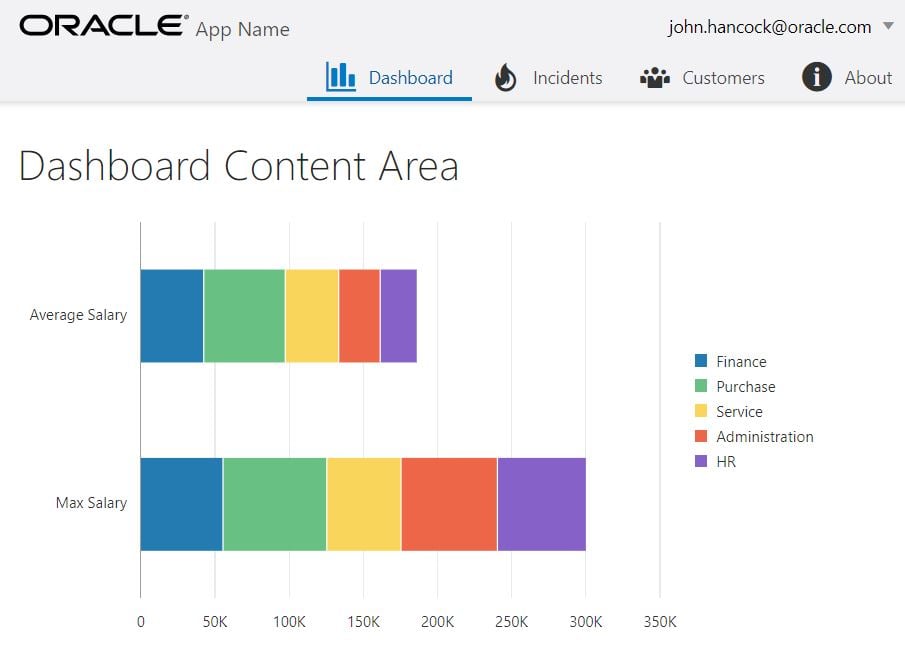
Now I am adding a choice list that shows different chart type and on the selection of specific type, the chart should change so for this requirement I am adding an oj-select-one component on the page and referencing chart’s type attribute to its value
This is the complete code of the dashboard.html
<div class="oj-hybrid-padding"> <h1>Dashboard Content Area</h1> <div> <oj-label for="basicSelect">Select Chart Type</oj-label> <oj-select-one id="basicSelect" value="{{chartType}}" style="max-width:20em"> <oj-option value="bar">Bar</oj-option> <oj-option value="pie">Pie</oj-option> <oj-option value="line">Line</oj-option> <oj-option value="area">Area</oj-option> </oj-select-one> <oj-chart id="barChart" type="[[chartType]]" orientation="[[orientationValue]]" stack="[[stackValue]]" series="[[barSeriesValue]]" groups="[[barGroupsValue]]" animation-on-display="auto" animation-on-data-change="auto" hover-behavior="dim" style="max-width:650px;width:100%;height:350px;"> </oj-chart> </div> </div>
and the dashboard.js file looks like this
/* * Your dashboard ViewModel code goes here */ define(['ojs/ojcore', 'knockout', 'jquery', 'ojs/ojchart','ojs/ojselectcombobox'], function (oj, ko, $) { function DashboardViewModel() { var self = this; //Setting choice list attribute this.chartType = ko.observable("bar"); /*Chart Properties*/ self.stackValue = ko.observable('off'); self.orientationValue = ko.observable('vertical'); /* chart data */ var barSeries = [ {name : "Finance", items : [42000, 55000]}, {name : "Purchase", items : [55000, 70000]}, {name : "Service", items : [36000, 50000]}, {name : "Administration", items : [28000, 65000]}, {name : "HR", items : [25000, 60000]} ]; var barGroups = ["Average Salary", "Max Salary"]; self.barSeriesValue = ko.observableArray(barSeries); self.barGroupsValue = ko.observableArray(barGroups); . . .
Now run and try changing chart type from the choice list
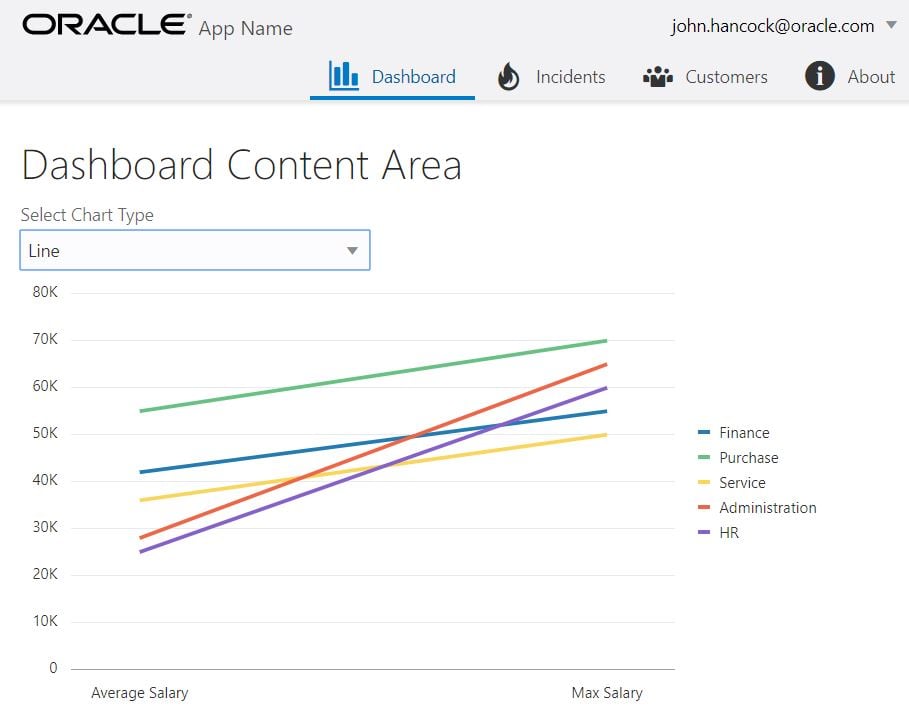
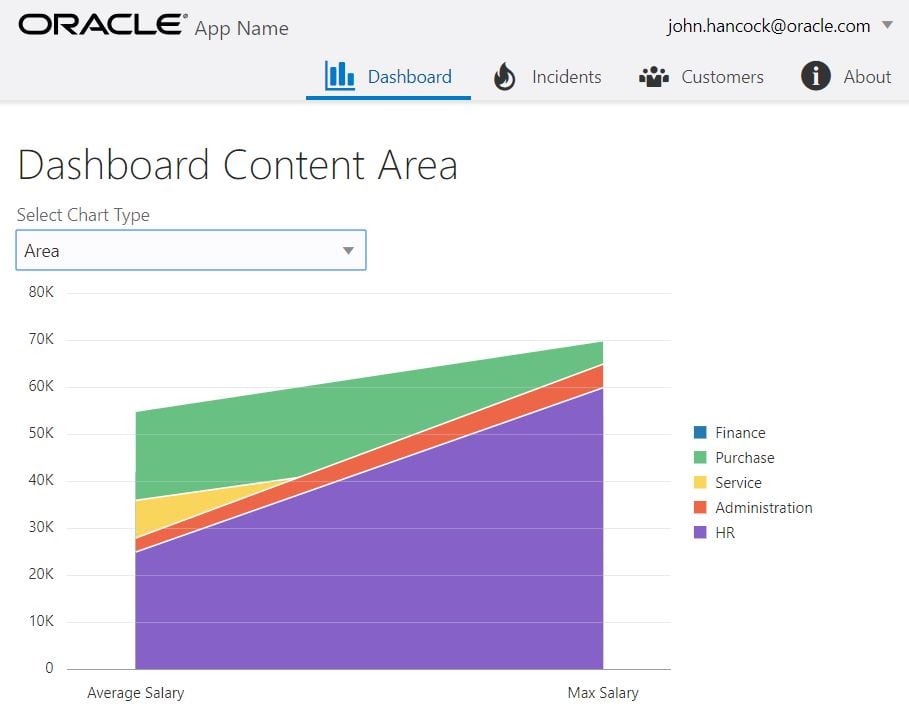
You can download the Sample Oracle JET Application here, its approx 13mb as JET library filed are included in the project
Cheers Happy Learning
Published on Web Code Geeks with permission by Ashish Awasthi, partner at our WCG program. See the original article here: Working with Oracle JET Chart Component Opinions expressed by Web Code Geeks contributors are their own. |