Oracle JET Common Model Factory Method to Consume REST Services
Previously I have posted about consuming web services in Oracle JET using jQuery method and using Oracle JET Common Model. I have seen Oracle JET Common Model Factory method in one of the blog and in MOOC course so tried same but because of some version change or problem it was not working as expected but I got a solution in OTN JET forum and sharing same here for reference.
Here I am using the same JET nav drawer template application that is used in my first post – Using Oracle JET with JDeveloper. and using ADF BC based REST web service
Add an oj-table component in the dashboard.html page and define the required number of columns to show Departments details.
<div class="oj-hybrid-padding"> <h1>Dashboard Content Area</h1> <div id="div1"> <oj-table id="table" summary="Department List" aria-label="Departments Table" data='[[datasource]]' columns='[{"headerText": "Department Id", "field": "DepartmentId"}, {"headerText": "Department Name", "field": "DepartmentName"}, {"headerText": "Location Id", "field": "LocationId"}, {"headerText": "Manager Id", "field": "ManagerId"}]'> </oj-table> </div> </div>
Next is to create a Factory JS file that holds the basic code for calling web service and read its response (Oracle JET Common Model and Collection is used in a separate file for better understanding of code )
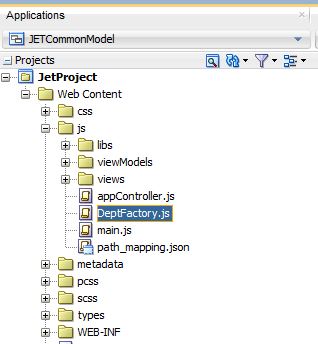
Here goes the code for DeptFactory.js
define(['ojs/ojcore'], function (oj) { var DeptFactory = { resourceUrl : 'http://127.0.0.1:7101/RestWebServApp-RESTWebService-context-root/rest/Jdev12.2.1/Department', // Single Department Model createDeptModel : function () { var dept = oj.Model.extend( { urlRoot : this.resourceUrl, idAttribute : "DepartmentId" }); return new dept(); }, // Departments Collection createDepartmentsCollection : function () { var departments = oj.Collection.extend( { url : this.resourceUrl, model : this.createDeptModel(), comparator : "DepartmentId" }); return new departments(); } }; return DeptFactory; });
And this Factory JS is used in dashboard.js View Model to populate data in the JET table
/** * @license * Copyright (c) 2014, 2019, Oracle and/or its affiliates. * The Universal Permissive License (UPL), Version 1.0 */ /* * Your dashboard ViewModel code goes here */ define(['ojs/ojcore', 'knockout', 'jquery', 'DeptFactory', 'ojs/ojtable', 'ojs/ojcollectiontabledatasource'], function (oj, ko, $, DeptFactory) { function DashboardViewModel() { var self = this; self.deptCollection = DeptFactory.createDepartmentsCollection(); self.datasource = ko.observable() // Setting collection in row and column format to show in JET table self.datasource(new oj.CollectionTableDataSource(this.deptCollection)); } return new DashboardViewModel; });
Now run application and check, Department details are populated in the table from web service
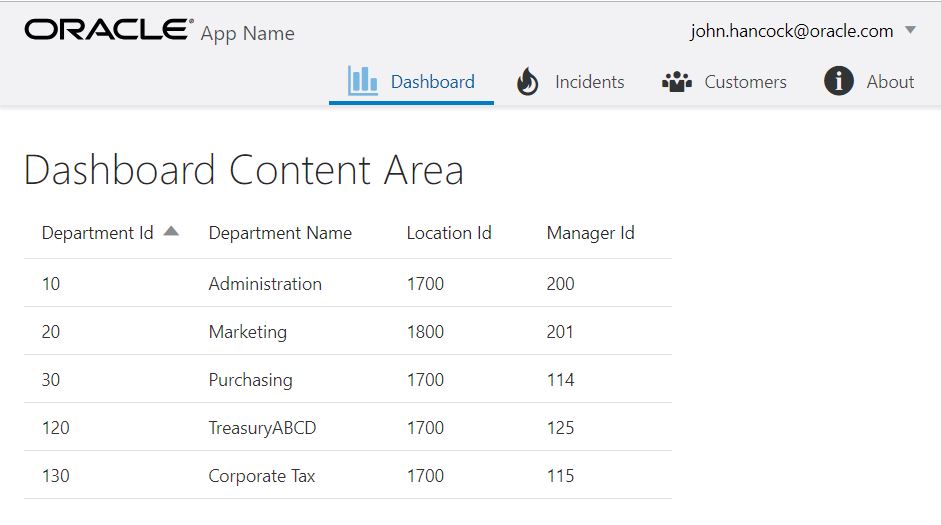
So this is how we can use Oracle JET Common Model Factory method to consume REST web services.
Cheers Happy Learning
Published on Web Code Geeks with permission by Ashish Awasthi, partner at our WCG program. See the original article here: Oracle JET Common Model Factory Method to Consume REST Services Opinions expressed by Web Code Geeks contributors are their own. |