PHP Checkbox Example
Checkboxes are those HTML elements that behave like toggle switches. So, unlike the radio buttons, checkboxes do not enforce to select an option from a group of options; we can select as much as we want. In this example, we will see how to deal with these checkboxes in PHP.
For this example, we will use:
- Linux Mint (17.03) as Operating System.
- Apache HTTP server (2.4.7).
- PHP (5.5.9).
You may skip environment preparation and jump directly to the beginning of the example below.
1. Preparing the environment
Below, commands to install Apache and PHP are shown:
sudo apt-get update sudo apt-get install apache2 php5 libapache2-mod-php5 sudo service apache2 restart
Note: if you want to use Windows, installing XAMPP is the fastest and easiest way to install a complete web server that meets the prerequisites.
2. HTML Form
Let’s see with a simple form how the checkboxes work:
form.html
<!DOCTYPE html> <head> <meta charset="UTF-8"> <title>HTML form</title> <style> html { width: 35%; } .form-input { margin-bottom: 20px; } </style> </head> <body> <form method="GET" action="process.php"> <div class="form-input"> <label for="name">Your name</label> <input type="text" name="name" id="name" required> </div> <div class="form-input"> <fieldset> <legend>Programming languages you have worked with</legend> <div> <input type="checkbox" name="programming-languages[]" id="python" value="Python"> <label for="python">Python</label> </div> <div> <input type="checkbox" name="programming-languages[]" id="php" value="PHP" checked> <label for="php">PHP</label> </div> <div> <input type="checkbox" name="programming-languages[]" id="haskell" value="Haskell"> <label for="haskell">Haskell</label> </div> <div> <input type="checkbox" name="programming-languages[]" id="other" value="Other"> <label for="other">Other</label> </div> </fieldset> </div> <div class="form-input"> <input type="submit" value="Submit"> </div> </form> </body> </html>
For which the following rendering will be generated:
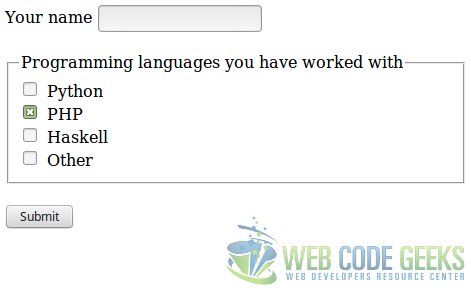
The are some things that we have to know:
- We can set the checkboxes checked from the beginning with the
checked
attribute (line 28). By default, the checkboxes are unchecked. - Note that each checkbox name ends with
[]
. This is for accessing the selected values of the checkboxes with the same name as an array. If we leave the same name but with the square brackets in the end, PHP will only be able to retrieve the last item. - HTML5 does not provide a standard way of marking a group of checkboxes as
required
. This can be achieved with JavaScript, but it’s not the aim of this example.
3. Form handling
The only difference in the form processing with other form elements is that PHP takes the elements of a checkbox group as an array, as previously mentioned.
But the way the data is submitted is not different from other elements. This is an example of the data generated for our GET
request:
process.php?name=Julen&programming-languages[]=PHP&programming-languages[]=Other
As you can see, there are two different values with the same name, which makes sense since we have chosen different values that share the name. But PHP will know that, when retrieving these values, it has to put them inside an array, because of the square brackets []
placed in the end of each parameter name.
So, processing this form data would be pretty simple, as shown in the following script:
process.php
<?php /** * Checks if the given parameters are set. If one of the specified parameters * is not set, die() is called. * * @param $parameters The parameters to check. */ function checkGETParametersOrDie($parameters) { foreach ($parameters as $parameter) { isset($_GET[$parameter]) || die("'$parameter' parameter must be set."); } } /** * Print the submitted data. If the value is an array, is imploded to a string. * * @param array $data The form data, with $name => $value format. */ function printData($data) { echo 'You have submitted the form with the following data:<br>'; echo '<ul>'; foreach ($data as $name => $value) { echo "<li>$name: "; if (is_array($value)) { $value = implode(', ', $value); } echo "<b>$value</b>"; echo '</li>'; } echo '</ul>'; } // Flow starts here. checkGETParametersOrDie([ 'name', 'programming-languages' ]); $name = $_GET['name']; $programmingLanguages = $_GET['programming-languages']; // An array. printData([ 'Name' => $name, 'Programming languages you have worked with' => $programmingLanguages ]);
The way of retrieving the data is exactly the same (line 46), but in this case we have to be aware that we are receiving an array.
Because of this, when printing the form data (or doing anything else), we have to know if we are dealing with an array or not. For this, we have to check explicitly if it is an array, with is_array()
function, to then manage it as appropriate. In this case, we just implode it to a string.
So, the script could generate an output like the following:
You have submitted the form with the following data:
- Name: Julen
- Programming languages you have worked with: PHP, Other
4. Generating checkbox structures dynamically
It’s worth mentioning how we could generate checkbox structures dynamically for a given data structure, that can be achieved with a script like the following:
generate_checkboxes.php
<?php /** * Generates checkboxes, for group => values => checked/unchecked structures, * wrapping each group in a fieldset. * * @param array $data The data to create the checkboxes for. * @return string The generated checkboxes. */ function generateCheckboxes($data) { $checkboxes = ''; foreach ($data as $group => $values) { $fieldset = $values['fieldset']; $options = $values['options']; $checkboxes .= '<fieldset>'; $checkboxes .= "<legend>$fieldset</legend>"; foreach ($options as $option => $checked) { $checked = ($checked) ? 'checked' : ''; $checkboxes .= '<div>'; $checkboxes .= "<input type='checkbox' name='$group" . '[]' . "' id='$option' value='$option' $checked>"; $checkboxes .= "<label for='$option'>$option</label>"; $checkboxes .= '</div>'; } $checkboxes .= '</fieldset>'; } return $checkboxes; }
Placing each checkbox group inside a fieldset (which is not actually necessary, just structure matters).
The following snippet shows an input data example for this function, which would generate the same output as the shown in section 2:
generate_radiobuttons.php
// ... $programmingLanguages = [ 'programming-languages' => [ 'fieldset' => 'Programming languages you have worked with', 'options' => [ 'Python' => false, 'PHP' => true, 'Haskell' => false, 'Other' => false ] ] ]; $checkboxes = generateCheckboxes($programmingLanguages); echo $checkboxes; echo 'Generated HTML code:<br>'; echo "<xmp>$checkboxes</xmp>";
5. Summary
In this example we have seen how to manage the checkbox elements with PHP, paying attention at the way of retrieving the values of a checkbox group, which is different from retrieving other input types, since they are defined as arrays. We have also seen how to generate checkbox structures dynamically with a simple PHP function that generates the HTML code from a data structure.
6. Download the source code
This was an example of checkboxes with PHP.
You can download the full source code of this example here: PHPCheckboxExample