PHP Text Area Example
When building forms, we use text type inputs when we want the user to provide some text. But, for when we expect larger, multi-line texts, we have available the text areas.
In this example we will see how to deal with these.
For this example, we will use:
- Linux Mint (17.03) as Operating System.
- Apache HTTP server (2.4.7).
- PHP (5.5.9).
You may skip environment preparation and jump directly to the beginning of the example below.
1. Preparing the environment
Below, commands to install Apache and PHP are shown:
sudo apt-get update sudo apt-get install apache2 php5 libapache2-mod-php5 sudo service apache2 restart
Note: if you want to use Windows, installing XAMPP is the fastest and easiest way to install a complete web server that meets the prerequisites.
2. HTML form
Let’s create a simple form having a text area:
form.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>PHP Text Area Example</title> <style> .form-input { margin-bottom: 20px; } </style> </head> <body> <form method="POST" action="process.php"> <div class="form-input"> <label for="name">Your name</label> <input type="text" name="name" id="name" required> </div> <div class="form-input"> <label for="text">Type any text</label> <div> <textarea name="text" id="text" rows="4" cols="35" required></textarea> </div> </div> <div> <input type="submit" value="Submit"> </div> </form> </body> </html>
The text areas are handled by the server as same as any other form element, but they are not an input
element, but they have an “own” HTML element, textarea
(line 21). Regardless this, they also have to have assigned a name
attribute.
3. Form handling
As we have said, the way of dealing with these inputs is the same as with any other. So, a script like the following is enough to process the form data:
process.php
<?php /** * Checks if the given parameters are set. If one of the specified parameters * is not set, die() is called. * * @param $parameters The parameters to check. */ function checkPOSTParametersOrDie($parameters) { foreach ($parameters as $parameter) { isset($_POST[$parameter]) || die("'$parameter' parameter must be set."); } } // Flow starts here. checkPOSTParametersOrDie([ 'name', 'text' ]); $name = $_POST['name']; $text = $_POST['text']; echo 'You have submitted the form with the following data:<br>'; echo '<ul>'; echo "<li><b>Name:</b> $name</li>"; echo "<li><b>Text:</b> $text</li>"; echo '</ul>';
Easy, right? The output generated by this file will be similar to the following:
You have submitted the form with the following data:
- Name: Julen
- Text: a random text.
4. Warning: this form is insecure
In fact, this is applicable to any type of text input, not only to text areas, but is worth mentioning here, since we are speaking about text inputs.
This form is vulnerable to XSS attacks. Try to submit the form with a value similar to the following (for any of the inputs):
<script>alert(1)</script>
You would see that the browser is actually executing the code:
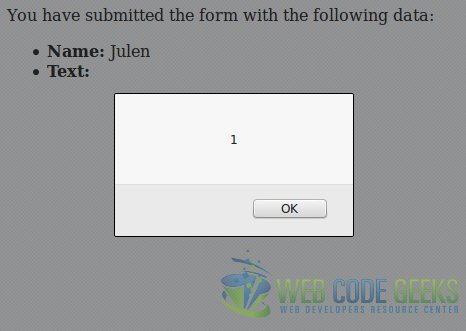
That is, an attacker could execute arbitrary code with our form. This “attack” seems harmless, but, in the worst of the cases, an attacker could use this form for hijacking sessions.
Fortunately, fixing this scary vulnerability in this form is pretty simple. Escaping the HTML metacharacters would be enough. This is achieved with htmlentities
() function. This function will convert the evil input we have seen above, to the following:
<script>alert(1)</script&>
Converting the HTML metacharacters to HTML entities.
So, we only have to change the lines where we retrieve the parameters:
process.php
// ... $name = htmlentities($_POST['name']); $text = htmlentities($_POST['text']); // ...
And the form would behave as expected:
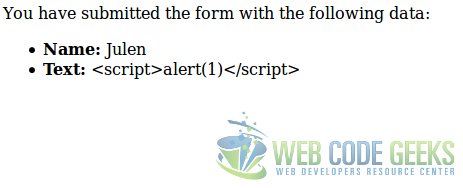
5. Summary
This example has shown how to manage the textarea
HTML element, which actually does not differ from other form elements, seeing also how to handle securely the form data to avoid XSS attacks (which is applicable to other text inputs, not only text areas).
6. Download the source code
This was an example of text areas with PHP.
You can download the full source code of this example here: PHPTextAreaExample