Python Tutorial: Strings Datatype
Data stored in memory can of different types and Python like other languages have different standard data types. Sometime back we did a post on
Python Numbers. Today we will be covering other standard datatypes i.e. Strings.
Note: All examples shown in the post are based on python3.
Like other languages, python also has the same meaning/definition for Strings.
They are a contiguous set of characters enclosed within single/double quotation marks.
#!/usr/bin/python strA = "Hello " strB = 'World!' #Printing the above variables on screen print strA #This will work in Python2 print strB #This will work in Python2 print( strA ) #This will work in Python3 print( strB ) #This will work in Python3
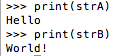
String Slicing:
Strings can be sliced i.e. subsets of a string, using the slice operator ([:] or []). The index starts from 0.
#!/usr/bin/python print( strA[0] ) #prints the first character of variable strA print( strA[1:3] ) #prints characters from first index to third print( strA[3:] ) #prints characters from third index
String Concatenation:
Like other languages python also provides the functionality to concatenate strings. It is done its the + operator.
#!/usr/bin/python print( "Print Concatenated Output: " + strA + strB )

If you try to concat another datatype using + operator, you would get an error ” cannot convert ‘int’ object to str implicitly“. So to achieve that we have two ways:
1. We can do by putting values using a comma inside print()
2. Other way, we can use an inbuilt function str(). This will convert any datatype to string thus, allowing us to use + operator
#!/usr/bin/python print( strA + 4 ) #This will give an error as mentioned above #Correct Way to Concat String and another Datatype print( strA, 4 ) #Method 1 print( strA + str(1234) ) #Method 2
Escape Characters:
The definition an escape character is a character which invokes an alternative interpretation on subsequent characters in a character sequence. It can be interpreted in a single as well as double quoted string.
Below is the list of escape characters with their description:
Some of the special operators
We only saw the + operator, but apart from this there are many others. Below is the list of all operators:
Formatting Operator
Formatting Operator %, is one of the features which reminded me of the time when I used to write code in C. Here in python it functions the same way:
Below is a list of formatting operators:
Example:
#!/usr/bin/python """You can have multiple formatting operators, but remember the sequence of variables must be followed after % inside a bracket () separated by comma""" num = 2 post_num = 129 print( "Code %s Learn" %num) print( "Code %s Learn\'s post number: %s" %(num,post_num))

You must have noticed that I have used Triple quotes in the above example. Triple quote is used for writing multi-line comments, whereas # is used for writing a single line comment.
Python also provides multiple built-in functions for String manipulations. Below is the gist of some functions:
Check Python Docs for detailed reference.
Reference: | Python Tutorial: Strings Datatype from our WCG partner Farhan Khwaja at the Code 2 Learn blog. |