AngularJS File Upload Example
Hello readers, in this tutorial, we will understand how to upload a file or files in the angular web applications. For this tutorial, we’ll create a simple form that has a file upload field and binds it to the angular controller to get the data from the form.
1. Introduction
Before we begin with the tutorial, let’s take a look at the Angular JavaScript and its characteristics.
1.1 Angular JavaScript
Angular is a JavaScript MVC or Model-View-Controller framework developed by Google that lets developers build well structured, easily testable, and maintainable front-end applications. But before we start creating a real application using the angular library, let us see what the important parts of an angular application are.
1.1.1 Templates
In Angular, a template is an HTML
with added markups. The angular library compiles the templates and renders the resultant HTML
page.
1.1.2 Directives
Directives are the markers (i.e. attributes) on a DOM element that tell angular to attach a specific behavior to that DOM element or even transform the DOM element and its children. Most of the directives in the angular library start with the ng
. The directives consist of the following parts:
ng-app
: Theng-app
directive is a starting point. If the angular framework finds theng-app
directive anywhere in theHTML
document, it bootstraps (i.e. initializes) itself and compiles theHTML
templateng-model
: Theng-model
directive binds anHTML
element to a property on the$scope
object. It also binds the values of angular application data to theHTML
input controlsng-bind
: Theng-bind
directive binds the angular application data to theHTML
tagsng-controller
: Theng-controller
directive specifies a controller in the HTML element. This controller will add behavior or support the data in that HTML element and its child elementsng-repeat
: Theng-repeat
directive repeat a set of HTML elements for each item in a given collection
1.1.3 Expressions
An expression is like a JavaScript code usually wrapped inside the double curly braces such as {{ expression }}
. Angular library evaluates the expression and produces a result.
Th Angular file upload API makes developer’s life easier, really! But it would be difficult for a beginner to understand this without an example. Therefore, let us create a simple example to show how to upload the file using the angular library.
2. AngularJS File Upload Example
Here is a step-by-step guide for implementing the file upload functionality in the angular web applications.
2.1 Tools Used
We are using Eclipse Kepler SR2, JDK 8 and Maven. Having said that, we have tested the code against JDK 1.7 and it works well.
2.2 Project Structure
Firstly, let’s review the final project structure if you are confused about where you should create the corresponding files or folder later!
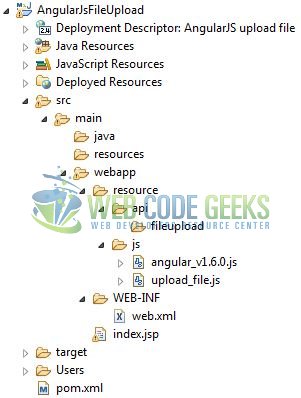
2.3 Project Creation
This section will show how to create a Java-based Maven project with Eclipse. In Eclipse Ide, go to File -> New -> Maven Project
.
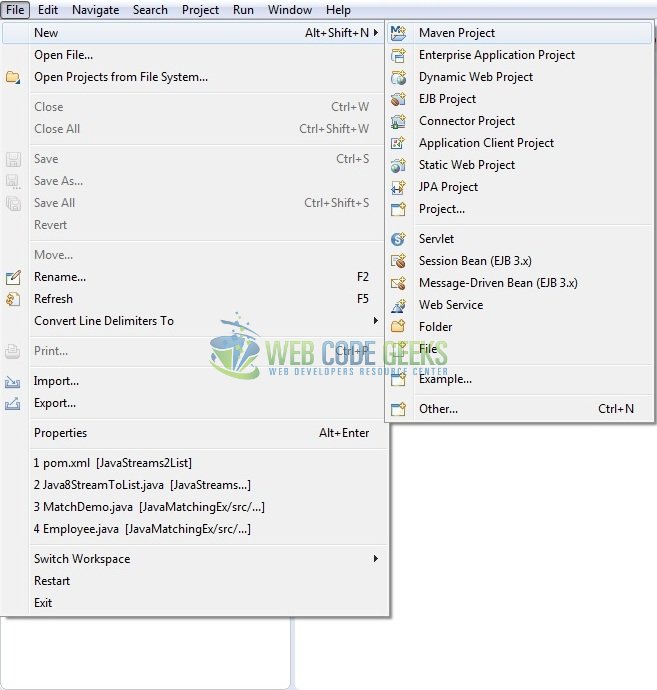
In the New Maven Project window, it will ask you to select project location. By default, ‘Use default workspace location’ will be selected. Just click on next button to proceed.
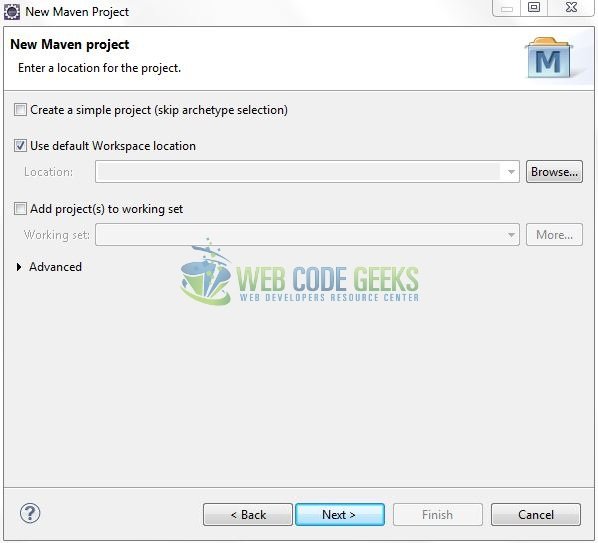
Select the ‘Maven Web App’ Archetype from the list of options and click next.
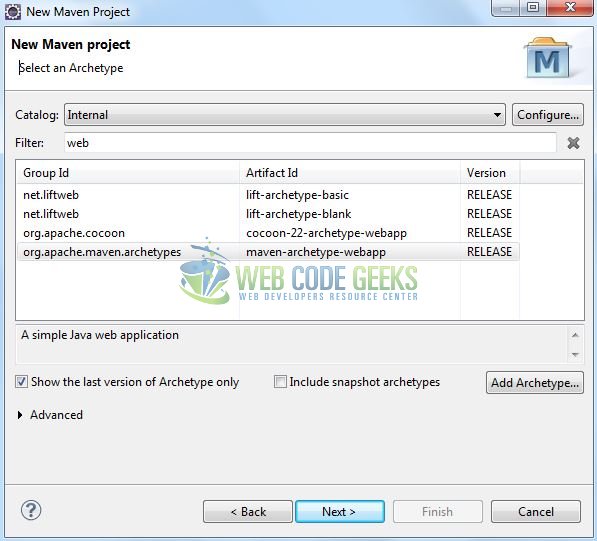
It will ask you to ‘Enter the group and the artifact id for the project’. We will enter the details as shown in the below image. The version number will be by default: 0.0.1-SNAPSHOT
.
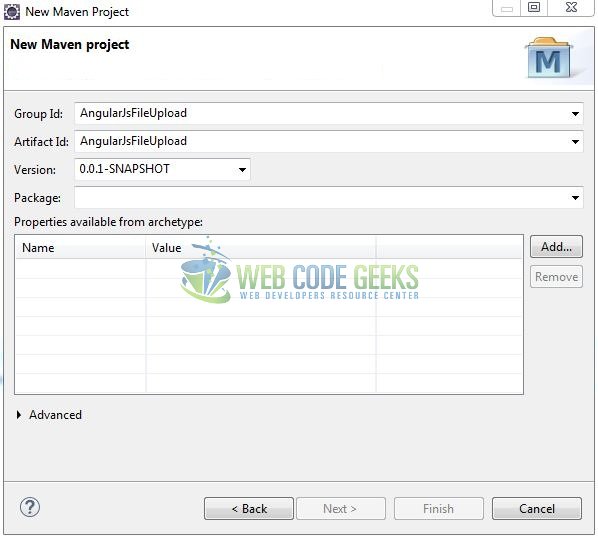
Click on Finish and the creation of a maven project is completed. If you see, it has downloaded the maven dependencies and a pom.xml
file will be created. It will have the following code:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>AngularJsFileUpload</groupId> <artifactId>AngularJsFileUpload</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> </project>
3. Application Building
Let’s start building an application to understand the basic building blocks of the file upload functionality in the angular library.
3.1 Load the Angular framework
Since it is a pure JavaScript framework, we should add its reference using the <script>
tag.
<script type="text/javascript" src="https://code.angularjs.org/1.2.2/angular.min.js"></script>
3.2 Define the Angular application
Next, we will define the angular application using the <ng-app>
and <ng-controller>
directives.
index.jsp
<div ng-app="app"> < form id="uploadfrom" ng-controller="ctrl"> ... </form> </div>
3.3 Create the Angular controller
The upload_file.js
JavaScript file is divided into two parts where the first part is the custom directive named fileInput
that displays the change occurred in the file input element. This change is captured using the link
option that takes a function i.e.
link:function(scope,elm,attrs){ …. }
The second part is the angular controller used by the application to get access to the files. Let’s understand these two parts with the help of the code snippet as shown below.
upload_file.js
angular.module('app', []). directive('fileInput', ['$parse', function($parse) { return { restrict: 'A', link: function(scope, elm, attrs) { elm.bind('change', function() { $parse(attrs.fileInput) .assign(scope, elm[0].files) scope.$apply() }); } } }]). controller('ctrl', ['$scope', '$http', function($scope, $http) { $scope.filesChanged = function(elm) { $scope.files = elm.files $scope.$apply(); } $scope.upload = function() { var fd = new FormData() angular.forEach($scope.files, function(file) { fd.append('file', file) }) $http.post('https://<your_sample_application_url>/resource/api/fileupload', fd, { transformRequest: angular.identity, headers: { 'Content-Type': undefined } }) .success(function(d) { $scope.message = "Upload Successful!"; $scope.successmessagebox = true; console.log(d); }) } } ])
3.4 Complete Application
Complete the above steps to show the front view and understand the file upload functionality in an angular web application.
index.jsp
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>AngularJS upload file</title> <!-- AngularJs Files --> <script type="text/javascript" src="https://code.angularjs.org/1.2.2/angular.min.js"></script> <script type="text/javascript" src="resource/js/upload_file.js"></script> <!-- Bootstrap File --> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-alpha.6/css/bootstrap.min.css"> </head> <body> <div id="angularUploadFile" class="container"> <h1 align="center" class="text-primary">AngularJS File Upload</h1> <hr /> <!------ ANGULAR JAVASCRIPT 'FILE UPLOAD' EXAMPLE ------> <div ng-app="app"> <form id="uploadfrom" ng-controller="ctrl"> <div class="form-group"> <input id="inp_file" type="file" file-input="files" multiple="multiple" /> </div> <!------ SUBMIT BUTTON ------> <div id="btn"> <button id="submit_btn" type="button" class="btn btn-primary" ng-click="upload()">Upload</button> </div> <!------ LIST OF UPLOADED FILES ------> <div> </div> <div id="uploaded-files-list"> <ul><li class="pointer" ng-repeat="file in files">{{ file.name }}</li></ul> </div> <!------ UPLOAD MESSAGE ------> <div> </div> <div id="result" class="alert alert-success ng-hide" ng-show="successmessagebox">{{ message }}</div> </form> </div> </div> </body> </html>
4. Run the Application
As we are ready for all the changes, let us compile the project and deploy the application on the tomcat server. To deploy the application on Tomat7, right-click on the project and navigate to Run as -> Run on Server
.
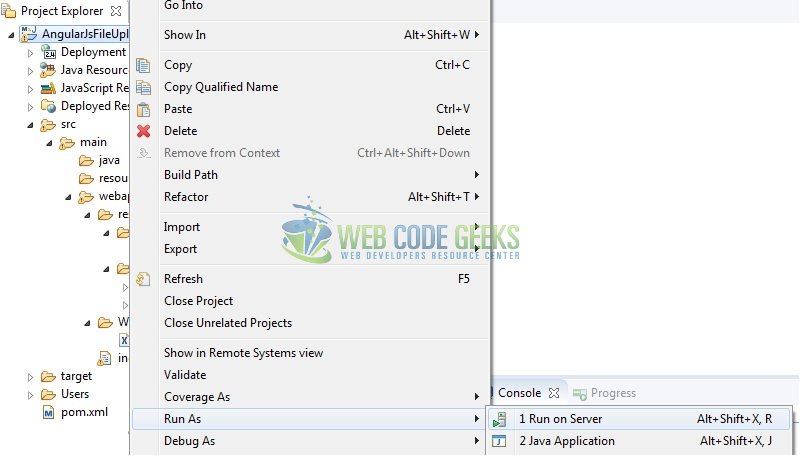
Tomcat will deploy the application in its web-apps folder and shall start its execution to deploy the project so that we can go ahead and test it in the browser.
5. Project Demo
Open your favorite browser and hit the following URL. The application’s index page will be displayed.
http://localhost:8080/AngularJsFileUpload/
Server name (localhost) and port (8080) may vary as per your Tomcat configuration.

In this example, users can select the file and upload it to the server by clicking the “Upload” button. If everything goes well, the $http
service will send the files to the controller class and will do the rest as shown in Fig. 8.
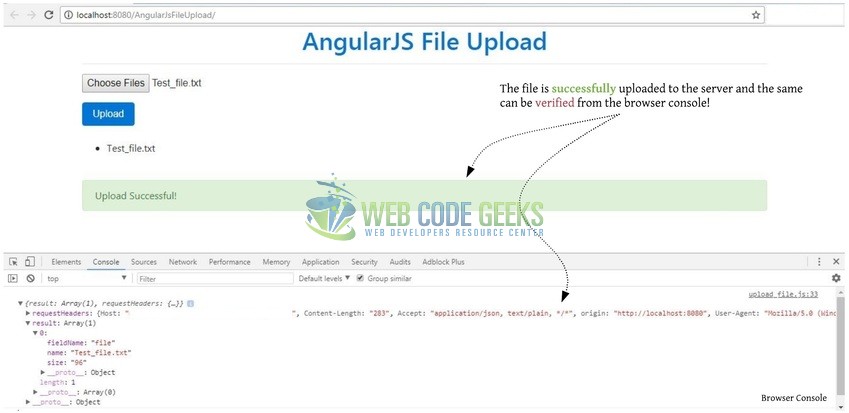
That’s all for this post. Happy Learning!!
6. Conclusion
In this section, developers learned how to upload a file in Angular web applications by using a custom directive that makes the value of the “file” field in the controller accessible. Then, using a controller we call an angular service to send it to the server. Developers can download the sample application as an Eclipse project in the Downloads section.
7. Download the Eclipse Project
This was a tutorial of the file upload feature in the angular library.
You can download the full source code of this example here: AngularJsFileUpload