jQuery Add/Remove Class Example
In this example, we’ll go through jQuery .addClass()
and .removeClass()
methods. jQuery provides a seamless, easy and efficent way to add or remove classes to specific DOM elements and trigger these events on the various listening events it provides like click
, mouseover
, mouseleave
ect.
These methods are very useful to dynamically change content based on your cases, and gives your website a rather interactive and engaging user experience. From changing styles and colors to animations, it provides powerful ways to get you going.
You’ll be able to access these methods just by including the jQuery library in your HTML document.
1. Basic Document Setup
To begin, create a new HTML document and add the following basic syntax inside:
<!DOCTYPE html> <html> <head> <title>jQuery Add/Remove Class Example</title> </head> <body> <!-- STYLE SECTION --> <style type="text/css"> </style> <!-- HTML SECTION --> <!-- JAVASCRIPT SECTION --> <script src="jquery-1.11.3.min.js"></script> <script type="text/javascript"> </script> </body> </html>
Also, let’s create some elements with classes and define properties for these classes in CSS. For now, there will be only one element and two classes:
<!-- HTML SECTION --> <div class="element">I am going to get classes added or removed!<div>
For now, we’d have this view:
Alongside with this element, let’s add two classes, one for styling and another for shaping:
<!-- STYLE SECTION --> <style type="text/css"> .style { font-family: "Clear Sans"; font-size: 1.5em; color: #AE0001; font-style: oblique; padding: 1em; } .shape { width: 25em; height: 3em; border: 0.1em solid #2c3e50; margin: 5em; line-height: 3em; text-align: center; } .decoration { text-decoration: underline; font-size: 1.2em; padding: 1em; width: 15em; } .color { color: #e74c3c; } .background { background-color: #ecf0f1; font-size: 1.2em; padding: 1em; width: 15em; } </style>
Next, we’ll continue adding and removing classes that we have.
2. .addClass() and .removeClass() Methods
2.1 Add Class
The addClass()
method adds the specified class(es) to each element in the set of matched elements:
1. .addClass("className")
, in which className
is a string containing one or more space-separated classes to be added to the class attribute of each matched element.
2. .addClass(function)
in which className
A function returning one or more space-separated class names to be added to the existing class name(s). Receives the index position of the element in the set and the existing class name(s) as arguments. Within the function, this refers to the current element in the set.
Now using jQuery, we can add a class to our .element
:
<!-- JAVASCRIPT SECTION --> <script type="text/javascript"> $('.element').addClass('style'); </script>
Easy as that, just selected the DOM element and added a class.
We added the ‘.style’ class, the same goes for ‘.shape’ class and we’d have this view:
But what is interesting, is that you can have more than one class added, just by leaving a blank space between them:
<!-- JAVASCRIPT SECTION --> <script type="text/javascript"> $('.element').addClass('style shape'); </script>
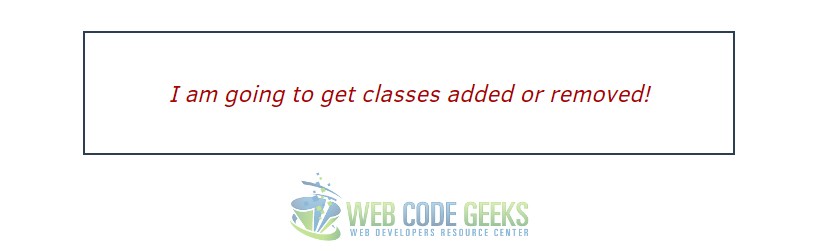
2.2 Remove Class
Similarily, .removeClass() removes a (or some) class/es from an element. To show this, add a new HTML element:
<!-- HTML SECTION --> <p class="decoration color background">I need to get rid of some classes</p>
In the JS section, let’s remove two of these classes:
<!-- JAVASCRIPT SECTION --> <script type="text/javascript"> $('.element').addClass('style shape'); </script>
The view we’d get is this:
3. Adding or Removing Classes on Event Listeners
You can trigger classes only when a specific event listener happens to become true. Let’s add some elements:
<!-- HTML SECTION --> <h2 class="decoration">This is a click event add class.</h2>
<!-- JAVASCRIPT SECTION --> <script type="text/javascript"> $('article').hide(); $('h2').click(function(e){ e.preventDefault(); $(this).addClass('shape color'); $(this).removeClass('decoration'); }); </script>
The result would be two classes added and one removed after click like so:
Toggle Classes on Event Listeners
You can use .toggleClass()
to toggle between the two states of an element, with and without classes like this:
<!-- JAVASCRIPT SECTION --> <script type="text/javascript"> $('article').hide(); $('h2').click(function(e){ e.preventDefault(); $(this).toggleClass('shape color'); }); </script>
The result would be:
4. Conclusion
To conclude, the jQuery methods for adding or removing classes are just right whenever it feels useful. You can actually add or remove content (by adding or removing classes), change the view on mouse or keyboard event ect. It is a process where you can try a lot and see how it best fits to what you want to achieve. I use it a lot when adding classes from a third party CSS stylesheet like an animation one, to animate elements mouseover
or mouseleave
. It is up to you.
5. Download
You can download the full source code of this example here: jQuery Add/Remove Class
Thanks for great post. I have the question about
$(this).toggleClass(‘shape color’);
In this example, we can add 2 class, can we?