jQuery Select Option Example
The aim of this example is to explain and use various jQuery selectors and methods related to manipulation the select dropdown element of HTML. The <select>
element is used to create a drop-down list. The <option>
tags inside the element define the available options in the list. These basic HTML elements are the ones we’ll apply special selector and/or methods to make them fit our every need.
So, why do we need to manipulate the select dropdown? Well, in many cases, the information stored in this element needs to be dynamic, meaning depended on some other action taken beforehand. Also, in other cases, the user may have interacted with the dropdown and we need to reset it when a new user accesses the page.
1. Document Setup
To begin, create a new HTML document and add the basic syntax inside, including the jQuery library link:
<!DOCTYPE html> <html> <head> <title>jQuery Select Option Example</title> </head> <body> <!-- HTML SECTION --> <!-- JAVASCRIPT SECTION --> <script src="jquery-1.11.3.min.js"></script> <script type="text/javascript"> </script> </body> </html>
Just to illustrate the idea of having a select dropdown in our page, this is an example:
<!-- HTML SECTION --> <select> <option>Option 1</option> <option>Option 2</option> <option>Option 3</option> </select>
Well, this is the most basic example, because normally the select
and option
also have their respective attributes applied to make them more dynamic and accessible. For now, it would have this simple view in the browser:

2. Cases and Examples
In the following section, we’re having a look at the various cases we can use jQuery with the select
element.
2.1 Get selected text from the select box
In this example, we’ll get the text of the selected option:
<select id="box"> <option>First Text</option> <option>Second Text</option> <option selected="selected">Third Text</option> </select> <p></p>
As you can see, we added a new id
and a selected
attribute and also an empty paragraph where we’ll store the text we got from the select box:
<script type="text/javascript"> var boxText = $("#box option:selected").text(); // get the text $('p').text(boxText); // place the boxText in a paragraph </script>
In this case the result would be “Third Text” because that is the option currently pre selected:

2.2 Select option using the value attribute
In this example we’ll get the text of a specific value on an option:
<select name="box"> <option value="1">First Text</option> <option value="2">Second Text</option> <option value="3">Third Text</option> </select>
In this case, we used the name
attribute inside the select element
. Additionally, option
s now have a unique value
identifier. In jQuery, we’ll be selecting using the name attribute and applying the .val()
method we’ll choose to display only the option we want as the default selected in the select box:
<script type="text/javascript"> $('[name=box]').val( 2 ); // this will select the second text </script>
As expected, the view in the browser would be:

2.3 Select option using the option text
In this third example, we’ll be selecting and displaying an option in a rather professional way.
<script type="text/javascript"> $('[name=box] option').filter(function() { // applied the filter method return ($(this).text() == 'Third Text'); // select 'Third Text' }).prop('selected', true); // change selected property to true </script>
So, after selecting the right select
element, we apply the filter
method (seems like we need to filter options) and give it a function as a parameter, which then leads to (returns) this element we’re selecting the text of which is equal to ‘Third Text’. But that just returns it, we don’t see anything. To display what is returned we add the .prop()
method to all of this and define the selected
attribute to true
. In this case, the third option will be selected like so:

Setting the selected attribute to true
is the same as setting it to 1
or selected
.
3. Best jQuery Select Plugins
There are quite a lot of jQuery plugins out there to help you with a nice a smoothly animated select box/dropdown that you may want to use. Below, we introduce some of the best plugins that you can use.
3.1 jQuery Nice Select
jQuery Nice Select is a lightweight jQuery plugin that replaces native select elements with customizable dropdowns.
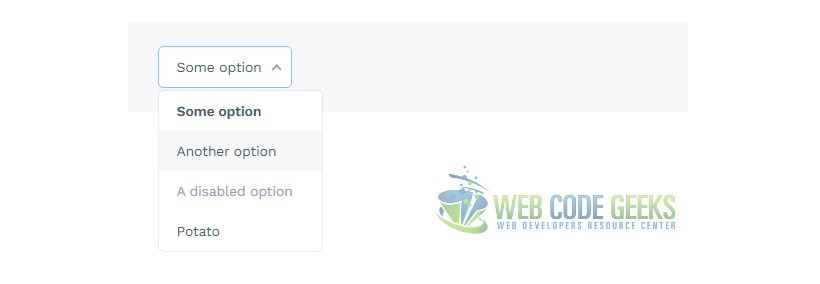
- You can find this plugin here: Source + Demo
3.2 jQuery Selectbox plugin
Custom select box replacement inspired by jQuery UI source. Requires jQuery 1.4.x or higher.
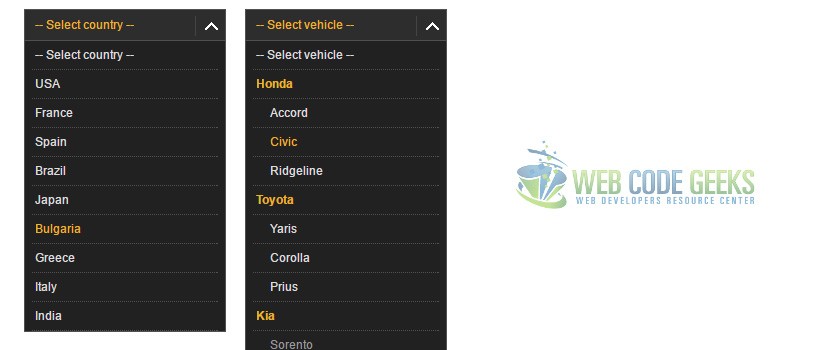
You can find this plugin here: Source + Demo
3.3 jQuery Selectbox plugin
Good dropdown plugin, adds images and description to otherwise boring drop downs.
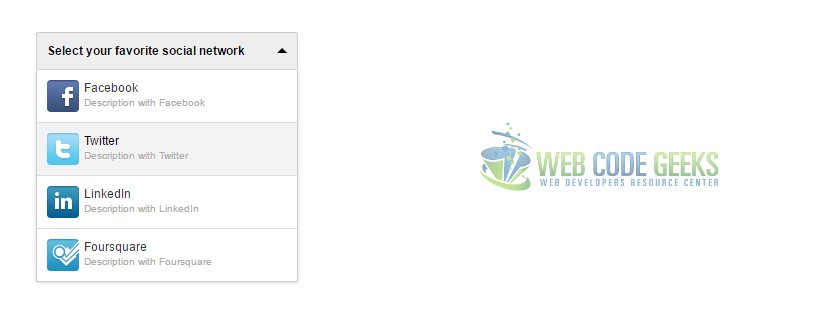
You can find this plugin here: Source + Demo
4. Conclusion
To conclude, there are many ways we can use and play with the select
dropdown element. Use jQuery to either manipulate behaviour or animate the dropdown box. While using jQuery to get or set values/text for the options is important based on what you want to achieve, animation will always be an enhancement towards a better user experience, so just try to make it look as good as possible.
5. Download the Source Code
This was an example about jQuery Select Option.