JQuery Datatable Example
Hello readers, in this article we will take a look on how to use a JQuery Data table plugin. We will learn how to implement a few simple examples using the JQuery Data table API and also we will check some details about this API. Before we start, check the requirements related to the technologies and the frameworks used to the example below:
- jquery 3.2.1
- jquery datatable plugin 1.10.15
- HTML
- CSS
1. Introduction
A DataTable is a JQuery´s plugin for creating HTML tables using the JQuery’s DOM manipulation power, this plugin allows add interactions to them. Interactions like searching, sorting, and pagination without configuration.
DataTable and its extensions provide an extensive API that allows manipulating the data contained in the table. The design of this API represents the structure of the data on the table and provide ways to interact with the table. The API have 6 key areas to work with a table and its data:
- Tables
- Columns
- Rows
- Cells
- Core
- Utilities
The DataTable API provides a set of extensions and plugins that add more functionality to the methods exposed through this API. To see the currently available extensions and plugins check the links below:
For the full API methods documentation please refer to API reference
2. Concepts
In order to be clear on some API concepts for the development of these examples, below I’ll explain a few important concepts to keep in mind for this implementation.
- Instance: Is a simple API object that refers to a specific set of DataTables.
- Result set: Is the data that is held for the API instance, comes in a javascript array way and you can access it using array notation.
[]
- Context: Describe the API DataTables linked with the instance, using this context you can access to one or more DataTables and interact with them.
3. Using The API
On this section, we’ll see the different ways that allow obtaining an API instance for one or more tables. The API provides 3 ways to access to it, these ways are:
$( selector ).DataTable();
$( selector ).dataTable().api();
new $.fn.dataTable.Api( selector );
The result of each way is a DataTables API instance that has the tables found by the selector. It is an important difference between $( selector ).DataTable();
and $( selector ).dataTable();
. The first one returns a DataTables instance, while the second one returns a jQuery type
object. An api()
method allows converting that JQuery object on a DataTables API instance.
The JQuery object created by the $( selector ).dataTable();
can be useful to manipulate the table node like any other JQuery object.
4. Chainig
This JQuery API feature allows the DataTables API to be used in an extensive way. This applies for methods that return the DataTable API instance, so you can call immediately another API method like is shown below.
var table = $('#wcg_example').DataTable(); table.search( 'Carlos' ).draw();
In this code example, we are using the search
and draw
API, on a single line. This can be helpful to make our code shorter and clear.
5. Setup Environment
For implementing this example we need the next tools:
- Web Browser (Chrome, FireFox, etc..), to check the Jquery browser compatibility please check this URL
- Text Editor (ATOM, Brackets, Notepad++, etc..)
In order to implement these examples, we’ll use the JQuery CDN. Also, you can download the JQuery library on a non-minified version for development purposes from here.
Also, we’ll use the DataTable CDN. In order to know how to use the CDN please refer to datatables.net. Also you can download the source code for development purposes with dependencies management tools like NPM and Bower.
6. Examples Implementation
In order to implement a JQuery data table it is first necessary to create a well formatted HTML table.
6.1 HTML Table
To use the JQuery data table we need to create an HTML table following the structure below:
<table id="wgc_table" class="display"> <thead> <tr> <th>Column 1</th> <th>Column 2</th> </tr> </thead> <tbody> <tr> <td>Row 1 Data 1</td> <td>Row 1 Data 2</td> </tr> <tr> <td>Row 2 Data 1</td> <td>Row 2 Data 2</td> </tr> </tbody> </table>
The previous table must have the tags thead
and tbody.
Also like optional you can use the tfoot
tag. So this is the stantard html table structure that allows to integrate the JQuery data table with our html table.
6.2 JQuery DataTable Basic Initialization Example
In this example, we’ll see how to create a basic JQuery data table using the DataTable API and an HTML table. Please check the code below to see the implementation.
basic_datatable_initialization_example.html
<!DOCTYPE html> <html> <head> <!-- Using the jquery CDN --> <script src="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9hamF4Lmdvb2dsZWFwaXMuY29tL2FqYXgvlibs/jquery/3.2.1/jquery.min.js"></script> <!-- Using the jquery dataTables CSS CDN --> <link rel="stylesheet" type="text/css" href="//cdn.datatables.net/1.10.15/css/jquery.dataTables.css"> <!-- Using the jquery dataTables API CDN --> <script type="text/javascript" charset="utf8" src="//cdn.datatables.net/1.10.15/js/jquery.dataTables.js"></script> </head> <body> <!-- Target table to instantiate the DataTable API --> <table id="wgc_table" class="display"> <thead> <tr> <th>Column 1</th> <th>Column 2</th> </tr> </thead> <tbody> <tr> <td>Row 1 Data 1</td> <td>Row 1 Data 2</td> </tr> <tr> <td>Row 2 Data 1</td> <td>Row 2 Data 2</td> </tr> </tbody> </table> <!-- JQuery code to initializate the DataTables API --> <script> $(document).ready( function () { $('#wgc_table').DataTable();//Basic DataTable API instance }); </script> </body> </html>
The output of the previous example shows us a default JQuery data table with some other default components provided by the API like a paginator, search text box, sorting component, and a default CSS style. See the output below.
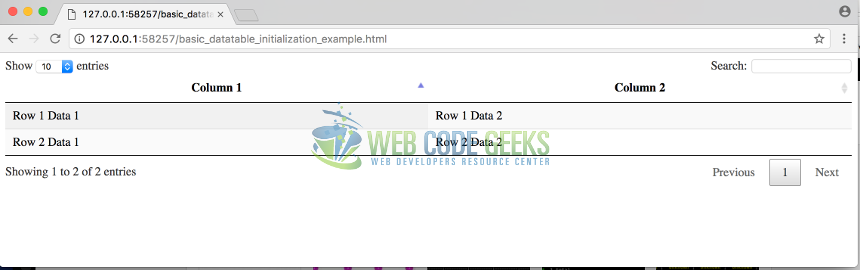
6.3 JQuery DataTable Advanced Initialization Example
In this example, we’ll see how to create a JQuery data table using an advanced DataTable API initialization. In this case data table events like order, search and page. For these events we will add a notification function that will be fired when the data table event occurs. Please check the code below to see the implementation.
advanced_datatable_initialization_example.html
<!DOCTYPE html> <html> <head> <!-- Using the jquery CDN --> <script src="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9hamF4Lmdvb2dsZWFwaXMuY29tL2FqYXgvlibs/jquery/3.2.1/jquery.min.js"></script> <!-- Using the jquery dataTables CSS CDN --> <link rel="stylesheet" type="text/css" href="//cdn.datatables.net/1.10.15/css/jquery.dataTables.css"> <!-- Using the jquery dataTables API CDN --> <script type="text/javascript" charset="utf8" src="//cdn.datatables.net/1.10.15/js/jquery.dataTables.js"></script> </head> <body> <!-- Target table to instantiate the DataTable API --> <table id="wgc_table" class="display"> <thead> <tr> <th>Column 1</th> <th>Column 2</th> </tr> </thead> <tbody> <tr> <td>Row 1 Data 1</td> <td>Row 1 Data 2</td> </tr> <tr> <td>Row 2 Data 1</td> <td>Row 2 Data 2</td> </tr> <tr> <td>Row 3 Data 1</td> <td>Row 3 Data 2</td> </tr> <tr> <td>Row 4 Data 1</td> <td>Row 4 Data 2</td> </tr> <tr> <td>Row 5 Data 1</td> <td>Row 5 Data 2</td> </tr> <tr> <td>Row 6 Data 1</td> <td>Row 6 Data 2</td> </tr> <tr> <td>Row 7 Data 1</td> <td>Row 7 Data 2</td> </tr> <tr> <td>Row 8 Data 1</td> <td>Row 8 Data 2</td> </tr> <tr> <td>Row 9 Data 1</td> <td>Row 9 Data 2</td> </tr> <tr> <td>Row 10 Data 1</td> <td>Row 10 Data 2</td> </tr> <tr> <td>Row 11 Data 1</td> <td>Row 11 Data 2</td> </tr> <tr> <td>Row 12 Data 1</td> <td>Row 12 Data 2</td> </tr> <tr> <td>Row 13 Data 1</td> <td>Row 13 Data 2</td> </tr> <tr> <td>Row 14 Data 1</td> <td>Row 14 Data 2</td> </tr> <tr> <td>Row 15 Data 1</td> <td>Row 15 Data 2</td> </tr> <tr> <td>Row 16 Data 1</td> <td>Row 16 Data 2</td> </tr> </tbody> </table> <!-- Div panel for data table events --> <div id="events_output"> </div> <!-- JQuery code to initializate the DataTables API --> <script> //when the DOM is rendered $(document).ready(function() { /** This function will be fire when one of these events occurs Order, Search and page. This function prints a div with the name and date of the event fired. */ var whenTheEventIsFired = function (eventName){ var output = $('#events_output')[0]; output.innerHTML += '<div>'+eventName+' event - '+new Date().getTime()+'</div>'; } /** Advanced initialization adding a function that will be executed when one of the data table events provided by the API occurs. */ $('#wgc_table') .on( 'order.dt', function () { whenTheEventIsFired( 'Order' ); } )//when the table is ordered .on( 'search.dt', function () { whenTheEventIsFired( 'Search' ); } )//when search on the table using the search text box .on( 'page.dt', function () { whenTheEventIsFired( 'Page' ); } )//when the pagination buttons are clicked. .DataTable(); } ); </script> </body> </html>
After fired the 3 events provided by the JQuery data table API (order, search, and page) the function whenTheEventIsFired
is executed and creates a new div container with the event name and the date when was executed. See the output below.
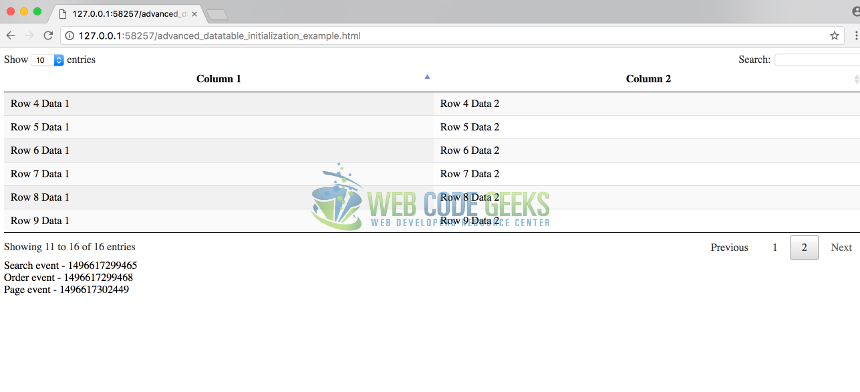
6.3 JQuery DataTable Initialization Using The API And Javascript Data Example
In this example, we’ll see how to create a JQuery data table using directly the DataTable API and use a Javascript array in order to initialize the table with a dynamic data. We will also see how to create on the fly a JQuery data table using just the HTML table tag. Please check the code below to see the implementation.
api_javascript_datatable_initialization.html
<!DOCTYPE html> <html> <head> <!-- Using the jquery CDN --> <script src="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9hamF4Lmdvb2dsZWFwaXMuY29tL2FqYXgvlibs/jquery/3.2.1/jquery.min.js"></script> <!-- Using the jquery dataTables CSS CDN --> <link rel="stylesheet" type="text/css" href="//cdn.datatables.net/1.10.15/css/jquery.dataTables.css"> <!-- Using the jquery dataTables API CDN --> <script type="text/javascript" charset="utf8" src="//cdn.datatables.net/1.10.15/js/jquery.dataTables.js"></script> <!-- Loading the JS script that allow us create the JQuery data table --> <script type="text/javascript" charset="utf8" src="api_javascript_datatable_initialization.js"></script> </head> <body> <!-- Target table to instantiate the DataTable API --> <table id="wgc_table" class="display"></table> </body> </html>
api_javascript_datatable_initialization.js
/** The data that will be show by the data table */ var dataSet = [["Carlos", "Nixon", "35"], ["Garrett", "Winters", "33"], ["Ashton", "Cox", "31"], ["Cedric", "Kelly", "38"], ["Airi", "Satou", "34"], ["Brielle", "Williamson", "32"], ["Herrod", "Chandler", "25"], ["Rhona", "Davidson", "22"], ["Colleen", "Hurst", "28"], ["Sonya", "Frost", "30"], ["Jena", "Gaines", "27"], ["Quinn", "Flynn", "20"], ["Charde", "Marshall", "39"], ["Haley", "Kennedy", "40"], ["Tatyana", "Fitzpatrick", "23"], ["Pedro", "Perez", "21"]]; /** When the DOM is ready, we use the data table API to create a new one based on a javascript array that provides the data. */ $(document).ready(function() { $('#wgc_table').DataTable( { data: dataSet, //data used by the data table columns: [ { title: "First Name" },//defining table presentation using the API { title: "Last Name" }, { title: "Age" } ] }); });
The output of the previous code will be a fully functional JQuery data table. Note that in the HTML page it was necessary just to create the table HTML tag with an ID to allows to JQuery to use that tag to create the data table using the API and define some presentation aspects like the column’s title. See the output below.
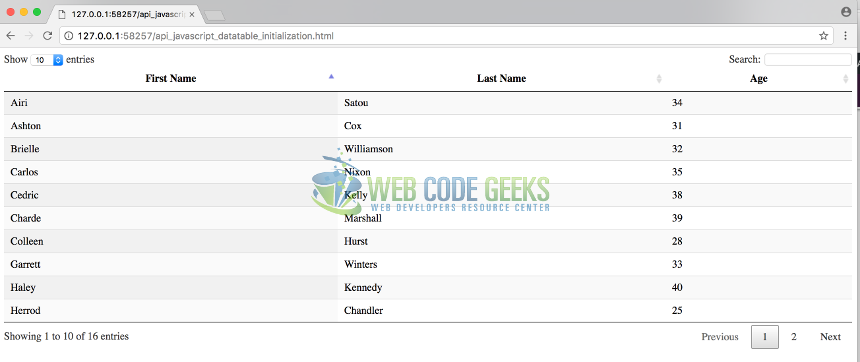
The official JQuery data table site provides a lot of examples that allow you to get the necessary knowledge and understanding to use the API and use a different kind of functionalities that this library provides.
To see all the usage examples please refer to the official examples site here.
7. Conclusion
In this post we learned how to use the JQuery data table library, some specific API aspects, and we saw some simplest examples to get more understanding about this library and its usage.
Also, we saw the functionalities that are provided by default for this API like sort pagination and searching. This is a powerful library based on JQuery framework that allows the developers to create a fully functional data table based on a different kind of data resources like javascript data JSON or Ajax API calls with a few lines of code.
To explore in more detail the API of this library, please refer to the official API reference here.
8. Download The Source Code
These were a JQuery data table examples.
You can download the full source code of this example here: JQueryDataTableExample