jQuery AJAX Example
The aim of this example is to give you a full understanding of AJAX, which stands for Asynchronous Javascript and XML. Ajax is not a programming language or a tool, but a concept.
Ajax is a client-side script that communicates to and from a server/database without the need for a postback or a complete page refresh.
The best definition for Ajax would be “the method of exchanging data with a server, and updating parts of a web page – without reloading the entire page.”
Ajax itself is mostly a generic term for various JavaScript techniques used to connect to a web server dynamically without necessarily loading multiple pages. In a more narrowly-defined sense, it refers to the use of XmlHttpRequest objects to interact with a web server dynamically via JS.
1. An Introduction to AJAX!
1.1 How we came here?
Traditionally webpages required reloading to update their content. For web-based email this meant that users had to manually reload their inbox to check and see if they had new mail. This had huge drawbacks: it was slow and it required user input. When the user reloaded their inbox, the server had to reconstruct the entire web page and resend all of the HTML, CSS, JavaScript, as well as the user’s email.
This was hugely inefficient. Ideally, the server should only have to send the user’s new messages, not the entire page. By 2003, all the major browsers solved this issue by adopting the XMLHttpRequest (XHR) object, allowing browsers to communicate with the server without requiring a page reload.
The XMLHttpRequest object is part of a technology called Ajax (Asynchronous JavaScript and XML). Using Ajax, data could then be passed between the browser and the server, using the XMLHttpRequest API, without having to reload the web page. With the widespread adoption of the XMLHttpRequest object it quickly became possible to build web applications like Google Maps, and Gmail that used XMLHttpRequest to get new map tiles, or new email without having to reload the entire page.
Ajax requests are triggered by JavaScript code; your code sends a request to a URL, and when it receives a response, a callback function can be triggered to handle the response. Because the request is asynchronous, the rest of your code continues to execute while the request is being processed, so it’s imperative that a callback be used to handle the response.
Unfortunately, different browsers implement the Ajax API differently. Typically this meant that developers would have to account for all the different browsers to ensure that Ajax would work universally. Fortunately, jQuery provides Ajax support that abstracts away painful browser differences. It offers both a full-featured $.ajax()
method, and simple convenience methods such as $.get()
, $.getScript()
, $.getJSON()
, $.post()
, and $().load()
.
1.2 Getting to Know AJAX Better
Like DHTML and LAMP, AJAX is not a technology in itself, but a group of technologies. AJAX uses a combination of:
• HTML and CSS for marking up and styling information.
• The DOM accessed with JavaScript to dynamically display and interact with the information presented.
• A method for exchanging data asynchronously between browser and server, thereby avoiding page reloads. The XMLHttpRequest (XHR) object is usually used, but sometimes an IFrame object or a dynamically added tag is used instead.
• A format for the data sent to the browser. Common formats include XML, pre-formatted HTML, plain text, and JavaScript Object Notation (JSON). This data could be created dynamically by some form of server-side scripting.
A picture being worth a thousand words, below a diagram that illustrates the communication between the client and the remote server, as well as the differences between the classic and the AJAX-powered applications:
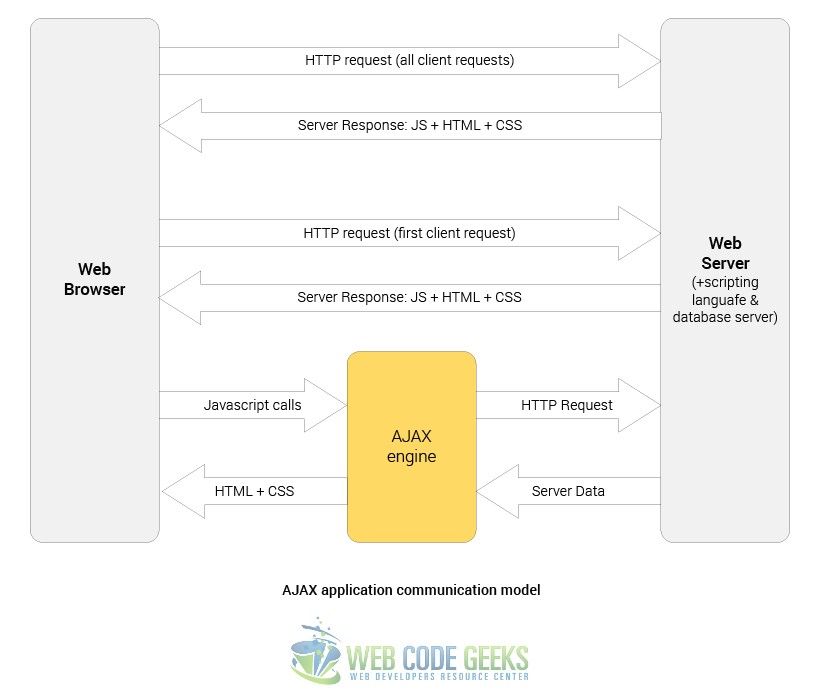
For the orange part, you can do everything by hand (with the XMLHttpRequest
object) or you can use famous JavaScript libraries like jQuery, Prototype, YUI, etc to “AJAXify” the client-side of your application. Such libraries aim to hide the complexity of JavaScript development (e.g. the cross-browser compatibility), but might be overkill for a simple feature.
On the server-side, some frameworks can help too (e.g. DWR or RAJAX if you are using Java), but all you need to do is basically to expose a service that returns only the required informations to partially update the page (initially as XML/XHTML – the X in AJAX – but JSON is often preferred nowadays).
1.3 AJAX Benefits
There are 4 main benefits of using Ajax in web applications:
1. Callbacks: Ajax is used to perform a callback, making a quick round trip to and from the server to retrieve and/or save data without posting the entire page back to the server. By not performing a full postback and sending all form data to the server, network utilization is minimized and quicker operations occur. In sites and locations with restricted bandwidth, this can greatly improve network performance. Most of the time, the data being sent to and from the server is minimal. By using callbacks, the server is not required to process all form elements. By sending only the necessary data, there is limited processing on the server. There is no need to process all form elements, process the ViewState, send images back to the client, or send a full page back to the client.
2. Making Asynchronous Calls: Ajax allows you to make asynchronous calls to a web server. This allows the client browser to avoid waiting for all data to arrive before allowing the user to act once more.
3. User-Friendly: Because a page postback is being eliminated, Ajax enabled applications will always be more responsive, faster and more user-friendly.
4. Increased Speed: The main purpose of Ajax is to improve the speed, performance and usability of a web application. A great example of Ajax is the movie rating feature on Netflix. The user rates a movie and their personal rating for that movie will be saved to their database without waiting for the page to refresh or reload. These movie ratings are being saved to their database without posting the entire page back to the server.
2. Implementation
To begin, set up your document, and get to learn how to organize your files for this to work.
2.1 Basic Document Setup
To begin, create a new HTML document and add the basic syntax inside it like so:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>jQuery AJAX Example</title> </head> <body> <!-- HTML SECTION --> <!-- JAVASCRIPT SECTION --> <script src="jquery-1.11.3.min.js"></script> <script type="text/javascript"> </script> </body> </html>
We will create another HTML file, where we’ll add some random content that we’d like to retrieve later with AJAX:
<!DOCTYPE html> <html> <head> <title>jQuery AJAX Example</title> </head> <body> <!-- HTML SECTION --> <div class="content"> <h2>I just showed up here!</h2> <p>I am a paragraph, just retrieved with AJAX!</p> <img src="image.png"> </div> </body> </html>
Here, we don’t need the javascript section at all. This is how this page looks like for now:
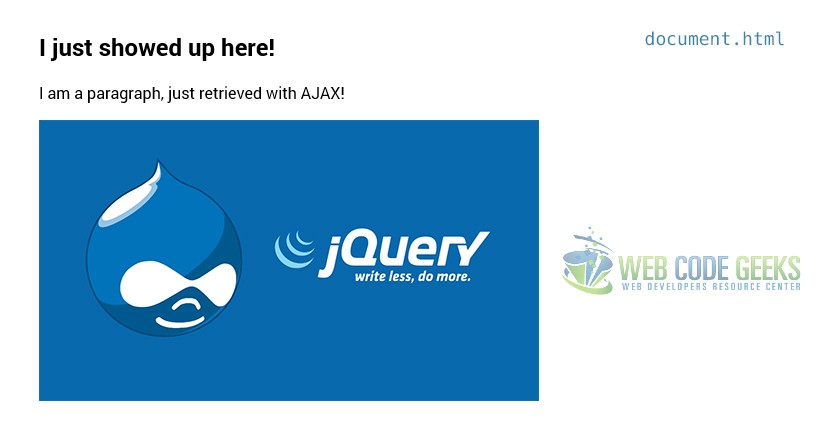
2.2 Ajax Declaration
The basix syntax in which you can start using AJAX is:
jQuery.ajax('url', {settings})
or simply $.ajax('url', {settings})
where:
1. url is of a type string
and contains the url to where the request is sent.
2. settings is of a type PlainObject
and can be considered set of key/value pairs that configure the Ajax request.
This is how a simple AJAX request would look like:
$.ajax('file.html', { success: function(){ /* do sth if the file.html is reached successfully */ }, type: 'GET' });
As you can see, in this simple example, we only configured two settings, because settings are optional, and you can set as many as you like referring to the existing ones. The success
function is going to do sth if the request is accepted and data can be retrieved, while type
is telling AJAX that this is a request and not a submission on the server. We’ll have a more extensive look at settings later in this article.
You can achieve the same using a shorthand method which is $.get(url, success);
like this:
$.get('document.html', function(response){ $('.content').html(response).slideDown(); });
2.3 A Real-World AJAX Example!
We’ve already set up our basic HTML docs, now let’s add some content on the first one:
<!-- HTML SECTION --> <div class="wrapper"> <button>Click Me</button> <!-- content will be shown on this button click --> <div class="content"></div> <!-- content will be shown in this div --> </div>
Now, on the JavaScript section, we’re going to listen for a click on the button we just created and then show any element we want that is located on the remote document.html
file: (look at the image below the source code for explanation)
<!-- JAVASCRIPT SECTION --> <script src="jquery-1.11.3.min.js"></script> <script type="text/javascript"> $('button').on('click', function(){ $.ajax('document.html', { success: function(response){ $('.content').html(response); }, type: 'GET', }); }); </script>

This would mean that AJAX will call all elements found in document.html
and show them just at the moment of clicking the button like so:
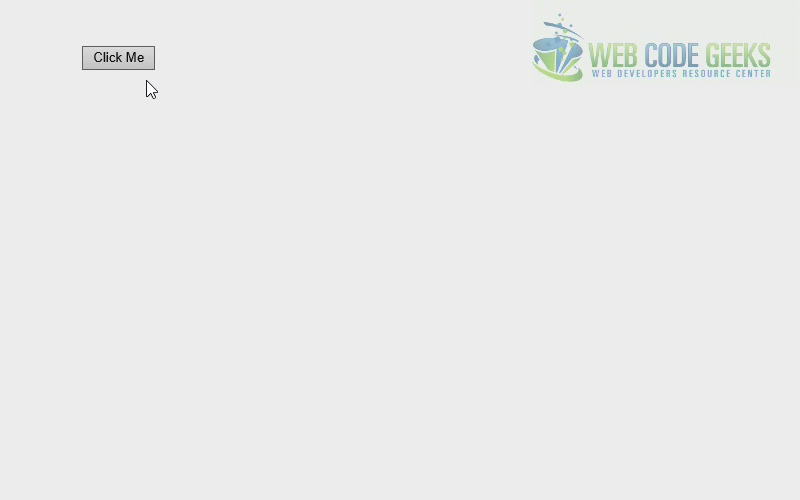
Additionally, you can show only part of a HTML document (that is, you can filter to show only section of a website). Let’s take the example above, where we have three different tag types that contain their own data, we ahve h2
, a paragraph p
and an image img
. If you want to show only one of them, or two you can modify your ajax call to search the DOM for the response and there find the wanted tag (or class if we referred to classes):
<!-- JAVASCRIPT SECTION --> <script src="jquery-1.11.3.min.js"></script> <script type="text/javascript"> $('button').on('click', function(){ $.ajax('document.html', { success: function(response){ $('.content').html($(response).find('img, h2').fadeIn()); }, type: 'GET', }); }); </script>
Only the line where we put data under the .content
div is changed to get both the image and h2 element on button click and fade them in, but not the paragraph. Now see it in action:
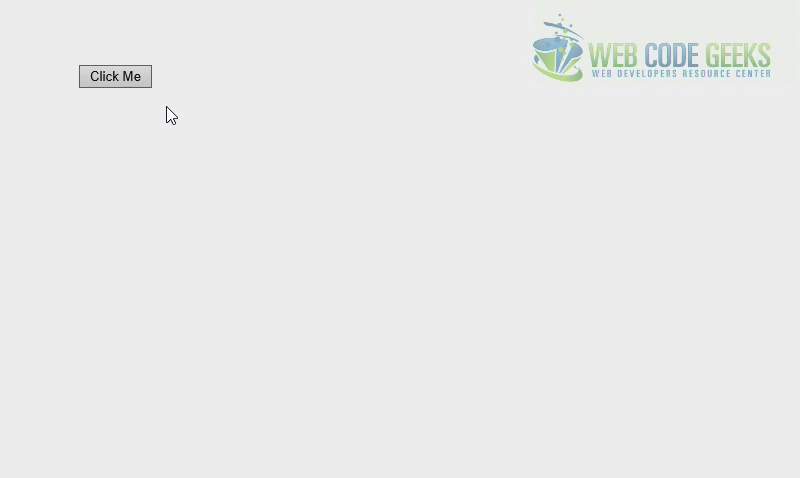
3. AJAX Settings
Below, there is basic information about the most important of the ajax settings that you can use.
3.1 accepts
• default: depends on DataType
• type: PlainObject
• function: The content type sent in the request header that tells the server what kind of response it will accept in return.
3.2 async
• default: true
• type: Boolean
• function: By default, all requests are sent asynchronously (i.e. this is set to true
by default). If you need synchronous requests, set this option to false
. Cross-domain requests and dataType: "jsonp"
requests do not support synchronous operation. Note that synchronous requests may temporarily lock the browser, disabling any actions while the request is active.
3.3 beforeSend
• default: does not apply
• type: Function
• function: A pre-request callback function that can be used to modify the jqXHR (in jQuery 1.4.x, XMLHTTPRequest) object before it is sent. Use this to set custom headers, etc. The jqXHR and settings objects are passed as arguments. This is an Ajax Event. Returning false
in the beforeSend
function will cancel the request.
3.4 cache
• default: true, false for dataType 'script' and 'jsonp'
• type: Boolean
• function: If set to false
, it will force requested pages not to be cached by the browser. Note: Setting cache
to false will only work correctly with HEAD and GET requests. It works by appending “_={timestamp}” to the GET parameters. The parameter is not needed for other types of requests, except in IE8 when a POST is made to a URL that has already been requested by a GET.
3.5 complete
• default: does not apply
• type: Function
• function: A function to be called when the request finishes (after success
and error
callbacks are executed). The function gets passed two arguments: The jqXHR (in jQuery 1.4.x, XMLHTTPRequest) object and a string categorizing the status of the request (“success
“, “notmodified
“, “nocontent
“, “error
“, “timeout
“, “abort
“, or “parsererror
“).
3.6 contents
• default: does not apply
• type: PlainObject
• function: An object of string/regular-expression pairs that determine how jQuery will parse the response, given its content type.
3.7 contentType
• default: 'application/x-www-form-urlencoded; charset=UTF-8'
• type: Boolean
, String
• function: When sending data to the server, use this content type. Default is “application/x-www-form-urlencoded; charset=UTF-8”, which is fine for most cases. If you explicitly pass in a content-type to $.ajax(), then it is always sent to the server (even if no data is sent).
3.8 context
• default: does not apply
• type: PlainObject
• function: This object will be the context of all Ajax-related callbacks. By default, the context is an object that represents the Ajax settings used in the call ($.ajaxSettings
merged with the settings passed to $.ajax
). For example, specifying a DOM element as the context will make that the context for the complete
callback of a request, like so:
$.ajax({ url: "test.html", context: document.body }).done(function() { $( this ).addClass( "done" ); });
3.9 data
• default: does not apply
• type: PlainObject
, String
, Array
• function: Data to be sent to the server. It is converted to a query string, if not already a string. It’s appended to the url for GET-requests. See processData
option to prevent this automatic processing. Object must be Key/Value pairs. If value is an Array, jQuery serializes multiple values with same key based on the value of the traditional setting (described below).
3.10 data
• default: does not apply
• type: Function
• function: A function to be used to handle the raw response data of XMLHttpRequest. This is a pre-filtering function to sanitize the response. You should return the sanitized data. The function accepts two arguments: The raw data returned from the server and the ‘dataType’ parameter.
3.11 dataType
• default: (xml
, json
, script
, or html
)
• type: String
• function: The type of data that you’re expecting back from the server. If none is specified, jQuery will try to infer it based on the MIME type of the response (an XML MIME type will yield XML, in 1.4 JSON will yield a JavaScript object, in 1.4 script will execute the script, and anything else will be returned as a string). The available types (and the result passed as the first argument to your success callback) are:
– "xml"
: Returns a XML document that can be processed via jQuery.
– "html"
: Returns HTML as plain text; included script tags are evaluated when inserted in the DOM.
– "script"
: Evaluates the response as JavaScript and returns it as plain text. Disables caching by appending a query string parameter, _=[TIMESTAMP]
, to the URL unless the cache
option is set to true
. Note: This will turn POSTs into GETs for remote-domain requests.
– "json"
: Evaluates the response as JSON and returns a JavaScript object. Cross-domain
– "jsonp"
: Loads in a JSON block using JSONP. Adds an extra "?callback=?"
to the end of your URL to specify the callback. Disables caching by appending a query string parameter, "_=[TIMESTAMP]"
, to the URL unless the cache
option is set to true
.
– "text"
: A plain text string.
3.12 error
• default: does not apply
• type: Function
• function: A function to be called if the request fails. The function receives three arguments: The jqXHR (in jQuery 1.4.x, XMLHttpRequest) object, a string describing the type of error that occurred and an optional exception object, if one occurred. Possible values for the second argument (besides null
) are “timeout
“, “error
“, “abort
“, and “parsererror
“. When an HTTP error occurs, errorThrown
receives the textual portion of the HTTP status, such as “Not Found” or “Internal Server Error.”
3.13 global
• default: true
• type: Boolean
• function: Whether to trigger global Ajax event handlers for this request. The default is true
. Set to false
to prevent the global handlers like ajaxStart
or ajaxStop
from being triggered. This can be used to control various Ajax Events.
3.14 method
• default: 'GET'
• type: String
• function: The HTTP method to use for the request (e.g. “POST
“, “GET
“, “PUT
“).
3.15 success
• default: does not apply
• type: Function
• function: A function to be called if the request succeeds. The function gets passed three arguments: The data returned from the server, formatted according to the dataType
parameter or the dataFilter
callback function, if specified; a string describing the status; and the jqXHR
(in jQuery 1.4.x, XMLHttpRequest) object.
3.16 timeout
• default: does not apply
• type: Number
• function: Set a timeout (in milliseconds) for the request. This will override any global timeout set with $.ajaxSetup()
. The timeout period starts at the point the $.ajax
call is made; if several other requests are in progress and the browser has no connections available, it is possible for a request to time out before it can be sent.
3.17 type
• default: 'GET'
• type: String
• function: An alias for method
. You should use type if you’re using versions of jQuery prior to 1.9.0.
3.18 url
• default: 'current page'
• type: String
• function: A string containing the URL to which the request is sent.
3.19 username
• default: does not apply
• type: String
• function: A username to be used with XMLHttpRequest in response to an HTTP access authentication request.
3.19 password
• default: does not apply
• type: String
• function: A password to be used with XMLHttpRequest in response to an HTTP access authentication request.
To be as precise as possible, information on AJAX options/settings is taken from the official website, where you can find even more by clicking here.
4. Where to use AJAX?
Ajax should be used anywhere in a web application where small amounts of information could be saved or retrieved from the server without posting back the entire pages. A good example of this is data validation on save actions. Another example would be to change the values in a drop down list-box based on other inputs, such as state and college list boxes. When the user selects a state, the college list box will repopulate with only colleges and universities in that state.
Another great example is when the client needs to save or retrieve session values from the server, based on a user preference such as the height, width or position of an object. Adjusting the width could make a callback to the server to set the session variable for the new width. This way, whenever the page is refreshed, the server can adjust the object’s width based on this session variable. Otherwise, the object would go back to its initial default width.
Other features include text hints and autocomplete text boxes. The client types in a couple of letters and a list of all values that start with those letters appear below. A callback is made to a web service that will retrieve all values that begin with these characters. This is a fantastic feature that would be impossible without Ajax and is also part of the Ajax Control Toolkit.
Credits go to SegueTech for providing a great overview on AJAX use cases.
5. Conclusion
There are so much ways you can benefit from using AJAX that you barely have time to notice all. However, it is important to learn the basics and expand knowledge on the various options that we presented above. This way you’ll know exactly when to use certain options to achieve data retrieval, on several conditions! AJAX can be as well used with php or other back-end programming languages, but here we focused on jQuery, where it gets its’ most usable features and it is easier to implement.
6. Download
You can download the full source code of this example here: jQuery AJAX
Note: You can only try and see the results of this code in Internet Explorer because we used offline files to demonstrate AJAX, which other browser cannot handle or require extra set up.
Interesting solution. Why did you choose to use the AJAX method over the Get method and the Find over Filter? Consider that you have a page that you want to dynamically change using Ajax based on conditions that you anticipate and that you have a file with the relevant tags for these conditions. In my world, I would save these conditions as HTML classes in the file; although ID’s would work also. With the Find method, would I not have to know the names of the tags I want to return, whereas with the filter method I filter on the… Read more »