jQuery Data Grid Control Example
In this example we will learn how to integrate a data grid plugin into a web application to display and manage many records of data instead of using the simple table
element without manipulation.
Most of these plugins provide a very flexible API to easily add/edit/remove records, paginate, sort and filter data based on predefined criteria.
I have chosen for our example the popular jQuery DataTables plugin because it is a highly flexible tool arround the web, provide a beautiful, expressive API and it is free open source software.
Default Datatable Example
Before we start coding, we should download the plugin from the official website here, I will use for this example the latest version 1.10.5
.
Now, create an HTML file called index.html then add link to jQuery library and datatable JavaScript and CSS files between the head
tags.
<link rel="stylesheet" type="text/css" href="css/jquery.dataTables.css"> <script type="text/javascript" language="javascript" src="js/jquery.js"></script> <script type="text/javascript" language="javascript" src="js/jquery.dataTables.js"></script>
Into the body tags, add a table
element with the attribute id="MyDT"
.
<table id="MyDT" cellspacing="0" width="100%">
cellspacing="0"
mean reduce the space between cells to zero.
Now between the table
tags, add the tags thead
, tbody
and tfoot
like below.
<table id="MyDT" cellspacing="0" width="100%"> <thead> ... </thead> <tbody> ... </tbody> <tfoot> ... </tfoot> </table>
The thead
and tfoot
tags are responsible for displaying the columns titles at the header and the footer of table. Note that the number of cells must be identical in each section.
After adding some records, the result of our tables is:
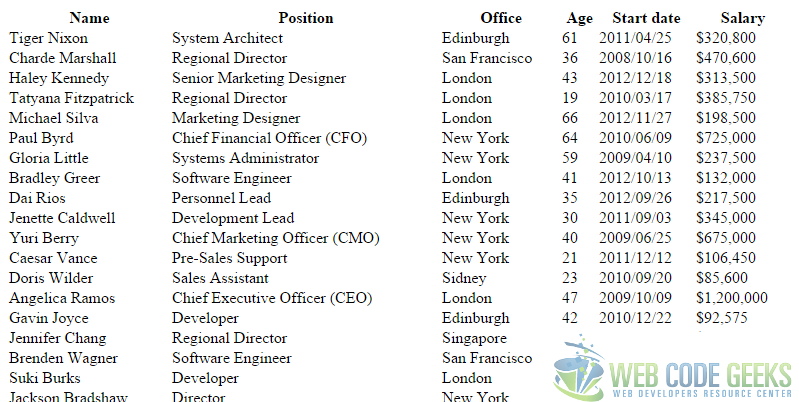
But wait! where is it our grid, the pagination?
You’re right, we should call the plugin, so put between a script
tags in the head
section the following:
$(document).ready(function() { $('#MyDT').DataTable(); });
DataTables has most features enabled by default, so all you need to do to use it with your own tables is to call the construction function.
Searching, ordering, paging etc goodness will be immediately added to the table, as shown in this example.
Voila:
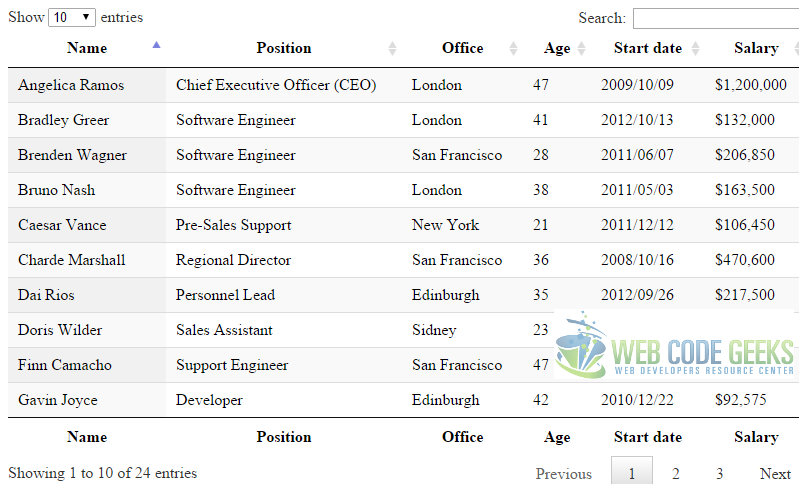
So, what’s happen when we apply the magic DataTable()
function:
- The table toke default cool style from the plugin.
- The rows are distributed to many pages and a paging navigation appear at the bottom right of the table.
- At the top left and bottom left, we have information about entries total records.
- And at the top right we have a search box that filter all columns in the client side.
- All columns are sortable.
Javascript sourced data Example
jQuery DataTable have many ways for data sources, via HTML, Ajax, Javascript and Server-side processing.
To initiate the DataTable from Javascript variable, we need to create an array of data and then passed to the DataTable like the following:
var dataSet = [ ['Trident','Internet Explorer 4.0','Win 95+','4','X'], ['Trident','Internet Explorer 5.0','Win 95+','5','C'], ... ]; $(document).ready(function() { $('#MyDT').DataTable( { "data": dataSet, "columns": [ { "title": "Engine" }, { "title": "Browser" }, { "title": "Platform" }, { "title": "Version", "class": "center" }, { "title": "Grade", "class": "center" } ] }); });
A <table>
must be available on the page for DataTables to use.
So in your HTML put:
<table id="MyDT" class="display" cellspacing="0" width="100%"></table>
You can see in this example that no records are putted into table in the HTML file, the data are set in a variable dataSet
and passed to the DataTable by the attribute "data"
, this array should be contain same number of elements in each record. Also we’ve add the attribute "columns"
that take an array of objects for length
equal to the length of elements in record, each object inside this array have a "title"
key that appear on top of each column in the table.
Conclusion
These are 2 simple examples about using jQuery DataTable, however there are many plugins that does the same job but in different way. If you need to create an enterprise web application or maybe a simple web application and you need to manage your data simplicitly, I recommend you to use jQuery DataTable because it have hundreds examples in the official website and over the internet, it’s very well supported and it have many useful extensions for advanced needs.
Download the source code for jQuery DataTable
This was an example of jQuery Data Grid control.
You can download the full source code of this example here : jQuery_Data_Grid_Contol_Example
Question: Can the data be imported from Excel, XML, etc. files? Right now it is all in the index.html in table records. What about writing back to those formats. Thanks in advance.
-SQ
Yes of course, you can write a server side function (php, jsp) to read your data from XML, Excel files then output data as JSON format.
$(‘#DT’).DataTable( {
“ajax”: ‘../url/read/data’
} );
Try also FancyGrid – JavaScript Grid Library.
http://FancyGrid.com