jQuery Http Get / Post Example
The aim of this example is to present and use the jQuery Http Get and Post methods. The jQuery get()
and post()
methods are used to request data from the server with an HTTP GET or POST request.
These are the two commonly used methods for a request-response between a client and server. GET requests data from a specified resource while POST submits data to be processed to a specified resource. GET is basically used for just getting (retrieving) some data from the server.
It may also return cached data. POST can also be used to get some data from the server. However, the POST method NEVER caches data, and is often used to send data along with the request.
1. Basic Document Setup
To begin, create a new HTML file with the basic syntax inside and also a new PHP file which will be used for testing:
main.html
<!DOCTYPE html> <html> <head> <title>jQuery HTTP Post/Get Example</title> </head> <body> <!-- HTML SECTION --> <!-- JAVASCRIPT SECTION --> <script src="jquery-1.11.3.min.js"></script> <script type="text/javascript"> </script> </body> </html>
Don’t forget to include the jQuery link, which in this case is linked locally.
process.php
<?php // php code goes here ?>
Now, we’re ready to explain and apply both methods.
2. jQuery POST Method
The jQuery POST method loads a page from the server using a POST HTTP request, and returns XMLHttpRequest object.
The syntax used is: $.post( url, [data], [callback], [type] )
where:
- url − A string containing the URL to which the request is sent
- data − This optional parameter represents key/value pairs or the return value of the
.serialize()
function that will be sent to the server. - callback − This optional parameter represents a function to be executed whenever the data is loaded successfully.
- type − This optional parameter represents a type of data to be returned to callback function: “xml”, “html”, “script”, “json”, “jsonp”, or “text”.
Let’s now see an example where we use the method with predefined values like so:
process.php
<?php if( $_REQUEST["name"] ) { $name = $_REQUEST['name']; echo "Hi there, ". $name; } ?>
In HTML, we’d have a message and a button to trigger the method:
main.html
<!-- HTML SECTION --> <p>Click on the button to see the result.</p> <p id = "message">Message Here</p> <button id = "btn">Load Data</button>
main.html
<!-- JAVASCRIPT SECTION --> <script src="jquery-1.11.3.min.js"></script> <script type = "text/javascript"> $(document).ready(function() { // wait for document to fully load $("#btn").click(function(event){ // capture a click on the button $.post( // define a post method "process.php", // the url where info is going to be posted { name: "Fabio" }, // data function(data) { // callback function $('#message').html(data); // add result to the p with id="message" } ); }); }); </script>
The result would be:
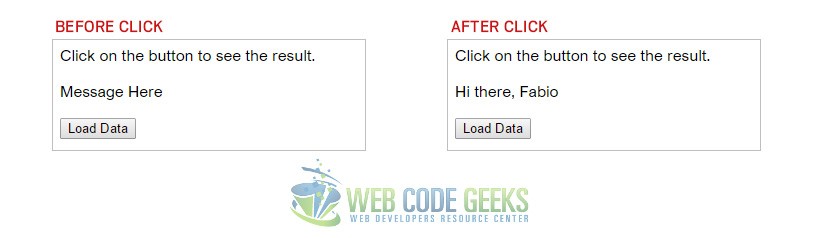
3. jQuery GET Method
The jQuery GET method loads data from the server using a GET HTTP request. The method returns XMLHttpRequest object. The most basic syntax of the method is: $.get( url, [data], [callback], [type] )
where:
- url − A string containing the URL to which the request is sent
- data − This optional parameter represents key/value pairs that will be sent to the server.
- callback − This optional parameter represents a function to be executed whenever the data is loaded successfully.
- type − This optional parameter represents a type of data to be returned to callback function: “xml”, “html”, “script”, “json”, “jsonp”, or “text”.
The example of GET pretty similar. Let’s have the same php file:
process.php
<?php if( $_REQUEST["name"] ) { $name = $_REQUEST['name']; echo "Hi there, ". $name; } ?>
Now the only thing that is going to change is the method. Whoa! Why is that, isn’t any other differences? Of course there is. Continue reading below to find out the differences.
main.html
<!-- JAVASCRIPT SECTION --> <script src="jquery-1.11.3.min.js"></script> <script type = "text/javascript"> $(document).ready(function() { // wait for document to fully load $("#btn").click(function(event){ // capture a click on the button $.get( // define a post method "process.php", // the url where info is going to be posted { name: "Fabio" }, // data function(data) { // callback function $('#message').html(data); // add result to the p with id="message" } ); }); }); </script>
Needles to say, the result would be exactly the same. Now let’s consider talking about what are the differences about these two methods, which seem to be producing the same result.
4. Differences between POST & GET
There are quite considerable distinctions between POST & GET. The following table is an essential overview:
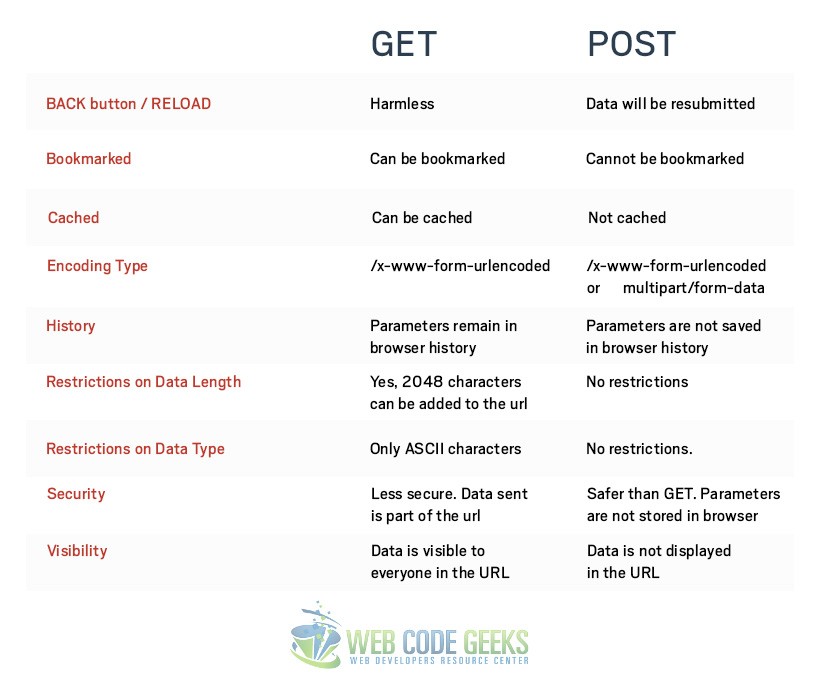
5. Conclusion
The Hypertext Transfer Protocol (HTTP) is designed to enable communications between clients and servers. HTTP works as a request-response protocol between a client and server. A web browser may be the client, and an application on a computer that hosts a web site may be the server. So, which one should you use? Use POST for destructive actions such as creation, editing, and deletion, because you can’t hit a POST action in the address bar of your browser. Use GET when it’s safe to allow a person to call an action.
6. Download the source code
This was an example about JQuery Http Get / Post.