jQuery remove class example
Very often on a webpage we need to change the visual representation of a DOM element to display some non-text information like changing a color to red that would mean some error or warning, or probably changing parameters of an element on mouseenter event.
All of this can be implemented using an elegant combination of css and javascript.
But practically every time you end up with having too much style options, you group them into classes, and then you run into a large heap of classes, which is hard to maintain.
It’s time when jQuery can help you! Because it provides a very simple API to deal with classes. Let’s look at an example.
1. HTML
First we’ll introduce an HTML code. Here we have a div
and a span
which contains a bit of text. Both of them have some classes described in style.css file. On button click event we’ll perform some actions to change colors or shapes or anything else.
<!DOCTYPE html> <html> <head> <title>JQuery remove class example</title> <link rel="stylesheet" href="style.css"> <script src="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9jb2RlLmpxdWVyeS5jb20vjquery-2.1.3.min.js"></script> <script src="js.js"></script> </head> <body> <div class="centered yellowed rectangled"> <span class="bordered blocked centeredText"> I'm so stylish! </span> <button onclick="changeSomething();" class="bordered">Change background</button> </div> </body> </html>
2. CSS
In css file we’ll define already used in html custom classes:
centered
yellowed
rectangled
bordered
blocked
centeredText
.rectangled { width: 400px; height: 100px; } .centeredText { text-align: center; } .centered { margin: 0 auto; } .bordered { border: 3px solid green; } .blocked { display: block; } .yellowed { background: yellow; }
3. Javascript
On button click we’ll just change the color of the div by removing yellowed
class
function changeSomething() { $("div").removeClass("yellowed"); }
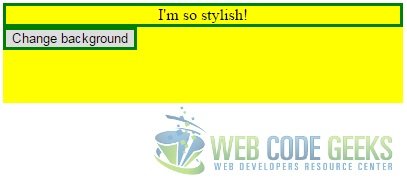
In your browser you should see something like this
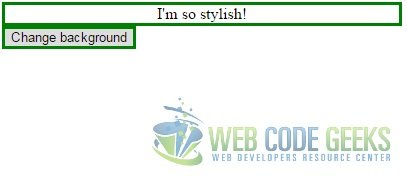
4. Modifying several elements
You should notice that these class modifications may influence not only one element, but everything you select. For example we could add one more div
to the body
<div class="centered rectangled greened"> This is the second div </div>
And of course implement a new style class
.greened { background: green; }
The Javascript function changeSomething()
will now contain another piece of code
$(".centered").removeClass("centered");
After implementing these changes and reloading the page you will see that both divs move to the left side of the window after button click.
5. Toggling
In some cases we don’t need to add or remove different style classes but just one single class. JQuery allows us to use toggleClass
method. It’s pretty useful because you don’t have to care if the class is present or not. Let’s create a little checkbox. In HTML we’ll need two nested divs
<!DOCTYPE html> <html> <head> <title>JQuery remove class example</title> <link rel="stylesheet" href="style.css"/> <script src="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9jb2RlLmpxdWVyeS5jb20vjquery-2.1.3.min.js"></script> <script src="js.js"></script> </head> <body> <div id="toggle" class="centered rectangled bordered"> <div id="inner" class="rectangled yellowed"></div> </div> </body> </html>
The stylesheet code can be reduced a little
.rectangled { width: 10px; height: 10px; } .centered { margin: 0 auto; } .bordered { border: 3px solid green; } .yellowed { background: yellow; }
And in javascript code we’ll add $(document).ready
just to be sure the DOM is ready and we can place script
tag wherever we want
$(document).ready(function() { // waits until DOM is ready $("#toggle").click(function() { // selects outer div $("#inner").toggleClass("yellowed"); // toggles class of inner div }); });
6. Removing multiple classes
removeClass
method allows you to remove several classes at the same time. It can be implemented using different approaches.
You may just provide a list of classes passing them as parameters. Consider the following javascript code in the previous example (it will only “uncheck” the checkbox)
$(document).ready(function() { $("#toggle").click(function() { $("#inner").removeClass("yellowed", "rectangled"); }); });
Or you may pass a single string parameter which contains a list of classes to remove separated with a space
$(document).ready(function() { $("#toggle").click(function() { $("#inner").removeClass("yellowed", "rectangled"); }); });
Or you may even provide a function that returns such list if you need to prepare it dynamically (since jQuery 1.4)
$(document).ready(function() { $("#toggle").click(function() { $("#inner").removeClass(getClasses); }); }); function getClasses() { return "yellowed rectangled"; }
7. Removing or replacing all classes
Sometimes you’ll probably need to remove or replace all the classes of an element. For this case jQuery provides a very convenient way. Let’s continue with the same HTML and CSS and add one more class
.reded { background: red; }
Depending on your needs you can use one of these approaches
$(document).ready(function() { $("#toggle").click(function() { $("#inner").removeClass(); // removes all the classes }); });
$(document).ready(function() { $("#toggle").click(function() { $("#inner").removeClass().addClass("rectangled reded"); // removes all the classes and adds the given ones }); });
$(document).ready(function() { $("#toggle").click(function() { $("#inner").attr("class", "rectangled reded"); // replaces all the classes with the given ones }); });
8. Download
You can download the full source code of this example here: JQueryRemoveClass