Exports vs module.exports in NodeJs, Which one to use?
We know that when our code is run through the node it’s wrapped in a function expression. This enables us to access the module object which is a parameter passed to that function.
1
2
3
|
( function (exports, require , module, __filename, __dirname) { //module code }); |
This function expression is then invoked with the following arguments:
1
|
fn(module.exports, require , module, filename, dirname); |
So we can see that in beginning both exports
and module.exports
point towards the same memory where the empty module.exports
{}
reside.

When we require a module using require("module_name")
, we get module.exports
in return and not exports
. This must always be kept in mind.
So when we do something like following in our module sample_module.js:
1
2
3
4
5
6
|
//sample_module export.prop1 = "This is property 1; export.func1 = function (){ console.log( "This is function 1" ); } |
Property and a method is being added to the object which is also pointed towards by module.exports
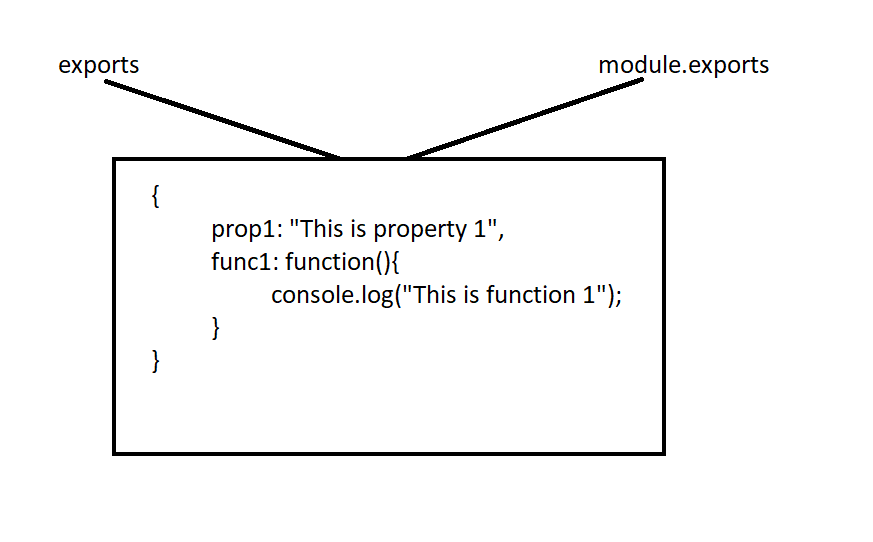
Hence when we require sample_module:
1
2
3
4
|
//app.js var sample = require ( "sample_module" ); sample.prop1; //This is property 1 sample.func1; //logs "This is function 1" |
Of course, this is the same result we’d have got had we used module.exports
in sample_module instead of exports
.
However, if we have to export a number instead of an object and we do
1
2
|
//sample_module exports = 5; |
The situation would be like
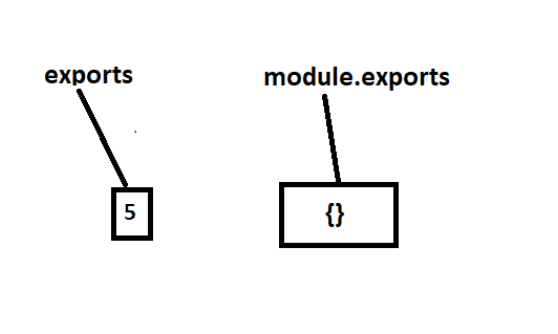
On trying to access this from app.js:
1
2
3
4
|
//app.js var sample = require ( "sample_module" ); typeof(sample); //object... not number conosle.log(sample); //will log an empty object |
This is happening because what actually gets returned via require() function is module.exports
; and in the above example, we’ve set exports = 5
, which essentially doesn’t mutate the object being pointed towards by module.exports
, but instead, assign a new memory location to exports
and set its value as 5;
This has nothing to do with node. In Javascript, objects are passed by “copy of a reference”. So when the wrapped module is executed, exports
got a copy of reference of module.exports
. So exports
can modify the content of module.exports
via the reference it received. However, if exports
tries to overwrite the reference(as in the above example), it will not alter module.exports
but rather create a new memory location for exports
and add content to that.
So what actually needs to be done for the above code to be properly executed is:
1
2
|
//sample_module module.exports = 5; |
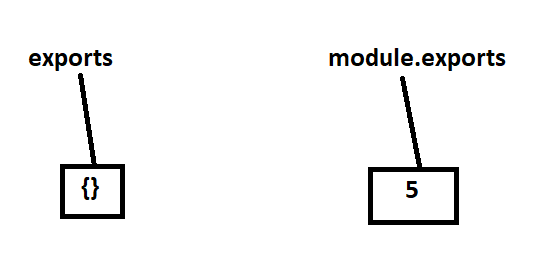
1
2
3
4
|
//app.js var sample = require ( "sample_module" ); typeof(sample); //number conosle.log(sample); //5 |
My personal advice is to always use module.exports
and forget that shorthand exports
exists. This will reduce your cognitive load of remembering these minute details and make life a lot more easier.
Published on Web Code Geeks with permission by Himansh Jain, partner at our WCG program. See the original article here: exports vs module.exports in NodeJs, Which one to use? Opinions expressed by Web Code Geeks contributors are their own. |