PHP Google Maps Example
According to wikipedia Google Maps is a web based mapping service developed by Google. It offers satellite imagery, street maps, 360° panoramic views of streets (Street View), real-time traffic conditions (Google Traffic), and route planning for traveling by foot, car, bicycle (in beta), or public transportation.
Google Maps provides detailed information about geographical regions and sites around the world. In this example we will create a web app with google map embeded.
For this example we will use:
- A computer with PHP >= 5.5 installed
- notepad++
1. Getting Started
Google maps allow access to its mapping service in five different ways. Those are:
- Google Maps Javascript API: This allows you to access google maps service through javascript and according to its website it features a robust support.
- Google Maps embed API: You do not need any working knowledge of javascript to access Google Map in this way because you do not need to write any code. Just enter the neccessary location and copy the given code.
- Google Street View Image Api
- Google Static Map Api: Embed a map image without JavaScript or dynamic page loading. With this method you embed a map image and you dont need javascript to do it.
- Google Places API Javascript Library: Up-to-date information about millions of locations.
For this example we are going to use the Google Maps Javascript API. Lets get started.
1.1 Google Maps Javascript AP
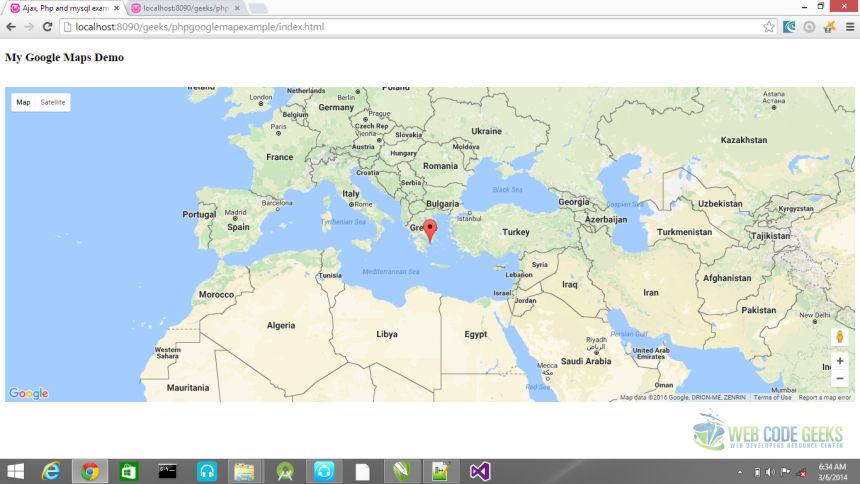
index.html
<!DOCTYPE html> <html lang=en> <head> <style> #map { height: 400px; width: 100%; } </style> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0"/> <title> Google Maps Demo </title> </head> <body> <h3> Google Maps Demo</h3> <div id=map style="width:100%;height:500px"></div> <script> function initMap() { var uluru = {lat: 37.59, lng: 23.44}; var map = new google.maps.Map(document.getElementById('map'), { zoom: 4, center: uluru }); var marker = new google.maps.Marker({ position: uluru, map: map }); } </script> <script async defer src="http://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap"> </script> </body > </html>
The script above shows the map of Athens, Greece.
Note: For this script to work in your browser you need to:
- Make sure to get an api key for your web app. You need the key to access Google Map API service. To get an Api key for google map please vist Google console and follow the instructions.
- Always make sure you have a valid div tag or element that will be used by Google map object. You should always set the height and width property of your div.
If you don’t follow the instructions above when implementing your web app, unexpected errors that are difficult to debug or even trace will occur. In line 35 we load the Google Map API script.
<script async defer src="http://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap"> </script>
The async attribute allows the browser to continue rendering the rest of your page while the API loads. We pass our API key and add a callback function ( The callback function is called after the script has loaded completely ). In this case the function name is initMap
.
In line 22 we create an object which holds the coordinates of the region we want displayed. In line 23 we instatiate the map object. We pass our div tag, the zoom value and our coordinate object. In line 27 we create a marker object and pass our map to it. Thats all it takes to create our google map.
Lets look at another example.
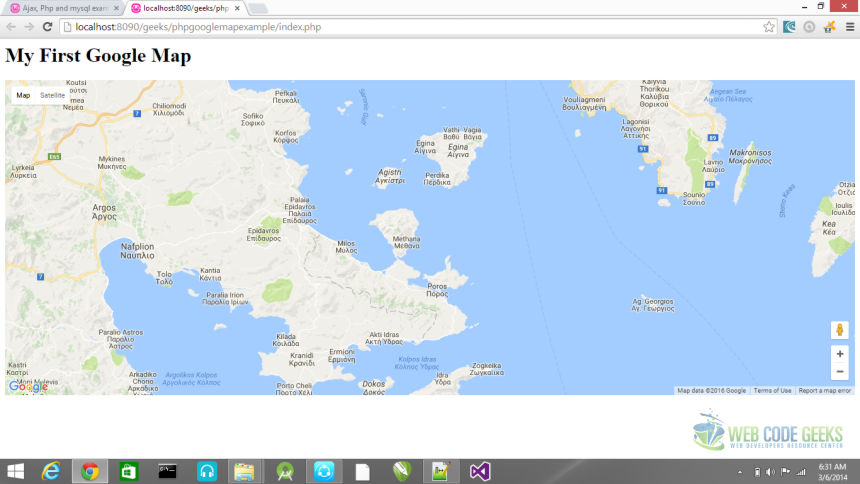
index.php
<!DOCTYPE html> <html> <body> <h1>My First Google Map</h1> <div id=map style="width:100%;height:500px"></div> <script> function myMap() { var mapCanvas = document.getElementById("map"); var mapOptions = { center: new google.maps.LatLng(37.59, 23.44), zoom: 10 } var map = new google.maps.Map(mapCanvas, mapOptions); } </script> <script src="http://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=myMap"></script> </body> </html>
The script above displays a map without a marker. If you didnt notice, we changed the zoom level from 4 to 10. This zooms into the map. Try experimenting with different options of the Google Map API and see the results.
Note: For the examples in this project to work you need to get an API key, this key allows you to successfully access the Google Maps API.
2. Summary
In this example we learnt about Google Maps, and the different ways we can access the Google Map service. We also learnt how to access the Google maps Api with javascript.
3. Download the source code
You can download the full source code of this example here: phpgooglemapexample
Excellent article, but php is not needed. You gave us two great examples and both are static web pages. Why was this article categorized under PHP? It should have been under Javascript.