HTML5 Accordion Example
In this article we will build an Accordion using HTML5 and a dash of JavaScript. The Accordion widget is quite useful when we want to present just a teaser at first and if this interests the user, we provide detailed information. Basically we have content which collapses or starts out as such allowing the user to expand and get into details if the teaser information tempts them.
1. Introduction
An accordion, also referred to as a Collapsible, allows us to accommodate large content in a way that makes it easy for the user to go directly to things of interest. With an accordion we hide verbose content initially till the user clicks on a teaser to reveal the entire content and in the same way the user is able to hide content by clicking on the teaser. This widget allows us to efficiently use the screen real estate. Some implementations allow for only one content node to be expanded at a time. In our example we are going to let the user decide on this point.
2. Tools & Technologies
- HTML5
- CSS3
- JavaScript
- Nodejs v6.3.0
- Express
- Visual Studio Code IDE
NodeJS, in essence, is JavaScript on the server-side. The Express module is used to create a barebones server to serve files and resources to the browser. I have used the Visual Studio Code IDE since I am the most familiar with it although you could others as per preference.
3. Project Layout
The layout of our project is as shown in the screen grab below:
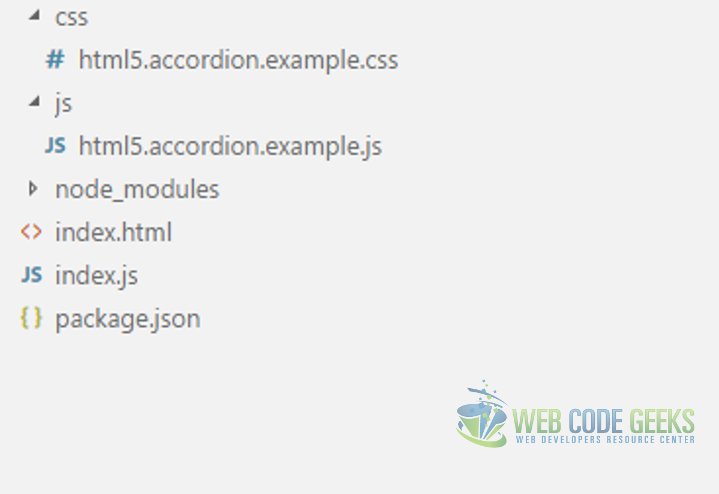
CSS Folder
This folder hosts our CSS files, namely, html5.accordion.example.css
. We stylize our UI with classes in this file.
JS Folder
Our client side JavaScript files reside here. These are referenced in our HTML page(s).
index.js
I have used NodeJS
and Express
module to spin up a barebones Web Server for this example.
index.html
This is our Markup file, it hosts the UI of this example.
4. Creating HTML Markup
We start out with a basic HTML5 page and modify it as per our needs. The structure of markup for the accordion looks like below:
index.html
... <div class="accordion"> <div class="accordion-item"> <div class="item-header"> First Header <span>⇩</span> </div> <div class="item-content"> <p> Content of the First Item. Content of the First Item. Content of the First Item. Content of the First Item. Content of the First Item. </p> </div> </div> <div class="accordion-item"> <div class="item-header"> Second Header <span>⇩</span> </div> <div class="item-content"> <p> Content of Second Item. Content of Second Item. Content of Second Item. Content of Second Item. Content of Second Item. </p> </div> </div> <div class="accordion-item"> <div class="item-header"> Third Header <span>⇩</span> </div> <div class="item-content"> <p> Content of the Third Item. Content of the Third Item. Content of the Third Item. Content of the Third Item. Content of the Third Item. </p> </div> </div> ...
5. CSS Classes
Now that we have our markup ready we will stylize the same with the help of our css file. We add classes to collapse all our item-content
divs initially. We spruce up the UI by adding colors and some padding and margin as required. Our CSS file needs to look like this:
html5.accordion.example.css
.accordion{ max-width: 50%; } .item-header{ background-color: darkblue; color: whitesmoke; min-height: 20px; border: 4px; border-color: lightblue; } .item-content{ background-color: whitesmoke; border-color: darkblue; border-radius: 12px; display: none; } .item-header span{ padding-right: 5px; margin-bottom: 0px; margin-top: 0px; float: right; } .item-content p{ margin-top: 0px; margin-bottom: 0px; padding-top: 2px; padding-bottom: 2px; }
Finally we write the JavaScript to make things tick. We do so in our JavaScript file, viz., html5.accordion.example.js
. Our client side JavaScript should look like below:
html5.accordion.example.js
var accordion = document.getElementById('accordion'); var accordion_items = document.getElementsByClassName('accordion-item'); var item_headers = document.getElementsByClassName('item-header'); var item_contents = document.getElementsByClassName('item-content'); function setupAccordion(){ Array.prototype.forEach.call(item_headers, (function(header){ header.addEventListener("click", function(evt){ var src = evt.srcElement; if(src.nextElementSibling.style.display === "none" || !src.nextElementSibling.style.display){ expandItem(evt); } else { collapseItem(evt); } }); })); } function expandItem(evt){ var src = evt.srcElement; src.nextElementSibling.style.display = "block"; } function collapseItem(evt){ var src = evt.srcElement; src.nextElementSibling.style.display = "none"; } setupAccordion();
In the above JavaScript we bind an event handler to each item-header
div to toggle visibility of the corresponding content node. We write code for this in the click
event handler.
6. Running the Project
Now that we have everything setup let us go ahead and run the project and look at the output. Firstly, we need to run the following commands at the root of the project.
>npm install
and
>node index.js
Now we need to navigate to the URL http://localhost:8090/index.html
, We should see the below screen:
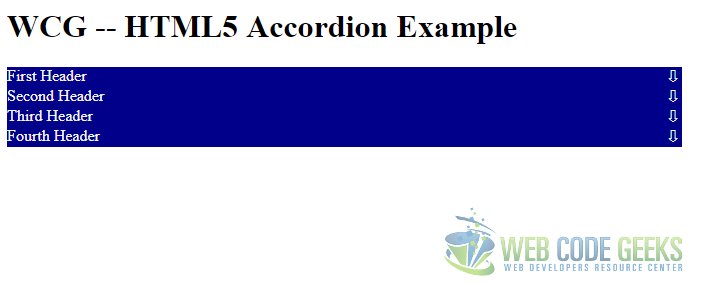
If we click on any of the header elements it leads to the appearance of the content below it. The screen should look like below:
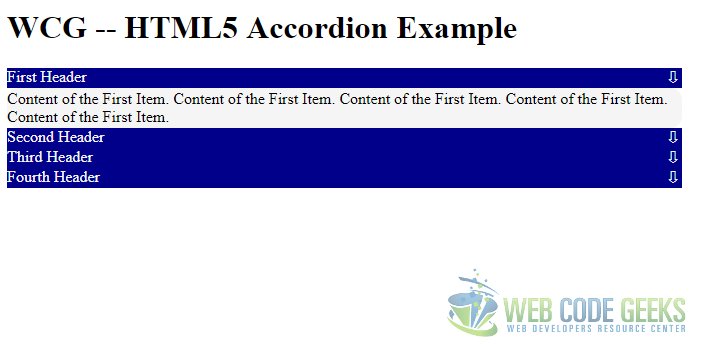
7. Download the Source Code
That was HTML5 Accordion Example.
You can download the full source code of this example here: WCG — HTML5 Accordion Example