HTML5 Video Tag Example
The aim of this example is to introduce and show the usage of the video
tag of HTML5.
Before HTML5, there was no standard for showing videos on a web page and videos could only be played with a plug-in (like flash).
The HTML video
element is used to embed video content. It may contain several video sources, represented using the src attribute or the element; the browser will choose the most suitable one.
1. Basics
1.1 Initial Document Setup
Go ahead and create a new html document and add the basic sytnax in it like so:
<!DOCTYPE html> <html> <head> <title>HTML5 Video Tag Example</title> </head> <body> <!-- STYLE SECTION --> <style type="text/css"> </style> <!-- HTML SECTION --> </body> </html>
1.2 Understanding the Attributes
autoplay
A Boolean attribute; if specified, the video will automatically begin to play back as soon as it can do so without stopping to finish loading the data.
buffered
An attribute you can read to determine which time ranges of the media have been buffered. This attribute contains a TimeRanges object.
controls
If this attribute is present, Gecko will offer controls to allow the user to control video playback, including volume, seeking, and pause/resume playback.
height
The height of the video’s display area, in CSS pixels.
loop
A Boolean attribute; if specified, we will, upon reaching the end of the video, automatically seek back to the start.
muted
A Boolean attribute which indicates the default setting of the audio contained in the video. If set, the audio will be initially silenced. Its default value is false, meaning that the audio will be played when the video is played.
played
A TimeRanges object indicating all the ranges of the video that have been played.
poster
A URL indicating a poster frame to show until the user plays or seeks. If this attribute isn’t specified, nothing is displayed until the first frame is available; then the first frame is displayed as the poster frame.
src
The URL of the video to embed. This is optional; you may instead use the element within the video block to specify the video to embed.
width
The width of the video’s display area, in CSS pixels.
1.3 Media Formats Supported
1. WebM
The WebM format is based on a restricted version of the Matroska container format. It always uses the VP8 or VP9 video codec and the Vorbis or Opus audio codec. WebM is natively supported in desktop and mobile Gecko (Firefox), Chrome and Opera, and support for the format can be added to Internet Explorer and Safari (but not on iOS) by installing an add-on.
video/webm
A WebM media file containing video (and possibly audio as well).
2. Ogg Theora Vorbis
The Ogg container format with the Theora video codec and the Vorbis audio codec is supported in desktop/mobile Gecko (Firefox), Chrome, and Opera, and support for the format can be added to Safari (but not on iOS) by installing an add-on. The format is not supported in Internet Explorer in any way.
WebM is generally preferred over Ogg Theora Vorbis when available, because it provides a better compression to quality ratio and is supported in more browsers. The Ogg format can however be used to support older browser versions (for example Firefox 3.5/3.6 don’t support WebM, but do support Ogg.)
video/ogg
An ogg file containing video (and possibly also audio).
3. MP4 H.264
The MP4 container format with the H.264 video codec and the AAC audio codec is natively supported by desktop/mobile Internet Explorer, Safari and Chrome, but Chromium and Opera do not support the format. IE and Chrome also support the MP3 audio codec in the MP4 container, but Safari does not. Firefox/Firefox for Android/Firefox OS supports the format in some cases, but only when a third-party decoder is available, and the device hardware can handle the profile used to encode the MP4.
1.4 A Basic Application
A very basic application where we insert a video into a webpage would be the following:
<!-- HTML SECTION --> <video src="videos/minions.mp4" controls></video> <!-- src to locate the video, controls attribute to show video controls -->
In the top left side of your browser the video would show and require to press the play button to start:
1.5 HTML5 Video Browser Support
The following graphics show the support given to HTML5 video and its features by browsers.
2. Cases and Examples
2.1 Include Various Formats
There are three video formats that work natively in some browsers. Unfortunately, no format works in all browsers, so you need to do at least two if you want meaningful HTML5 video support.
<!-- HTML SECTION --> <video controls> <source src="videos/minions.mp4" type="video/mp4"> <source src="videos/minions.webm" type="video/webm"> <source src="videos/minions.ogg" type="video/ogg"> </video>
Notice we used the source
tag to add videos of different formats, and not inside the video tag as an attribute.
Minimally, you must use MP4 + H.264, with AAC or MP3. MP4 video plays natively in Safari, Chrome, and IE9 (Vista/Windows 7). It is also your best option for a Flash video fallback, and plays natively on many devices (iOS, Android, Blackberry, PSP, Xbox, PS3, etc.). Use H.264 High Profile for the best quality, or Baseline profile if you want the same video to be playable on mobile devices.
Beyond that, use either WebM + VP8 or Ogg + Theora with Vorbis audio for other browsers. WebM works in Firefox (4+), Chrome (6+ or Chromium), and Opera (10.60+), and Ogg works in Firefox (3.5+), Chrome (3+), and Opera (10.54+).
The result in the browser woulld be just the same video but compatible (able to be read) by all browsers.
2.2 Custom Video Size
Attributes (most of which we mentioned and explained in the first section) are very useful when considering a customized video element that fits your needs inside the page. So in this section, we’ll have a look at how we can use the various attributes offered for the video element to enhance our content.
<!-- HTML SECTION --> <!-- added a custom width and height for the video size and also autoplay --> <video width="640px" height="320px" autoplay controls> <source src="videos/minions.mp4" type="video/mp4"> <source src="videos/minions.webm" type="video/webm"> <source src="videos/minions.ogg" type="video/ogg"> </video>
The result would be a scaled video size that would automatically play as soon as the webpage is opened.
2.3 Using a Custom Poster for the Video
Attributes also provide us a very cool feature called the poster
. It gives the video an inital default image to display before being played.
<!-- HTML SECTION --> <!-- added a 'poster' attribute and specified the location of the poster image --> <video width="856px" height="480px" poster="image.jpg" controls> <source src="videos/minions.mp4" type="video/mp4"> <source src="videos/minions.webm" type="video/webm"> <source src="videos/minions.ogg" type="video/ogg"> </video>
The result would be a poster image overlaying our video.
2.4 Fullscreen HTML5 Video
When talking about fullscreen video of HTML5, it all comes down to CSS (unless you want to use javascript). You can actually override any attributes regarding the size of the video and force a video to display fullscreen like this:
<!-- HTML SECTION --> <video width="856px" height="480px" autoplay> <source src="videos/minions.mp4" type="video/mp4"> <source src="videos/minions.webm" type="video/webm"> <source src="videos/minions.ogg" type="video/ogg"> </video>
Applying this CSS snippet will make it possible:
<!-- STYLE SECTION --> <style type="text/css"> video { position: fixed; top: 50%; left: 50%; min-width: 100%; min-height: 100%; width: auto; height: auto; z-index: -100; -webkit-transform: translateX(-50%) translateY(-50%); transform: translateX(-50%) translateY(-50%); } </style>
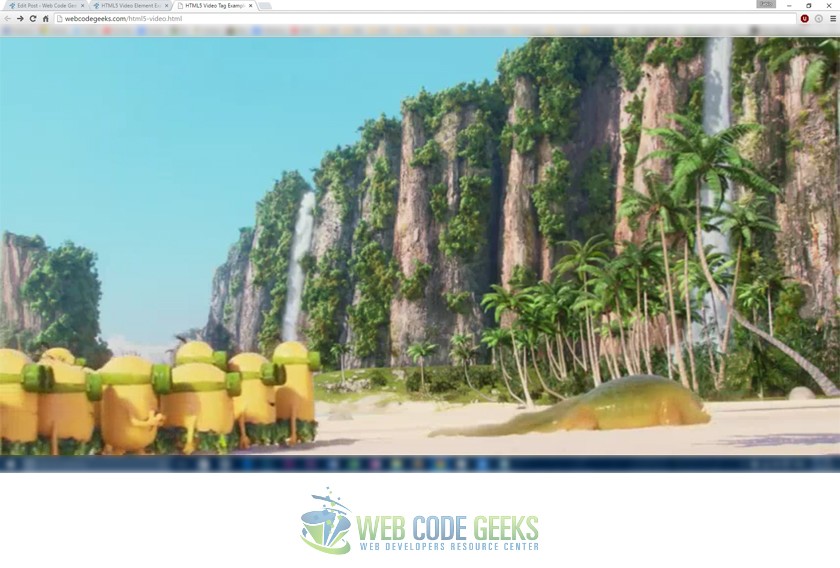
3. Conclusion
To conclude, HTML5 video is a quick and easy way to add video content to websites. It provides full compatibility with browsers and has a solid mobile device support. Built in video/audio playback make it useless to consider third party players like before with quicktime, flash and silverlight (even though there are certain advantages why some pages still use them).
Customization is pretty good for now and provides a very clean code. Do remember to convert videos into the right formats for all-browser compatibility, and shrink them in size as much as you can to prevent a slow page loading experience to the user.
4. Download
You can download the full source code of this example here: HTML5 Video Tag