Angular.js Transclusion Example
There is a myth that wants transclusion to be a hard concept to understand and manage, according to Angular.js. So, for today, we’ll wear the hat of Mythbusters, by displaying several simple examples of transclusion in Angular.js.
1. Introduction
First things first, which means that we have to understand the term itself, in order to delve into tougher/technical questions that may occur.
Transclusion is generally the inclusion of the content of a document into another document by reference.
1.1 Transclusion Definitions
In other words, one could say “it’s all about templating; specifically, when you want to include some content from one template to another”, but I’m sure, there are still some logical gaps, so, I hereby quote several transclusion definitions, in order to ensure everyone’s in the same page, before displaying a single line of code:
- a directive (with a template) that either wraps, or is wrapped by end user code
- a setting to tell angular to capture everything that is put inside the directive in the markup and use it somewhere (where actually the
ng-transclude
is at) in the directive’s template. - provides a way to pass in templates to a directive and it is displayed
- it allows you to place HTML inside of a directive’s declaring HTML to be used within the directives template
- is used to insert the original contents of a directive’s element into a specified place in the template of the directive
After reading the above, the very first output is “what are directives?”, but this is covered in the following section, as I will hereby stick with the official definition:
Transclusion is the process of extracting a collection of DOM elements from one part of the DOM and copying them to another part of the DOM, while maintaining their connection to the original AngularJS scope from where they were taken.
1.2 Angular Directives
Directives are markers on a DOM element (attributes, CSS classes, names of elements, comments) that tell Angular’s HTML compiler to attach a specific behavior to that DOM element (i.e through event listeners), or even to transform the DOM element and its children. Some of the built-in Angular directives are: ngBind
, ngModel
and ngClass
.
In addition to all the built-in Angular.js directives, you can also create your own directives, by using the .directive
function.
Such directives look like this, on their html part:
<!DOCTYPE html> <html> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <script src="second.js"></script> <body ng-app="app"> <div test-directive></div> Hello from page </body> </html>
whereas the javascript part is like:
var app = angular.module("app", []); app.directive("testDirective", function() { return { restrict : "EA", template : "<h1>Hey, I'm the directive's content!</h1>" }; });
As expected, the output firstly contains the template inserted from the directive and then the actual html content of the page itself:

Did you notice the transition between the camel-case, used in the js part and the dash (“-“), when on html, according to the directive’s reference?
There are some points here, according to directives:
- A camel-case name must be used for naming a directive (js), but for invoking it (html), a dash (“-“) separated name must be used (i.e.
testDirective
->test-directive
). - The
restrict
property is used to restrict the directive’s invocation by some of the existing methods. - The
template
property is used to define the html content that the specified directive will contain.
For instance, the legal restrict values are:
E
for element’s nameA
for attributeC
for classM
for comment
By default, the restrict
property has a value of EA
, which means that both element names and attribute names can invoke the directive.
2. Transclusion in Angular.js
In Angular.js, transclusion is used with the ngTransclude
directive to insert the original contents of a directive’s element into a specified place in the template of the directive.
That is, it can be used within the directive’s template, which provides better modularity on the directive itself, when having to handle lots of templates for different purposes.
Here a simple transclusion directive, where the HTML paragraph is used within its own template, using ngTransclude
:
<my-transclusion-directive> <div>I am the transcluded element. I have transclude property enabled.</div> </my-transclusion-directive>
.directive('myTransclusionDirective', function() { return { restrict: 'EA', scope:{}, //isolate scope. transclude:true, template: "<div ng-transclude></div><div>My first transclusion directive.</div>" }; });
The above transcluded directive will place <div>I am the transcluded element. I have transclude property enabled.</div>
inside the div marked with ng-transclude
in the directive’s code.
3. Today’s Example
So, here’s the today’s example, according to the html, js and visual part.
index.html
<!DOCTYPE html> <html> <head lang="en"> <meta charset="utf-8"> <title>AngularJS Transclusion</title> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.5/angular.min.js"></script> <script type="text/javascript" src="app.js"></script> </head> <body> <div ng-app="mainApp"> <my-transclusion-directive> <div>I am the transcluded element. I have transclude property enabled.</div> </my-transclusion-directive> </div> </body> </html>
script.js
var app = angular.module('mainApp', []); app.directive('myTransclusionDirective', function() { return { restrict: 'EA', scope:{}, //isolate scope. transclude:true, template: "<div ng-transclude></div><div>My first transclusion directive.</div>" }; });
As expected/explained from the previous paragraph, the output is like:
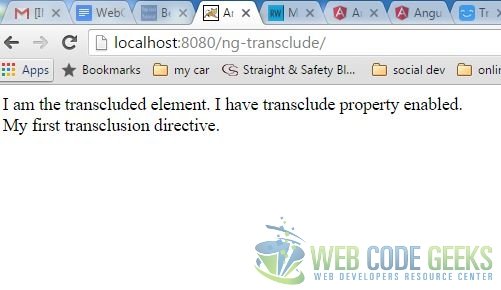
What if setting the transclude property to false? That is, the html content of the transcluded element will not be displayed. Instead, only the directive’s template will be displayed, which means:
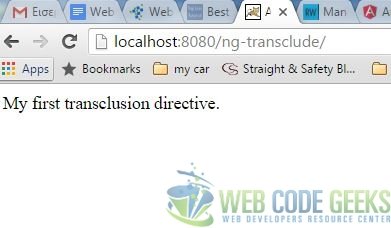
4. Download
This was an example of Angular.js Transclusion.
You can download the full source code of this example here : ng-transclude.zip.