AngularJS Data Bindings Example
Hello readers, data binding in an angular library is a data synchronization process between the Model and the View components. In this tutorial, we will see how data binding works in the angular library.
1. Introduction
Binding of data is an important feature in the UI technology. The data binding acts as a communication medium between the Model and the View components of an application. In angular, a model works as an application source and View as an angular model projection.Below are the two types of data bindings that are available in the angular library.
1.1 One-way data binding
It is a unidirectional binding where the data from a model is inserted into the HTML
elements. It is used in the old template systems where there is no automatic synchronization between the model and the view components. Thus this approach processes the data in a single direction as shown below.
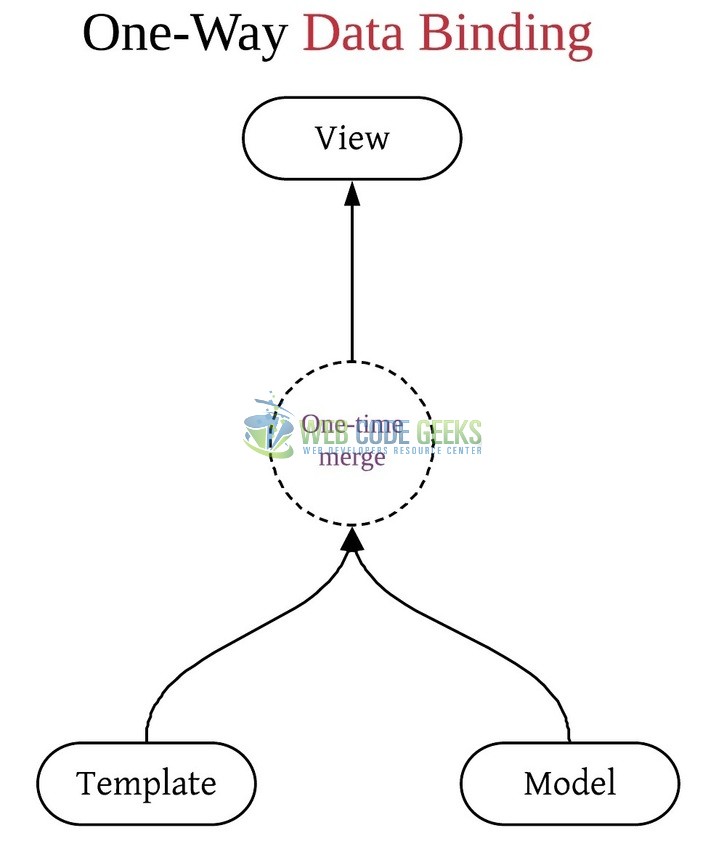
1.2 Two-way data binding
It is a bidirectional binding where the view is automatically updated as soon as the model is changed. Thus if the model is changed, the view immediately reflects the change and vice-versa. It is used in modern-day template systems and offers an automatic synchronization between the model and the view components as shown below.
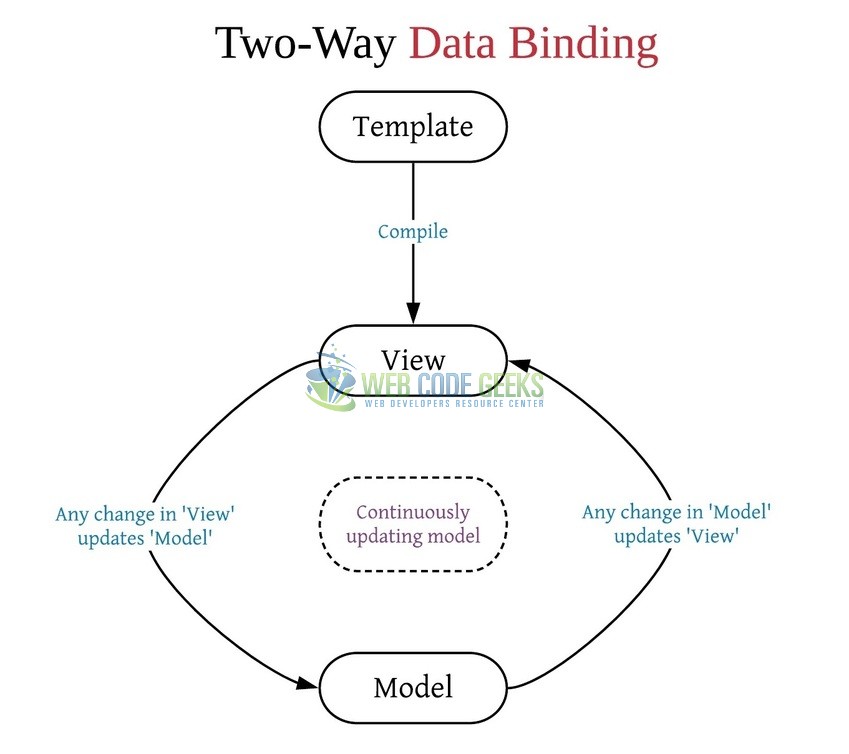
In angular, developers can make this binding by using the ng-model directive. This tag binds the value of the HTML
form controls to the application data.
1.2.1 How binding works in Angular?
In angular, the ng-mode
directive remembers the present data and compares it with the old value. A time change event is triggered automatically as soon as a change is observed in the old value and this will update the view every time the data got changed. The HTML
tells the angular compiler to create a $watch
scope for the controller methods and execute it inside the $apply
method.
These new APIs make developer’s life easier, really! But it would be difficult for a beginner to understand this without an example. Therefore, let us create a simple data binding application using the angular library.
2. AngularJS Data Bindings Example
Here is a step-by-step guide for implementing the two-way data binding in angular applications.
2.1 Tools Used
We are using Eclipse Kepler SR2, JDK 8 and Maven. Having said that, we have tested the code against JDK 1.7 and it works well.
2.2 Project Structure
Firstly, let’s review the final project structure if you are confused about where you should create the corresponding files or folder later!
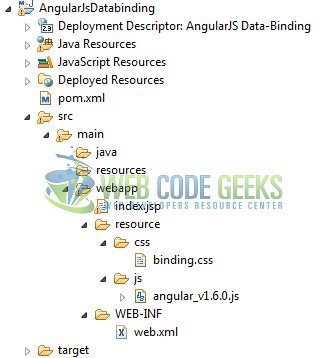
2.3 Project Creation
This section will show how to create a Java-based Maven project with Eclipse. In Eclipse Ide, go to File -> New -> Maven Project
.
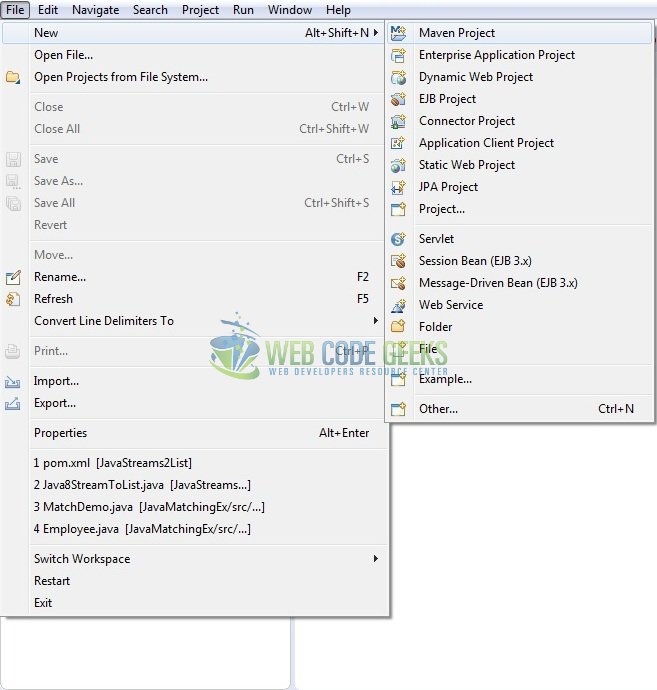
In the New Maven Project window, it will ask you to select project location. By default, ‘Use default workspace location’ will be selected. Just click on next button to proceed.
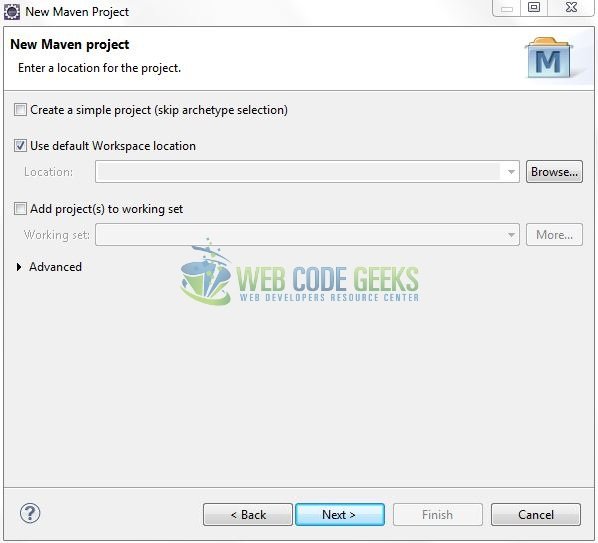
Select the ‘Maven Web App’ Archetype from the list of options and click next.
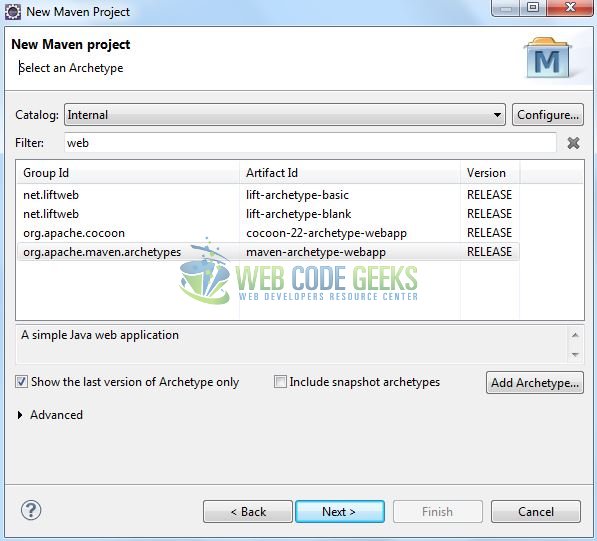
It will ask you to ‘Enter the group and the artifact id for the project’. We will enter the details as shown in the below image. The version number will be by default: 0.0.1-SNAPSHOT
.

Click on Finish and the creation of a maven project is completed. If you see, it has downloaded the maven dependencies and a pom.xml
file will be created. It will have the following code:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>AngularJsDatabinding</groupId> <artifactId>AngularJsDatabinding</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> </project>
Let’s start building the application!
3. Application Building
Let’s create an application to understand the basic building blocks of the two-way data binding in the angular library.
3.1 Load the Angular framework
Since it is a pure JavaScript framework, we should add its reference using the <script>
tag.
<script type="text/javascript" src="resource/js/angular_v1.6.0.js"></script>
3.2 Define the Angular application
Next, we will define the angular application using the <ng-init>
, <ng-model>
,and the <ng-bind>
directives.
index.jsp
<div ng-app = ""ng-init="f_name = 'Charlotte'; l_name = 'O\' Neil';"> ... </div>
3.3 Complete Application
In this example, we have defined the ng-model
directive to the HTML
input control and we are using the same ng-model
value for displaying on the output page. Complete the above steps and I will show you how to use the two-way data binding with real-life practices.
index.jsp
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>AngularJS Data-binding</title> <!-- AngularJs Javascript File --> <script type="text/javascript" src="resource/js/angular_v1.6.0.js"></script> <!-- Bootstrap Css --> <link rel="stylesheet" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/4.0.0/css/bootstrap.min.css"> <link rel="stylesheet" href="resource/css/binding.css"> </head> <body> <div id="angularBinding" class="container"> <h1 align="center" class="text-primary">AngularJS - Data-binding Example</h1> <hr /> <!------ ANGULAR BINDING EXAMPLE ------> <div ng-app="" ng-init="f_name = 'Charlotte'; l_name = 'O\' Neil';"> <div class="form-group"> <label for="fName">First name:</label><input id="fName" type="text" class="form-control" ng-model="f_name" /> </div> <div class="form-group"> <label for="lName">Last name:</label><input id="lName" type="text" class="form-control" ng-model="l_name" /> </div> <div> </div> <hr /> <!------ ANGULAR TWO-WAY BINDING ------> <p class="fontSize"> <strong>First name:</strong> <span id="first_name" class="text-success" >{{ f_name }}</span> </p> <p class="fontSize"> <strong>Last name:</strong> <span id="last_name" class="text-success" ng-bind="l_name"></span> </p> </div> </div> </body> </html>
4. Run the Application
As we are ready for all the changes, let us compile the project and deploy the application on the Tomcat7 server. To deploy the application on Tomat7, right-click on the project and navigate to Run as -> Run on Server
.
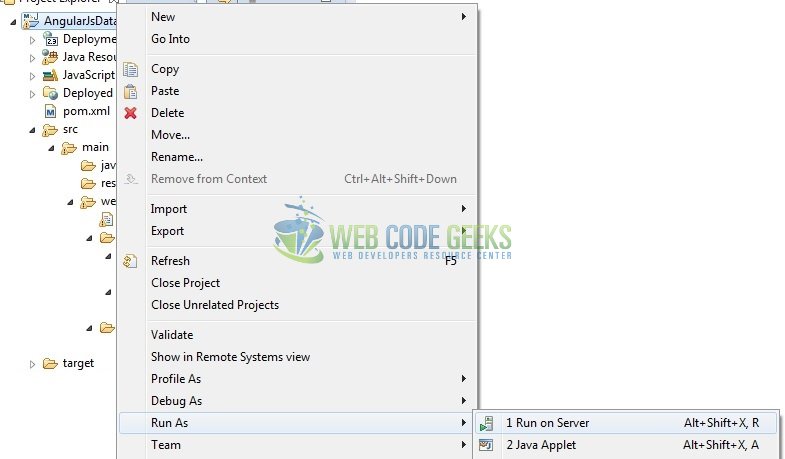
Tomcat will deploy the application in its web-apps folder and shall start its execution to deploy the project so that we can go ahead and test it in the browser.
5. Project Demo
Open your favorite browser and hit the following URL. The output page having the default values will be displayed.
http://localhost:8082/AngularJsDatabinding/
Server name (localhost) and port (8082) may vary as per your Tomcat configuration.
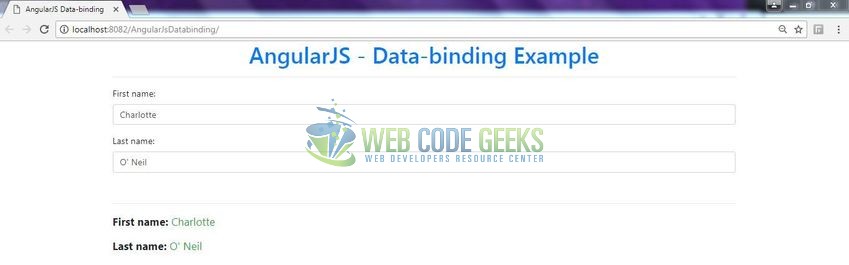
Here when a user changes the text-box values, the placeholder text will be changed in real-time.That’s all for this post. Happy Learning!!
6. Conclusion
In this section, developers learned how to create a simple two-way data binding application using the angular library. Developers can download the sample application as an Eclipse project in the Downloads section.
7. Download the Eclipse Project
This was an example of data binding in the angular library.
You can download the full source code of this example here: AngularJsDatabinding