AngularJS Events Tutorial
Hello readers, in this tutorial, we will understand how the event directives work in the angular library.
1. Introduction
Before we begin with the tutorial, let’s take a look and at the Angular JavaScript Events in angular and its characteristics.
1.1 Angular JavaScript
Angular is a JavaScript MVC or Model-View-Controller framework developed by Google that lets developers build well structured, easily testable, and maintainable front-end applications. But before we start creating a real application using the angular library, let us see what the important parts of an angular application are.
Table Of Contents
1.1.1 Templates
In Angular, a template is an HTML
with added markups. The angular library compiles the templates and renders the resultant HTML
page.
1.1.2 Directives
Directives are the markers (i.e. attributes) on a DOM element that tell angular to attach a specific behavior to that DOM element or even transform the DOM element and its children. Most of the directives in the angular library start with the ng
. The directives consist of the following parts:
ng-app
: Theng-app
directive is a starting point. If the angular framework finds theng-app
directive anywhere in theHTML
document, it bootstraps (i.e. initializes) itself and compiles theHTML
templateng-model
: Theng-model
directive binds anHTML
element to a property on the$scope
object. It also binds the values of angular application data to theHTML
input controlsng-bind
: Theng-bind
directive binds the angular application data to theHTML
tagsng-controller
: Theng-controller
directive specifies a controller in theHTML
element. This controller will add behavior or support the data in thatHTML
element and its child elements
1.1.3 Expressions
An expression is like a JavaScript code usually wrapped inside the double curly braces such as {{ expression }}
. Angular library evaluates the expression and produces a result.
1.2 Events in Angular
While creating the advanced angular web-applications, sooner or later developers will need to handle the HTML
events like mouse clicks, keyboard presses, change events etc. An angular library provides a simple model to add the event listeners to the HTML
components for generating the front-end views. The below list highlights the common event listeners that developers can attach to the HTML
elements.
1.2.1 ‘ng-click’ Event
The ng-click
angular directive evaluates the angular expression when an HTML
element is clicked. This angular directive will not override the element’s original onclick
event i.e. both operations will be executed. Here is the prototype form:
Syntax
<button id="singleClick" ng-click="clickme = clickme + 1" ng-init="clickme=0">Click me</button>
1.2.2 ‘ng-dblclick’ Event
The ng-dblclick
angular directive evaluates the angular expression when an HTML
element is double-clicked. This angular directive will not override the element’s original ondblclick
event i.e. both operations will be executed. Here is the prototype form:
Syntax
<button id="doubleClick" ng-dblclick="dblclickme = dblclickme + 1" ng-init="dblclickme=0">Double click me</button>
1.2.3 ‘ng-mousedown’ Event
The ng-mousedown
angular directive evaluates the angular expression when a mouse button is clicked on an HTML
element. This angular directive will not override the element’s original onmousedown
event i.e. both operations will be executed. Here is the prototype form:
Syntax
<div id="mouseDown" ng-mousedown="count = count + 1" ng-init="count=0">Click me</div>
1.2.4 ‘ng-mouseup’ Event
The ng-mouseup
angular directive evaluates the angular expression when a mouse click is finished. This angular directive will not override the element’s original onmouseup
event i.e. both operations will be executed. Here is the prototype form:
Syntax
<div id="mouseUp" ng-mouseup="count = count + 1" ng-init="count=0">Click me</div>
1.2.5 ‘ng-mouseenter’ Event
The ng-mouseenter
angular directive evaluates the angular expression when a mouse cursor enters the specific HTML
element. This angular directive will not override the element’s original onmouseenter
event i.e. both operations will be executed. Here is the prototype form:
Syntax
<div id="mouseEnter" ng-mouseenter="count = count + 1" ng-init="count=0">Mouse over me</div>
1.2.6 ‘ng-mouseleave’ Event
The ng-mouseleave
angular directive evaluates the angular expression when a mouse cursor leaves the specific HTML
element. This angular directive will not override the element’s original onmouseleave
event i.e. both operations will be executed. Here is the prototype form:
Syntax
<div id="mouseLeave" ng-mouseleave="count = count + 1" ng-init="count=0">Mouse over me and Mouse away from me</div>
1.2.7 ‘ng-mousemove’ Event
The ng-mousemove
angular directive evaluates the angular expression when a mouse cursor moves over the specific HTML
element. This angular directive will not override the element’s original onmousemove
event i.e. both operations will be executed. Here is the prototype form:
Syntax
<div id="mouseMove" ng-mousemove="count = count + 1" ng-init="count=0">Mouse over me</div>
Do note, this directive has a similar functionality to that of the ng-mouseover
event.
1.2.8 ‘ng-change’ Event
The ng-change
angular directive evaluates the angular expression when a value of an HTML
element changes i.e. this event is triggered at every change in a value and will not wait until all the changes are made or when the input text-field loses the focus. This directive has a pre-requirement of the ng-model
angular directive. Here is the prototype form:
Syntax
<div ng-app="app" ng-controller="ctrl"> <input type="text" ng-change="func()" ng-model="value" /> <p>The input field has changed {{ count }} times.</p> </div> <script> angular.module('app', []).controller('ctrl', ['$scope', function($scope) { $scope.count = 0; $scope.func = function() { $scope.count++; }; }]); </script>
1.2.9 ‘ng-keydown’ Event
The ng-keydown
angular directive evaluates the angular expression when a keyboard is used on the specific HTML
element. This angular directive will not override the element’s original onkeydown
event i.e. both operations will be executed. Here is the prototype form:
Syntax
<input ng-keydown="count = count + 1" ng-init="count=0" />
Do note, this directive has a similar functionality to that of the ng-keyup
and ng-keypress
events.
These new APIs make developer’s life easier, really! But it would be difficult for a beginner to understand this without an example. Therefore, let us create a simple example to implement the event listeners in the angular library.
2. AngularJS Events Tutorial
Here is a step-by-step guide for implementing the event listeners in the angular web applications.
2.1 Tools Used
We are using Eclipse Kepler SR2, JDK 8 and Maven. Having said that, we have tested the code against JDK 1.7 and it works well.
2.2 Project Structure
Firstly, let’s review the final project structure if you are confused about where you should create the corresponding files or folder later!
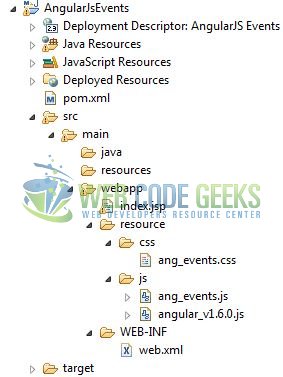
2.3 Project Creation
This section will show how to create a Java-based Maven project with Eclipse. In Eclipse Ide, go to File -> New -> Maven Project
.
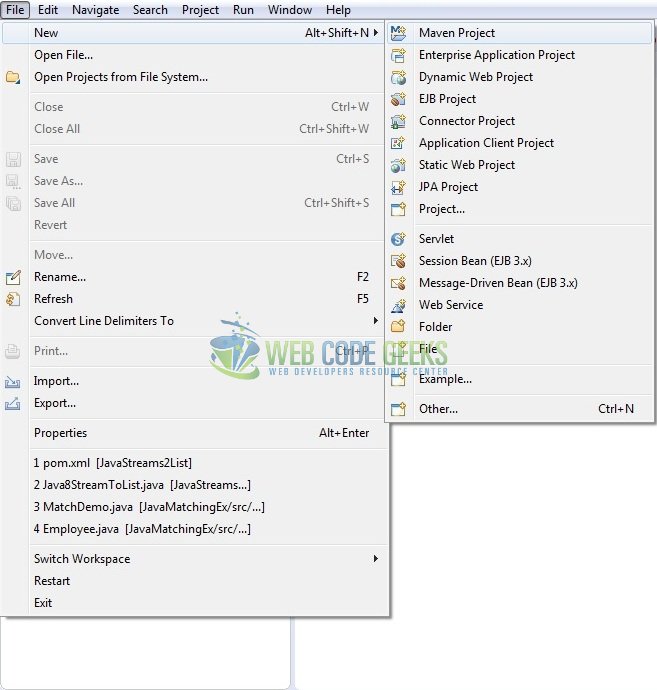
In the New Maven Project window, it will ask you to select project location. By default, ‘Use default workspace location’ will be selected. Just click on next button to proceed.
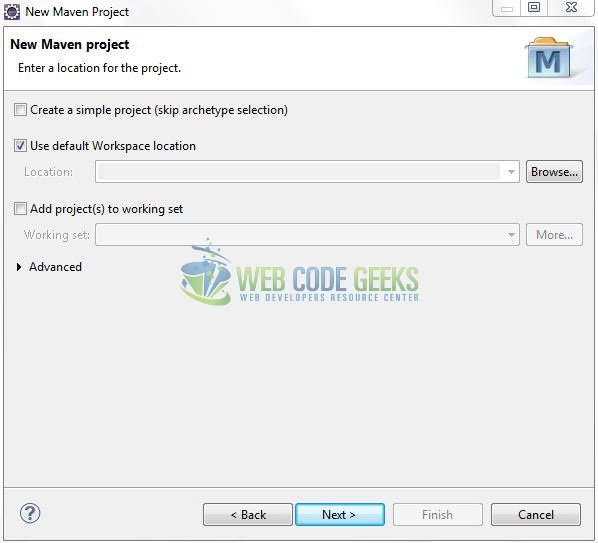
Select the ‘Maven Web App’ Archetype from the list of options and click next.
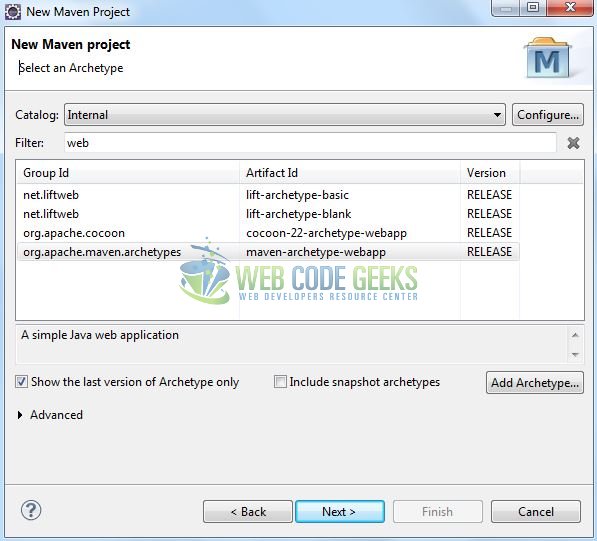
It will ask you to ‘Enter the group and the artifact id for the project’. We will enter the details as shown in the below image. The version number will be by default: 0.0.1-SNAPSHOT
.
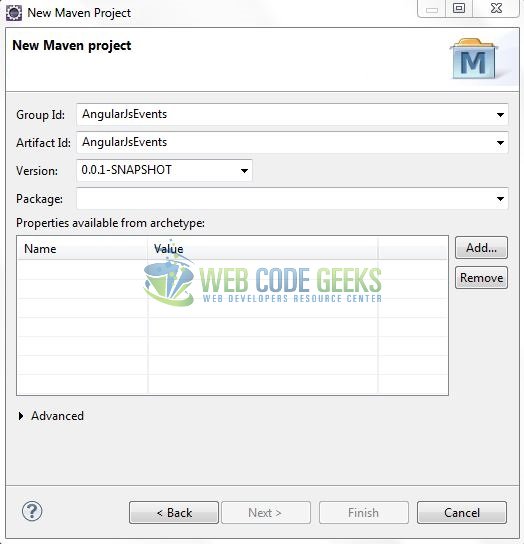
Click on Finish and the creation of a maven project is completed. If you see, it has downloaded the maven dependencies and a pom.xml
file will be created. It will have the following code:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>AngularJsEvents</groupId> <artifactId>AngularJsEvents</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> </project>
3. Application Building
Let’s start building an application to understand the basic building blocks of the event listeners in the angular library.
3.1 Load the Angular framework
Since it is a pure JavaScript framework, we should add its reference using the <script>
tag.
<script type="text/javascript" src="resource/js/angular_v1.6.0.js"></script>
3.2 Define the Angular application
Next, we will define the angular application using the <ng-app>
and <ng-controller>
directives.
index.jsp
<div ng-app="myApp" ng-controller="CalCtrl"> ... </div>
3.3 Create the Angular controller
The ang_events.js
javascript file initializes the car’s list and binds the list to the ng-repeat
tag. This directive will then generate the car’s list in the HTML
row element. The important point to note is that we have introduced a special variable named $event
which developers can use as an input argument to the event listener function.
ang_events.js
var mainApp = angular.module("myApp", []); mainApp.controller("myCtrl", [ '$scope', function($scope) { $scope.sortType = 'name'; // Set the default sort type $scope.sortOrder = false; // Set the default sort order // Create the list of car types $scope.cars = [ { name: "Mercedes-Benz", likes:0, dislikes:0 }, { name: "Ferrari", likes: 0, dislikes: 0 }, { name: "Audi", likes: 0, dislikes: 0 }, { name: "Volvo", likes: 0, dislikes: 0 }, { name: "BMW", likes: 0, dislikes: 0 }, { name: "Lamborghini", likes: 0, dislikes: 0 }]; $scope.incrementLikes = function (car) { car.likes++; }; $scope.incrementDislikes = function (car) { car.dislikes++; }; $scope.ownerReview = function ($event) { $scope.remark = $event.target; }; } ]);
3.4 Complete Application
Complete the above steps to show the front view and understand the use of event listeners in an angular web application.
index.jsp
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>AngularJS Events</title> <!-- Angular Javascript File --> <script type="text/javascript" src="resource/js/angular_v1.6.0.js"></script> <script type="text/javascript" src="resource/js/ang_events.js"></script> <!-- Bootstrap Css --> <link rel="stylesheet" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/4.0.0/css/bootstrap.min.css"> <link rel="stylesheet" href="resource/css/ang_events.css"> </head> <body> <div id="angularEvents" class="container"> <h1 align="center" class="text-primary">AngularJS - Events Example</h1> <hr /> <!------ ANGULAR JS EVENTS EXAMPLE ------> <div ng-app="myApp" ng-controller="myCtrl"> <table class="table"> <thead> <tr> <th scope="col"><a href="#" ng-click="sortType = 'name'; sortOrder = !sortOrder">Name</a></th><th scope="col">Likes</th><th scope="col">Dislikes</th><th scope="col">Like or Dislike?</th><th scope="col"></th><th scope="col">Comments</th> </tr> </thead> <tbody> <tr ng-repeat="car in cars | orderBy:sortType:sortOrder" ng-class="rollClass" ng-mouseenter="rollClass = 'highlight'" ng-mouseleave="rollClass = ''"> <td>{{ car.name }}</td> <td>{{ car.likes }}</td> <td>{{ car.dislikes }}</td> <td> <span id="likeBtnSpan"> <button id="likeBtn" type="submit" class="btn btn-outline-success" ng-click="incrementLikes(car)">Like</button> </span> <span id="btnSpace" class="space"></span> <span id="disLikeBtnSpan"> <button id="dislikeBtn" type="submit" class="btn btn-outline-warning" ng-click="incrementDislikes(car)">Dislike</button> </span> </td> <td> <input id="remark" name="remark" type="text" class="form-control form-control-sm" placeholder="Enter comments ..." ng-model="remark" ng-change="ownerReview(this)" /> </td> <td>{{ remark }}</td> </tr> </tbody> </table> </div> </div> </body> </html>
4. Run the Application
As we are ready for all the changes, let us compile the project and deploy the application on the tomcat server. To deploy the application on Tomat7, right-click on the project and navigate to Run as -> Run on Server
.
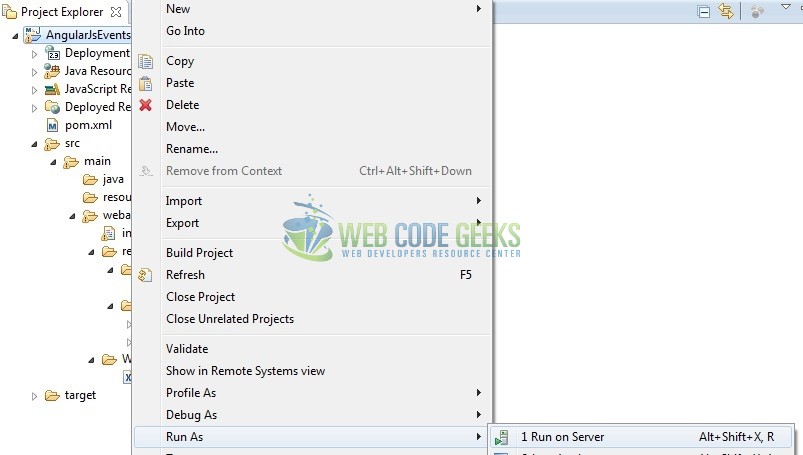
Tomcat will deploy the application in its web-apps folder and shall start its execution to deploy the project so that we can go ahead and test it in the browser.
5. Project Demo
Open your favorite browser and hit the following URL. The output page will be displayed.
http://localhost:8082/AngularJsEvents/
Server name (localhost) and port (8082) may vary as per your Tomcat configuration.
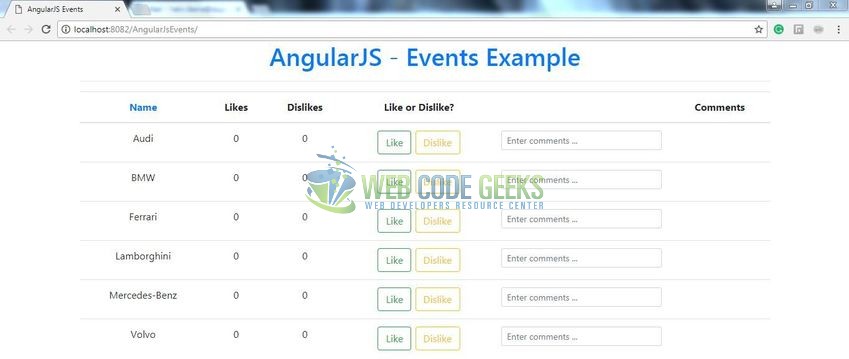
Users can click the buttons and write data in the input text-box to understand the behavior of different event listeners in the angular library. The application will generate the following output as shown below.
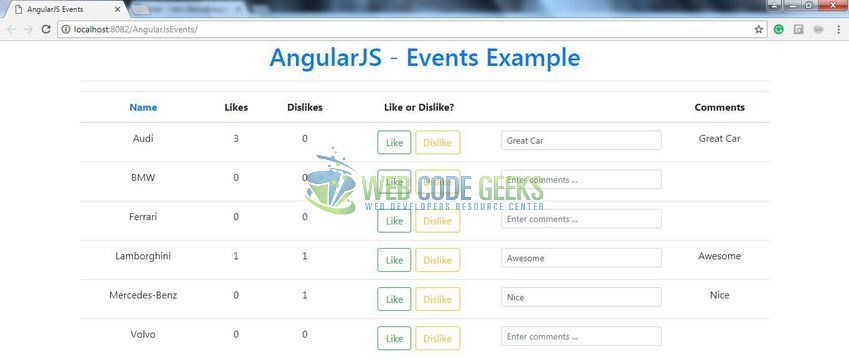
That’s all for this post. Happy Learning!!
6. Conclusion
In this section, developers learned about the concept of using event listeners in the angular library. Developers can download the sample application as an Eclipse project in the Downloads section.
7. Download the Eclipse Project
This was a tutorial of events in the angular library.
You can download the full source code of this example here: AngularJsEvents