AngularJS Get Current Date Time Example
Hello readers, in this tutorial, developers will learn what AngularJS is and how to display the current date and time using the angular filter.
1. Introduction
AngularJS is a JavaScript MVC or Model-View-Controller framework developed by Google that lets developers build well structured, easily testable, and maintainable front-end applications. Before we start with the creation of a real application using the angular framework, let us see the important parts of an angular application.
1.1.1 Templates
In angular, a template is an HTML
with added markups. AngularJS library compiles the templates and renders the resultant HTML
page.
1.1.2 Directives
Directives are the markers (i.e. attributes) on a DOM element that tell angular to attach a specific behavior to that DOM element or even transform the DOM element and its children. Most of the directives in the angular library start with the prefix ng
. Below is the list of important directives that are available in the angular library.
ng-app
: Theng-app
directive is a starting point. If the AngularJS framework finds theng-app
directive anywhere in theHTML
document, it bootstraps (i.e. initializes) itself and compiles theHTML
templateng-model
: Theng-model
directive binds anHTML
element to a property on the$scope
object. It also binds the values of AngularJS application data to theHTML
input controls.ng-bind
: Theng-bind
directive binds the AngularJS application data to theHTML
tagsng-controller
: Theng-controller
directive specifies a controller in theHTML
element. This controller will add behavior or maintain the data in thatHTML
element and its child elementsng-repeat
: Theng-repeat
directive repeat a set ofHTML
elements for each item in a given collection
1.1.3 Expressions
An expression is like a JavaScript code usually wrapped inside the double curly braces such as {{ expression }}
. AngularJS library evaluates the expression and produces a result.
These new APIs make developer’s life easier, really! But it would be difficult for a beginner to understand this without an example. Therefore, let us create a simple application using the date filter in the angular applications.
2. AngularJS Get Current Date Time Example
Here is a step-by-step guide for implementing this tutorial in the angular applications.
2.1 Tools Used
We are using Eclipse Kepler SR2, JDK 8 and Maven. Having said that, we have tested the code against JDK 1.7 and it works well.
2.2 Project Structure
Firstly, let’s review the final project structure if you are confused about where you should create the corresponding files or folder later!
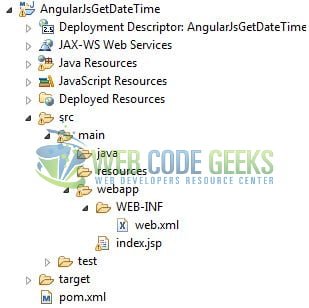
2.3 Project Creation
This section will show how to create a Java-based Maven project with Eclipse. In Eclipse Ide, go to File -> New -> Maven Project
.
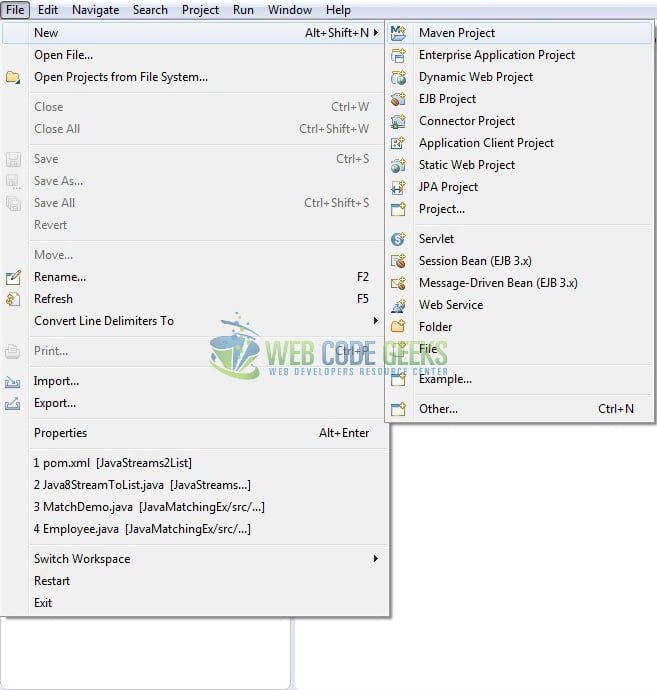
In the New Maven Project window, it will ask you to select project location. By default, ‘Use default workspace location’ will be selected. Just click on next button to proceed.
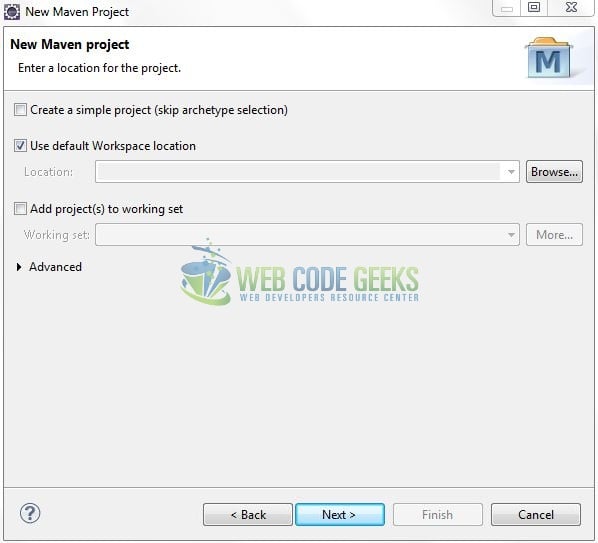
Select the ‘Maven Web App’ Archetype from the list of options and click next.
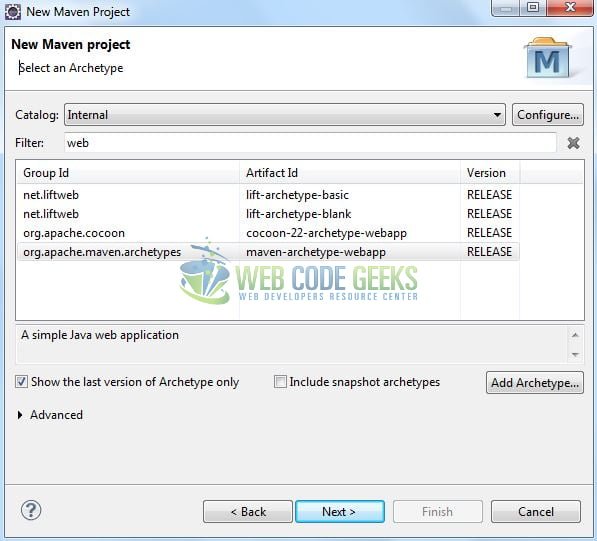
It will ask you to ‘Enter the group and the artifact id for the project’. We will enter the details as shown in the below image. The version number will be by default: 0.0.1-SNAPSHOT
.
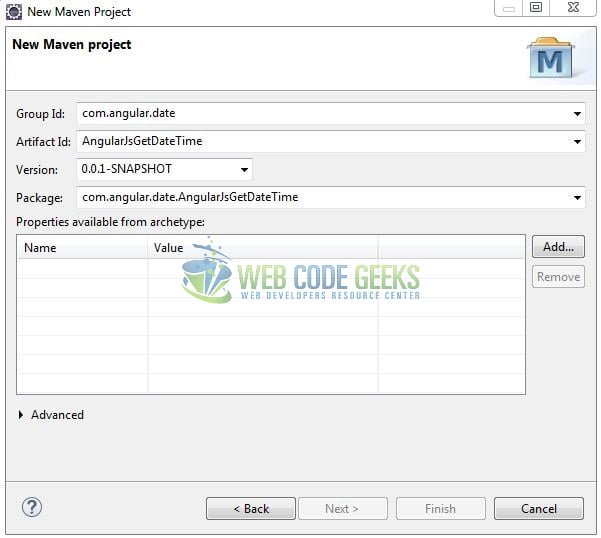
Click on Finish and the creation of a maven project is completed. If you see, it has downloaded the maven dependencies and a pom.xml
file will be created. It will have the following code:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.angular.date</groupId> <artifactId>AngularJsGetDateTime</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> </project>
Let’s start building the application!
3. Application Building
Let’s create an application to understand the basic building blocks of this tutorial.
3.1 Load the AngularJS framework
Since it is a pure JavaScript framework, we should add its reference using the <script>
tag.
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
3.2 Define the AngularJS application
Next, we will define the AngularJS application using the <ng-app>
and <ng-controller>
directives.
index.jsp
<div ng-app="app" ng-controller="dateCtrl"> ... </div>
3.3 Complete Application
In angular, the date
filter converts a date to a string based on the given format. AngularJS allow the conversation of data to multiple formats using this filter. Following is the syntax of using the date filter in angular applications.
Syntax
{{ date_expression | date : format }}
Developers can refer to this link for the different type of date formats available with this filter. Complete the above steps and I will show you how to use the ‘date
‘ filter with real-life practices. Let’s see the code snippet.
index.jsp
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>AngularJS Date/Time</title> <!-- AngularJs File --> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script> <!-- Bootstrap Css --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.2/css/bootstrap.min.css"> <script type="text/javascript"> var dateApp = angular.module('app', []); dateApp.controller('dateCtrl', ['$scope', '$filter', '$interval', function ($scope, $filter, $interval) { var date = new Date(); var date_format = 'EEEE MMMM d, y hh:mm:ss a'; var tick = function() { $scope.get_current_date_time = $filter('date')(new Date(), date_format); } tick(); $interval(tick, 1000); }]); </script> </head> <body> <div id="angularGetDateTime" class="container"> <h1 align="center" class="text-primary">AngularJS - Get Date/Time Example</h1> <hr /> <div ng-app="app" ng-controller="dateCtrl"> <h3 id="header_msg" class="text-warning">A demo to display the current <em>date & time</em> in AngularJS!</h3> <div> </div> <h5 class="text-black-50">Date = {{ get_current_date_time }}</h5> </div> </div> </body> </html>
4. Run the Application
As we are ready for all the changes, let us compile the project and deploy the application on the Tomcat7 server. To deploy the application on Tomat7, right-click on the project and navigate to Run as -> Run on Server
.
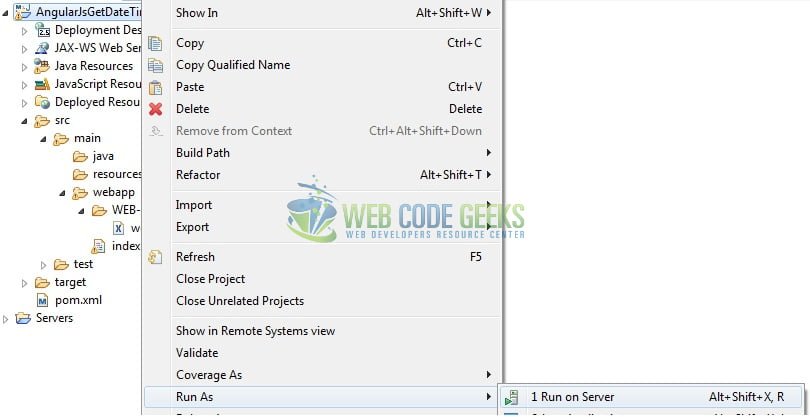
Tomcat will deploy the application in its web-apps folder and shall start its execution to deploy the project so that we can go ahead and test it in the browser.
5. Project Demo
Open your favorite browser and hit the following URL. The output page using the date filter will be displayed.
http://localhost:8082/AngularJsGetDateTime/
Server name (localhost) and port (8082) may vary as per your Tomcat configuration.

That’s all for this post. Happy Learning!!
6. Conclusion
In this section, developers learned how to create a simple application using the date
angular filter. Developers can download the sample application as an Eclipse project in the Downloads section.
7. Download the Eclipse Project
This was an example of the AngularJS Get Current Date Time of the AngularJS framework.
You can download the full source code of this example here: AngularJsGetDateTime