AngularJS Scope
1. Overview
In this post, we are reviewing scope in AngularJS. It is passed as an argument when we make a controller:
<script> var myApp = angular.module('myApp', []); myApp.controller('myController', function($scope) { $scope.name = "Red Dead Redemption"; }); </script>
Scope is actually an object that refers to the model in an application structure, such as Model View Controller (MVC) .
It provides definitions – also known as context – for JavaScript-like code snippets called expressions. Scopes are structured in a hierarchy that mimics the Document Object Model (DOM) structure of the application. Scopes can watch expressions and propagate events in a similar way to DOM events.
2. Scope is a Data Model
What does it mean for Scope to be a data model? It is a JavaScript object with properties and methods that can be accessed by both the view and controller:
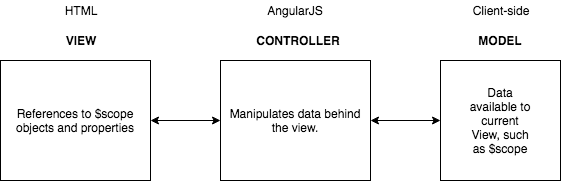
Here, we have an example that demonstrates how modifying the view can affect the controller and model:
<!DOCTYPE html> <html> <script src = "https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9hamF4Lmdvb2dsZWFwaXMuY29tL2FqYXgvlibs/angularjs/1.7.5/angular.min.js"></script> <body> <div ng-app="demoApp" ng-controller="demoCtrl"> <input ng-model="word"> <h1>Hello, {{word}}!</h1> </div> <script> var app = angular.module('demoApp', []); app.controller('demoCtrl', function($scope) { $scope.word = "word"; }); </script> <p>If we change the word in the input field, the change will affect the model and the word property in the controller.</p> </body> </html>
Feel free to copy and paste that code into your favorite text editor or download the file from my Github.
In this simple example, if we modify the input in the web page, we see the value change for the greeting in the <h1> tags:
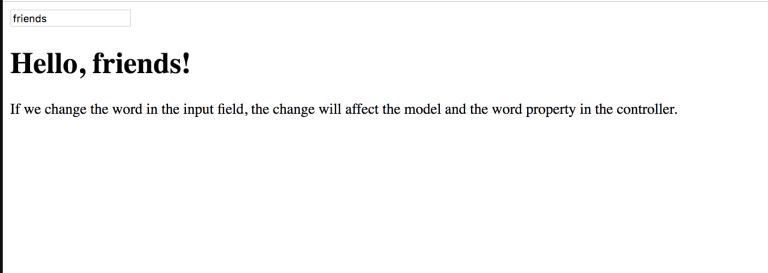
3. Root Scope & Hierarchies
Each AngularJS application has only one root scope, but can have any number of child scopes.
An application can have several scopes because directives can create new child scopes. When new scopes are created, they become children of their parent scope. This creates a tree structure which parallels the DOM where they’re attached.
The example below, which is available in my Github, shows how multiple scopes work in an application and also prototypal inheritance of properties:
<script src = "https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9hamF4Lmdvb2dsZWFwaXMuY29tL2FqYXgvlibs/angularjs/1.7.5/angular.min.js"></script> <script> (function(angular) { 'use strict'; angular.module('scopeExample', []) .controller('HelloController', ['$scope', '$rootScope', function($scope, $rootScope) { $scope.name = 'World'; $rootScope.department = 'Red Dead Redemption 2'; }]) .controller('ListController', ['$scope', function($scope) { $scope.names = ['Arthur', 'Dutch', 'Bill']; }]); })(window.angular); </script> </head> <body ng-app="scopeExample"> <div class="show-scope-demo"> <div ng-controller="HelloController"> Hello {{name}}! </div> <div ng-controller="ListController"> <ol> <li ng-repeat="name in names">{{name}} from {{department}}</li> </ol> </div> </div>
The page will display like this:
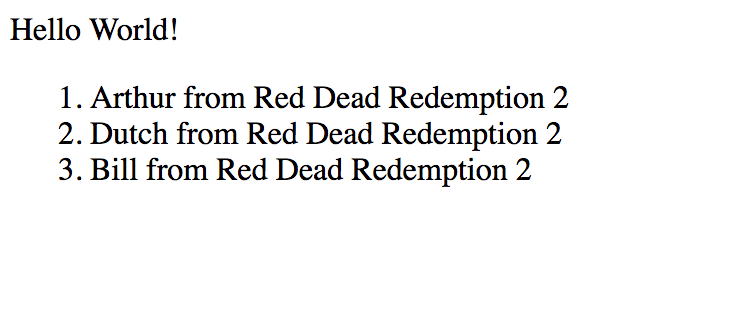
In the code shown above, when [[name]] is evaluated by AngularJS, it first looks at the scope associated with the value for the ng-controller attribute for the name property. This is why it says “Hello World!” on top rather than a character name from Red Dead Redemption 2.
If the property is not found, it searches the parent scope and so on until the root scope is reached. The AngularJS documentation calls this prototypical inheritance, while others like Mozilla call it prototypal inheritance.
Regardless of what it’s truly called, what we need to know is JavaScript only has one construct: objects. Every object has a private property that holds a link to another object called its prototype.
4. Conclusion
Today we reviewed the core concepts of scope in AngularJS. We reviewed that it is an object that resides in the model of an application; that it is hierarchical and has prototypal inheritance; and, there can only be one root scope.
AngularJS can be used effectively with the Spring Framework. To learn more AngularJS, I will be checking out courses on Udemy and Treehouse.
Published on Web Code Geeks with permission by Michael Good, partner at our WCG program. See the original article here: AngularJS Scope Opinions expressed by Web Code Geeks contributors are their own. |