jQuery Event Methods Example
Greetings readers, in this tutorial we will show how to use the jQuery library and the different event methods to perform an operation in an HTML page using jQuery.
1. Introduction
jQuery is nothing but a JavaScript library that comes with rich functionalities. It is small and faster than many JavaScript code written by an average web developer. By using jQuery developers can write less code and do more things, making web developer’s tasks very easy. In simple word, jQuery is a collection of several useful methods, which can be used to carry out many common tasks in JavaScript. A couple of lines of jQuery code can do things which need too many JavaScript lines to do. The true power of jQuery comes from its CSS-like selector, which allows it to select any element from DOM and change, update or manipulate it. Developers can use jQuery to do cool animations like fade in or fade out. They can also change the CSS class of a component dynamically e.g. making a component active or inactive. I have used this technique to make tabbed UI in HTML. I can vouch for jQuery that once developers start using it, they will never go back to plain old JavaScript as jQuery is clear, concise, and powerful.
Table Of Contents
1.1 Why jQuery?
- It helps in improving the application performance and increasing the developer’s productivity
- It helps in developing the browser compatible web-page
- It helps in implementing the UI related critical functionality without writing the several lines of codes
- It is fast and extensible i.e. developers can use the jQuery library to implement the customized behavior
- It is simpler and easy to learn
1.2 jQuery Event Methods
The jQuery library provides several event methods that trigger or fire an event or an operation in an HTML page. For example:
- Clicking an element
- Moving the move over an element
- Selecting the checkbox etc.
The following table lists the common jQuery event.
Mouse Events | Keyboard Events | Form Events | Document/Window Events |
---|---|---|---|
click | keypress | submit | load |
dblclick | keydown | change | resize |
mouseenter | keyup | focus | scroll |
mouseleave | blur | unload | |
select |
1.3 Commonly Used jQuery Event Methods
In jQuery, DOM elements have an equivalent jQuery method. Let us take a brief look at the most commonly used event methods in the jQuery library.
1.3.1 Click event
The jQuery click event or the click()
method attaches an event handler to an HTML element. This function executes when a mouse is clicked. Over here represent the simple syntax to practice this method.
Snippet
// A click event is attached to the selected HTML element. $(selector).click(); // or; A click event is attached to a function. $(selector).click(function);
1.3.2 Double-click event
The jQuery double-click event or the dblclick()
method attaches an event handler to an HTML element. This function executes when a mouse is double-clicked. Over here represent the simple syntax to practice this method.
Snippet
// A double-click event is attached to the selected HTML element. $(selector).dblclick(); // or; A double-click event is attached to a function. $(selector).dblclick(function);
1.3.3 Mouse Enter event
The jQuery mouse enter event or the mouseenter()
method attaches an event handler to an HTML element. This function executes when a mouse pointer enters the HTML element region. Over here represent the simple syntax to practice this method.
Snippet
// A mouseenter event is attached to the selected HTML element. $(selector).mouseenter(); // or; A mouseenter event is attached to a function. $(selector).mouseenter(function);
1.3.4 Mouse Leave event
The jQuery mouse leave event or the mouseleave()
method attaches an event handler to an HTML element. This function executes when a mouse pointer leaves the HTML element region. Over here represent the simple syntax to practice this method.
Snippet
// A mouseleave event is attached to the selected HTML element. $(selector).mouseleave(); // or; A mouseleave event is attached to a function. $(selector).mouseleave(function);
1.3.5 Mouse Down event
The jQuery mouse down event or the mousedown()
method attaches an event handler to an HTML element. This function executes when the left or right buttons of a mouse is pressed, while the mouse is over the HTML element. Over here represent the simple syntax to practice this method.
Snippet
// A mousedown event is attached to the selected HTML element. $(selector).mousedown(); // or; A mousedown event is attached to a function. $(selector).mousedown(function);
1.3.6 Mouse Up event
The jQuery mouse up event or the mouseup()
method attaches an event handler to an HTML element. This function executes when the left or right buttons of a mouse is released, while the mouse is over the HTML element. Over here represent the simple syntax to practice this method.
Snippet
// A mouseup event is attached to the selected HTML element. $(selector).mouseup(); // or; A mouseup event is attached to a function. $(selector).mouseup(function);
1.3.7 Focus event
The jQuery focus event or the focus()
method attaches an event handler to an HTML form field. This function executes when the form field gets focus. Over here represent the simple syntax to practice this method.
Snippet
// A focus event is attached to the selected HTML element. $(selector).focus(); // or; A focus event is attached to a function. $(selector).focus(function);
1.3.8 Blur event
The jQuery blur event or the blur()
method attaches an event handler to an HTML form field. This function executes when the form field loses focus. Over here represent the simple syntax to practice this method.
Snippet
// A blur event is attached to the selected HTML element. $(selector).blur(); // or; A blur event is attached to a function. $(selector).blur(function);
1.3.9 On event
The jQuery on event or the on()
method attaches one or more event handlers for the selected HTML elements. Over here represent the simple syntax to practice this method.
Snippet
$(selector).on(event, childSelector, data, function, map);
Where,
event
is a required parameter that specifies one or more event(s)childSelector
is an optional parameter that specifies that the event handler should only be attached to the specified child elementsdata
is an optional parameter that specifies the additional data to pass along to the functionfunction
is a required parameter that specifies the function to be executed when the event occursmap
specifies the event map
These new APIs make a developer life easier, really! But it would be difficult for a beginner to understand this without an example. Therefore, let us create a simple application using the jQuery library.
2. jQuery Event Methods Example
Here is a systematic guide for implementing this tutorial using the jQuery library.
2.1 Tools Used
We are using Eclipse Kepler SR2, JDK 8 and Maven. Having said that, we have tested the code against JDK 1.7 and it works well.
2.2 Project Structure
Firstly, let us review the final project structure if you are confused about where you should create the corresponding files or folder later!
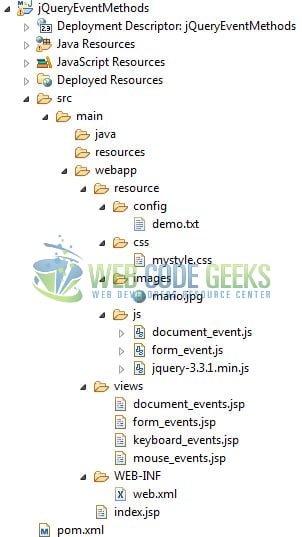
2.3 Project Creation
This section will show on how to create a Java-based Maven project with Eclipse. In Eclipse Ide, go to File -> New -> Maven Project
.
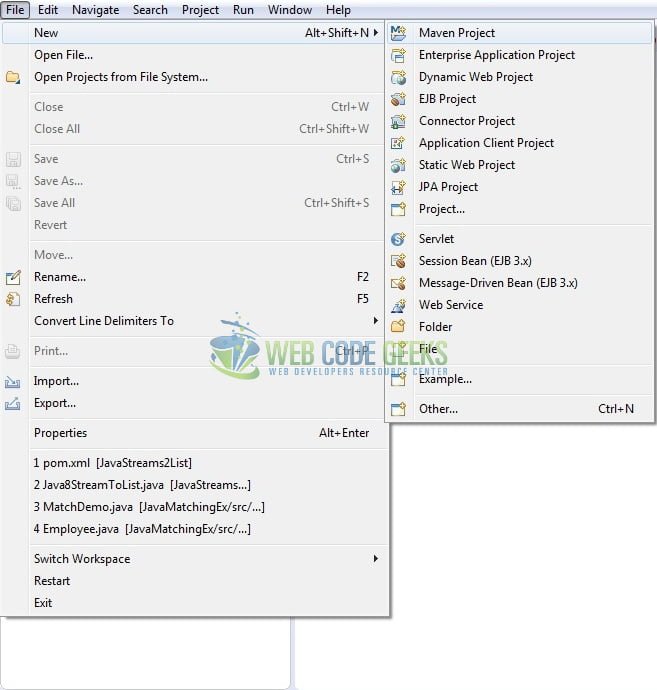
In the New Maven Project window, it will ask you to select project location. By default, ‘Use default workspace location’ will be selected. Just click on next button to proceed.
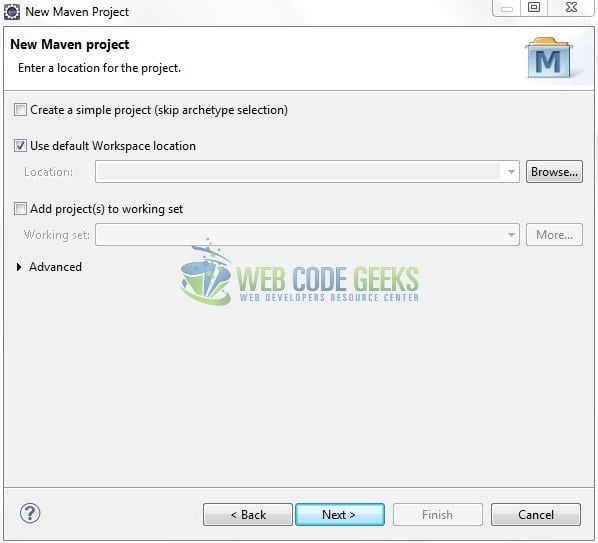
Select the ‘Maven Web App’ Archetype from the list of options and click next.
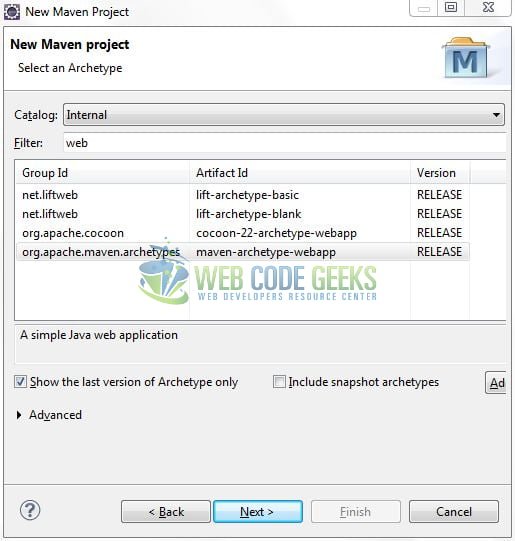
It will ask you to ‘Enter the group and the artifact id for the project’. We will input the details as shown in the below image. The version number will be by default: 0.0.1-SNAPSHOT
.
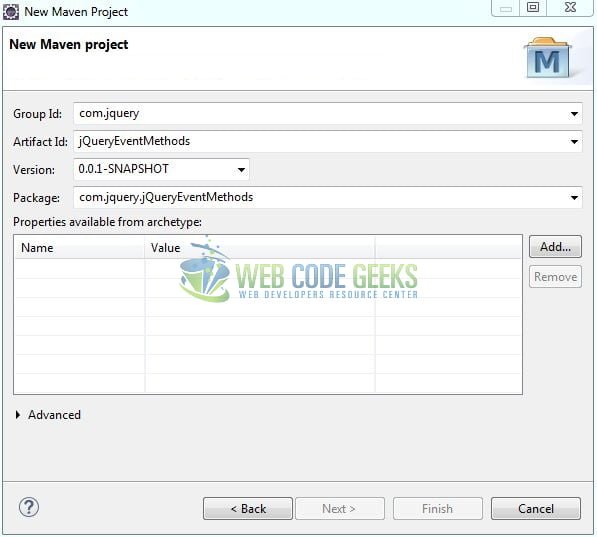
Click on Finish and the creation of a maven project is completed. If you see, it has downloaded the maven dependencies and a pom.xml
file will be created. It will have the following code:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.jquery</groupId> <artifactId>jQueryEventMethods</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> </project>
3. Application Building
Let us create an application to understand the basic building blocks of this tutorial.
3.1 Load the jQuery Library
Since it is a pure JavaScript framework, so developers should add its reference using the <script>
tag.
<script type="text/javascript" src="resource/js/jquery-3.3.1.min.js"></script>
Developers can either download the jQuery from the official website and the application will then directly load the jQuery library from the local drive or they can also include the direct CDN link under the script
tag.
3.2 Define the Application
3.2.1 Index Page
Let us write a simple index page in the jQueryEventMethods/src/main/webapp/
folder. Add the following code to it:
index.jsp
<!DOCTYPE html> <html lang="en"> <head> <title>Index Page</title> <meta charset="UTF-8"> <!-- ==== Css files ==== --> <link rel="stylesheet" type="text/css" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/4.1.3/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="resource/css/mystyle.css"> <!-- ==== JavaScript files ==== --> <script src="resource/js/jquery-3.3.1.min.js"></script> </head> <body> <div class="container"> <h2 class="text-primary text-align">jQuery Event Methods</h2><hr /> <div> </div> <!-- ==== jQuery Event Methods Example ==== --> <div id="start_message"> <h4 class="text-info text-align">This demo is for the different event methods in jQuery</h4> </div> <div> </div> <div class="three-inline-btns"> <div class="inner"> <a class="btn btn-primary" href="views/document_events.jsp" role="button">Document Events</a> </div> <div class="inner"> <a class="btn btn-primary" href="views/form_events.jsp" role="button">Form Events</a> </div> <div class="inner"> <a class="btn btn-primary" href="views/keyboard_events.jsp" role="button">Keyboard Events</a> </div> <div class="inner"> <a class="btn btn-primary" href="views/mouse_events.jsp" role="button">Mouse Events</a> </div> </div> </div> </body> </html>
3.2.2 Document Events Page
Let us write a simple document events page in the jQueryEventMethods/src/main/webapp/views/
folder. Add the following code to it:
document_events.jsp
<!DOCTYPE html> <html lang="en"> <head> <title>Document Events</title> <meta charset="UTF-8"> <!-- ==== Css files ==== --> <link rel="stylesheet" type="text/css" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/4.1.3/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="../resource/css/mystyle.css"> <!-- ==== JavaScript files ==== --> <script src="../resource/js/jquery-3.3.1.min.js"></script> <script src="../resource/js/document_event.js"></script> </head> <body> <div class="container"> <h2 class="text-primary text-align">jQuery Document Events Example</h2><hr /> <div> </div> <!-- ==== jQuery Document Events Methods Example ==== ---> <div id="image"> <img id="myImage" src="../resource/images/mario.jpg" alt="Mario" height="125" /> </div> <div> </div> <span id="result"></span> <div> </div> <button id="driver" type="submit" class="btn btn-default">Load</button> <div id="stage"></div> </div> </body> </html>
3.2.2.1 Define the jQuery function
Add the following code to the document_event.js
file.
document_event.js
$(document).ready(function() { $(document).ready(function() { // load() $("#driver").click(function(event){ $("#stage").load('../resource/config/demo.txt'); }); /* // scroll() $("#myImage").scroll(function() { var message = "scrolled<div />"; $("#result").append(message); }); */ }); //resize() $(window).resize(function() { var message = "resize(): Height= " + $(this).height() + ", Width= " + $(this).width(); $("#result").text(message); })
3.2.3 Form Events Page
Let us write a simple form events page in the jQueryEventMethods/src/main/webapp/views/
folder. Add the following code to it:
form_events.jsp
<!DOCTYPE html> <html lang="en"> <head> <title>Form Events</title> <meta charset="UTF-8"> <!-- ==== Css files ==== --> <link rel="stylesheet" type="text/css" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/4.1.3/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="../resource/css/mystyle.css"> <!-- ==== JavaScript files ==== --> <script src="../resource/js/jquery-3.3.1.min.js"></script> <script src="../resource/js/form_event.js"></script> </head> <body> <div class="container"> <h2 class="text-primary text-align">jQuery Form Events Example</h2><hr /> <div> </div> <!-- ==== jQuery Form Events Example ==== ---> <div class="form-row"> <div class="col"> <input id="blur" type="text" class="form-control" placeholder="Blur Event" /> </div> <div class="col"> <input id="change" type="text" class="form-control" placeholder="Change Event" /> </div> <div class="col"> <input id="focus" type="text" class="form-control" placeholder="Focus Event" /> </div> <div class="col"> <input id="selectval" type="text" class="form-control" value="Select me!" disabled /> </div> </div> <div> </div> <form id="myform" action="#"> <div class="form-row"> <div class="col"> <input id="first" type="text" class="form-control" placeholder="First Name" /> </div> <div class="col"> <input id="second" type="text" class="form-control" placeholder="Last name" /> </div> <div class="col"> <button type="submit" class="btn btn-default">Submit</button> </div> </div> </form> <div> </div> <div id="formResult"> <h5 id="result"></h5> </div> </div> </body> </html>
3.2.3.1 Define the jQuery function
Add the following code to the form_event.js
file.
form_event.js
$(document).ready(function() { // blur() $("#blur").blur(function() { $(this).css({"background-color":"#FFE5CC", "color":"white"}); }); // change() $("#change").change(function() { alert("The text has been changed."); }); // focus() $("#focus").focus(function() { $(this).css({ "background-color": "#F1FFE6", "color": "black" }); }); // select() $("#selectval").select(function() { alert("Something is selected."); }); // submit() $("#myform").submit(function(event) { $("#result").text($("#first").val() + " " + $("#second").val()); event.preventDefault(); }); });
3.2.4 Keyboard Events Page
Let us write a simple keyboard events page in the jQueryEventMethods/src/main/webapp/views/
folder. Add the following code to it:
keyboard_events.jsp
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Keyboard Events</title> <!-- ==== Css files ==== --> <link rel="stylesheet" type="text/css" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/4.1.3/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="../resource/css/mystyle.css"> <!-- ==== JavaScript files ==== --> <script src="../resource/js/jquery-3.3.1.min.js"></script> <script> i = 0; $(document).ready(function(){ $("#name").keydown(function(){ $(this).css("background-color", "#CCFFCC"); }); $("#name").keyup(function(){ $(this).css("background-color", "#FFCCFF"); }); $("#name").keypress(function(){ $("#outputText").text(i += 1); }); }); </script> </head> <body> <div class="container"> <h2 class="text-primary text-align">jQuery Keyboard Events Example</h2><hr /> <div> </div> <!-- ==== jQuery Keyboard Events Example ==== ---> <h5>Click on input text and press key-down and key-up</h5> <div> </div> <label for="name">Enter Name: </label><input id="name" type="text" class="form-control" /><div> </div> <p>Key-press count is= <span id="outputText"></span></p> </div> </body> </html>
3.2.5 Mouse Events Page
Let us write a simple mouse events page in the jQueryEventMethods/src/main/webapp/views/
folder. Add the following code to it:
mouse_events.jsp
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Mouse Events</title> <!-- ==== Css files ==== --> <link rel="stylesheet" type="text/css" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/4.1.3/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="../resource/css/mystyle.css"> <!-- ==== JavaScript files ==== --> <script src="../resource/js/jquery-3.3.1.min.js"></script> <script> $(document).ready(function(){ $("p").on({ mouseenter: function(){ $(this).css("background-color", "lightgray"); }, mouseleave: function(){ $(this).css("background-color", "lightblue"); }, click: function(){ $(this).css("background-color", "yellow"); }, dblclick: function(){ $(this).hide(); } }); }); </script> </head> <body> <div class="container"> <h2 class="text-primary text-align">jQuery Mouse Events Example</h2><hr /> <div> </div> <!-- ==== jQuery Mouse Events Example ==== ---> <p>DblClick or Click or Move the mouse pointer over this paragraph.</p> </div> </body> </html>
4. Run the Application
As we are ready for all the changes, let us compile the project and deploy the application on the Tomcat7 server. To deploy the application on Tomat7, right-click on the project and navigate to Run as -> Run on Server
.
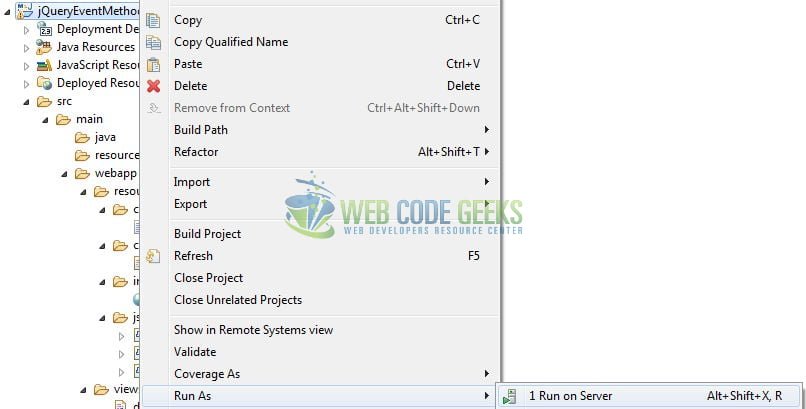
Tomcat will deploy the application in its web-apps folder and shall start its execution to deploy the project so that we can go ahead and test it in the browser.
5. Project Demo
Open your favorite browser and hit the following URL to display the application’s index page as shown in Fig. 7.
http://localhost:8082/jQueryEventMethods/
Server name (localhost) and port (8082) may vary as per your Tomcat configuration.
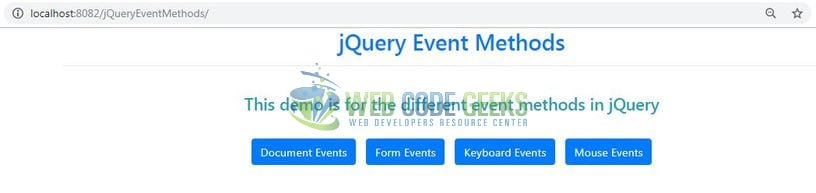
Users can click the buttons to display the result of the different jQuery event methods. That is all for this tutorial and I hope the article served you whatever you were looking for. Happy Learning and don’t forget to share!
6. Conclusion
In this section, developers learned how to create a simple application with the jQuery library.
jQuery event methods allow developers to attach an event handler to the native DOM events. Important DOM manipulation methods are:
click()
dblClick()
change()
submit()
keyup()
keydown()
mouseenter()
mouseleave()
on() etc.
Developers can download the sample application as an Eclipse project in the Downloads section.
7. Download the Eclipse Project
This was an example of jQuery Event Methods for the beginners.
You can download the full source code of this example here: jQueryEventMethods