Node.js Command Line Arguments Tutorial
In this post we will take a look at how to process Command Line Arguments in Node.js. Specifically we will look at how to handle input passed in through the Command Line to Node. Node.js has created quite a buzz in recent times and has won over thousands of developers who are using their knowledge of JavaScript for server side development. So let us get familiar with a feature of this platform.
1. Tools & Technologies
I have used the following toolset to build our sample application. In case you feel more comfortable with some other tools available out there then you can switch out to those.
On the Node.js Website you should see two versions listed. One of them an LTS one and a Current. You should install the LTS version as it is recommended for most users. The LTS version at the time of writing this was v8.9.4. Once you download and install Node, you can go to the command line and run the following commands to make sure things are setup properly.
> node -v
This should return the version of Node installed if everything went well.
> v8.9.4
The download in addition to installing Node will install npm and to check for it you can run the following command.
> npm -v
This will return the version of npm like below:
> v5.6.0
2. Project Layout
The layout of the project looks like the below screen grab:
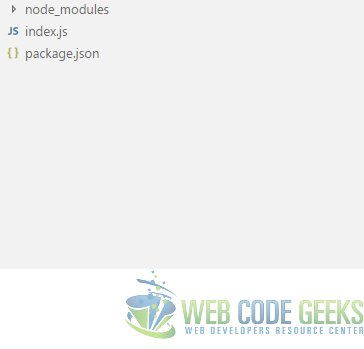
3. Command Line Arguments
Now that we have dealt with basic setup issues, let us now move on to the business at hand. Node.js exposes a global process
object and this object has a argv
property which allows access to the command line arguments. The property is an array and we can loop over it to access the arguments. So let us implement the same in our index.js
file. After making changes the code should look like below:
index.js
var express = require("express"); var app = express(); var hostname = "127.0.0.1"; var port = 8090; //Reading command line arguments and printing them to console process.argv.forEach(function(value, index){ console.log(value); }); app.use(express.static('.')); app.listen(port, hostname);
When we run this code with a command similar to node index.js WCG Post By Siddharth Seth
we should see the following output:
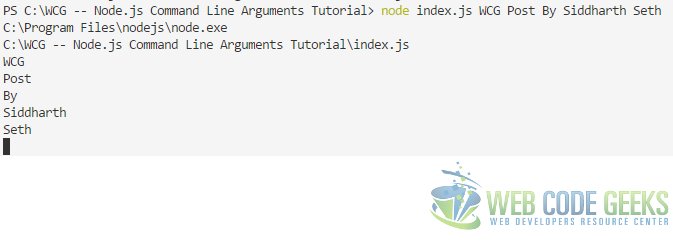
The first argument is the execPath
, the location of Node.js. Followed by the path to the file being executed. These are followed by the command line arguments that we passed in.
One use for this could be launching an application in Dev vs Prod mode. Dev meaning development mode and the Prod mode for Production mode. We could use this for a wide variety of scenarios. In case the keyword dev is passed in we could run all our test cases as opposed to prod mode which could completely skirt around the test cases. We create a file named example.js
which looks as follows.
example.js
var express = require("express"); var tests = require("./tests"); var app = express(); var hostname = "127.0.0.1"; var port = 8090; //Reading command line arguments and printing them to console process.argv.forEach(function(value, index){ if(value === "dev"){ console.log(tests.runTests()); } }); app.use(express.static('.')); app.listen(port, hostname);
An implementation of this could look like below:
example.js
var express = require("express"); var tests = require("./tests"); var app = express(); var hostname = "127.0.0.1"; var port = 8090; //Reading command line arguments and printing them to console process.argv.forEach(function(value, index){ if(value === "dev"){ console.log(tests.runTests()); } }); app.use(express.static('.')); app.listen(port, hostname);
This is very similar to our index.js
file but it has a important difference as it looks for the keyword “dev”. Upon finding it the module runs tests found in another file tests.js
and outputs the results from there. The test.js file looks like below:
tests.js
function runTests(){ return "All tests passed!"; } module.exports.runTests = runTests;
As you can see from the above examples that this is a pretty nifty feature. When we execute the application using command node example.js dev
, we should see the following output:
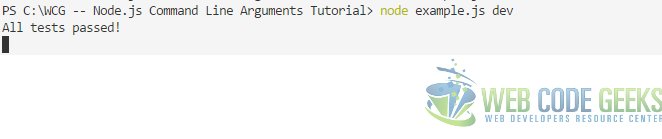
4. Running the Code
To run our application we need to execute the following command in a command window at the root of the project:
> npm install
then
> node index.js argument1, argument2,...
5. Download the Source Code
This wraps up our look at Node.js Command Line Arguments Tutorial.
You can download the full source code of this example here : WCG — Node.js Command Line Arguments Tutorial