PHP Google Geocode Example
In this example we will explain Google Map Geocoding, what it means and how we can manipulate the Google Map Geocoding API. For this example we will need:
- A computer with PHP >= 5.5 installed
- notepad++
1. Getting Started
Lets start by defining Google Map.
According to wikipedia, Google Maps is a web mapping service developed by Google . It offers satellite imagery, street maps , 360° panoramic views of streets (Street View), real-time traffic conditions (Google Traffic), and route planning for traveling by foot, car, bicycle (in beta), or public transportation.
Another good but simpler definition below.
Google map is a web based mapping service that provides access to up-to-date data or information about cities,states countries, continents or regions world wide. Simply put google maps allows us access different geographic data. Google Map provides access it its service through an API (Application Programming Interface).
We can access this API in a number of ways:
- Google Maps Javascript API: This allows you to access google maps service through javascript and according to its website it features a robust support.
To learn more about the Javascript API Visit this tutorial - Google Maps embed API: You do not need any working knowledge of javascript to access Google Map in this way because you do not need to write any code. Just enter the neccessary location and copy the given code.
- Google Street View Image Api.
- Google Static Map Api: Embed a map image without JavaScript or dynamic page loading. With this method you embed a map image and you dont need javascript to do it.
- Google Places API Javascript Library: Up-to-date information about millions of locations.
In this example we are going to develop web apps that use Google geocoding API.
1.1 Google Geocoding
According to Google, Geocoding is the process of converting addresses (like “1600 Amphitheatre Parkway, Mountain View, CA”) into geographic coordinates (like latitude 37.423021 and longitude -122.083739), which you can use to place markers on a map, or position the map. The reverse is also possible, it is called reverse Geocoding.
Reverse geocoding is the process of converting geographic coordinates into a human-readable address. The Google Maps Geocoding API’s reverse geocoding service also lets you find the address for a given place ID. Place IDs uniquely identify a place in the Google Places database and on Google Maps.
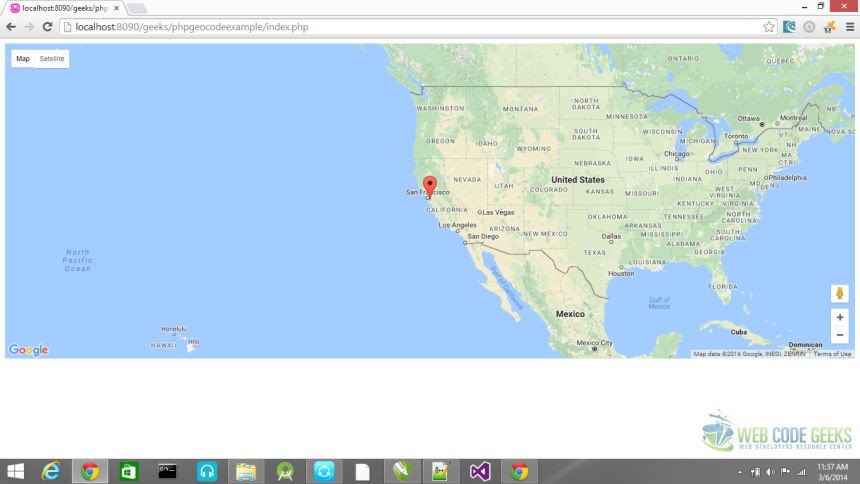
index.html
<!DOCTYPE html> <html lang=eng> <head> <style> #map { height: 400px; width: 100%; } </style> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0"/> <!DOCTYPE html> <html lang=eng> <head> <style> #map { height: 400px; width: 100%; } </style> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0"/> <body> <h1> Google Map Geocoding Example </h1> <form method=post action="index.php"> <select name=cali> <option value ="1600+Amphitheatre+Parkway,+Mountain+View,+CA">California</option> </select> <input type=submit name=button value=submit> </form> <?php } ?> </body> </html>
The above html page contains a drop down list inside a form which has a single address. When the submit button is clicked, the form is submitted and the content of the Drop down list is transmitted to the server (to a script called index.php) .
index.php
<?php if(isset($_POST["button"])){ $lati=null; $longi=null; $address = $_POST["cali"]; $address = urlencode($address);//properly encode the url // google map geocode api url $url = "http://maps.google.com/maps/api/geocode/json?address={$address}";//we are getting the response in json // get the json response $resp_json = file_get_contents($url); // decode the json $resp = json_decode($resp_json, true); //the response status would be 'OK', if are able to geocode the given address if($resp['status']=='OK'){ // get the longtitude and latitude data $lat = $resp['results'][0]['geometry']['location']['lat']; $long = $resp['results'][0]['geometry']['location']['lng']; ?> <div id=map style="width:100%;height:500px"> </div> <script async defer src="http://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=drawMap"></script> <script> function drawMap() { var pos = {lat: <?php echo $lat ?> , lng:<?php echo $long ?>}; var map = new google.maps.Map(document.getElementById('map'), { zoom: 4, center: pos }); var marker = new google.maps.Marker({ position: pos, map: map }); } </script> <?php } } else{ echo "DID NOT RECEIVE LATITUDE AND LONGITUDE DATA"; } ?>
In this script we process the content sent by the form (lines five and six) and call the geocoding API(lines eight to ten) and tell it to return the required data in json.
$url = "http://maps.google.com/maps/api/geocode/json?address={$address}"
In line 22 we load the google map API, in order to draw the map on a browser.
<script async defer src="http://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=drawMap"></script>
The async attribute allows the browser to continue rendering the rest of your page while the API loads. We pass our API key and add a callback function. (The callback function is called after the script has loaded completely). In this case the function name is drawMap.
In line 26 we create an object which holds the coordinates of the region we want to be displayed. In line 27 we instatiate the map object. We pass our div tag, the zoom value and our coordinate object. In line 31 we create a marker object and pass our map to it. We have successfully used the google map geocoding API to draw a map.
2. Summary
In this example we learnt about Google Maps Geocoding API, how to use it to get the coordinates of a street or area and display on a map. We also learnt about the different ways we can access the Google Map service.
3. Download the source code
You can download the full source code of this example here: phpgeocodingexample
First I would like to thank you for this code. It works just fine for me. I don’t know a lot about interfacing with google maps. PhP, CSS HTML and mysql are what I know. What I don’t know to do is display something like all the universities with in so many miles of a lat,long The site I am building is to assist veterans looking to further their education. The input form will have the city, state, and the number of miles to lint the search. I know you just like all of us are very busy. If you… Read more »
Small typo. Pleease change “initMap” to “drawMap”.
there is no need to use PHP , we can simply send ajax request to http://maps.google.com/maps/api/geocode/json?address={$address} in order to retrieve “lat & long”