Bootstrap Breadcrumb Navigation Example
We will look at Breadcrumb navigation using Bootstrap in this article. Breadcrumb navigation is essentially a display of the hierarchical path taken by the user to reach the current page in the site. It also gives the users the ability to jump back one or more levels to reach back the page they navigated through. In essence it is a trail of links to urls to pages within the site that the user navigated through to reach the current location. Bootstrap provides css classes to style this navigation component which we will take a look in the example that follows.
1. Tools & Technologies
We use the following tools & technologies to implement this example:
1. Bootstrap v3.3.7
2. JQuery v1.12.4
3. Nodejs v6.3.0
4. Express
5. Visual Studio Code IDE
2. Project Structure
We start off building our example project with the following project structure. Although I have used Visual Studio Code IDE, any text editor of your choice would suffice for the purposes of this example.
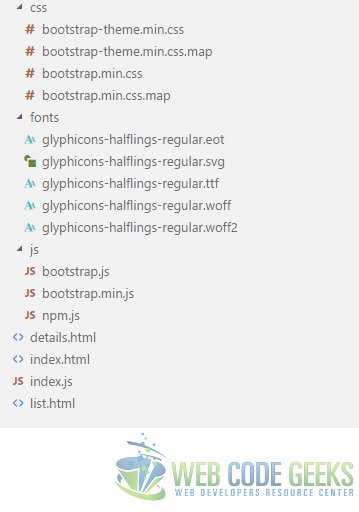
css
This css files from Bootstrap package reside in this folder. All the css stylesheets created by us would reside here as well.
fonts
All the font files that came with Bootstrap are located in this folder.
js
The Bootstrap JavaScript files are placed here along with the file we will create, namely, bootstrap.navbar.js
index.html
This is the file which holds the HTML markup and is our landing page. All the other files are referenced in this one and they come together in the browser and make things happen.
list.html
This page is level 2 and is navigated from index.html
details.html
This one is the last page in the navigation hierarchy with index.html at the top.
index.js
Our barebones web server is built using Node.js and Express module resides in this file.
3. Add Breadcrumbs Navigation
The bootstrap breadcrumbs component is used through decorating an ordered list with the breadcrumb
css class. The list items under this ordered list are hyperlinks to the page named in them. The only exception is the name of the current page which is not displayed using an anchor tag. So, we will add the following html to the corresponding pages to create a breadcrumbs navigation:
Html — index.html
<ol class="breadcrumb"> <li class="active">Home</li> </ol>
Html — list.html
<ol class="breadcrumb"> <li><a href="/index.html">Home</a></li> <li class="active">List</li> </ol>
Html — details.html
<ol class="breadcrumb"> <li><a href="/index.html">Home</a></li> <li><a href="/list.html">List</a></li> <li class="active">Details</li> </ol>
These little Html snippets are what is required to add Bootstrap Breadcrumbs Navigation to a page.
Now our pages, namely index.html, list.html, details.html
should look as below:
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- The above 3 meta tags *must* come first in the head; any other head content must come *after* these tags --> <title>Bootstrap Breadcrumbs Example - Web Code Geeks</title> <!-- Latest compiled and minified CSS --> <link rel="stylesheet" href="/css/bootstrap.min.css"> <!-- Optional theme --> <link rel="stylesheet" href="/css/bootstrap-theme.min.css"> <!-- HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries --> <!-- WARNING: Respond.js doesn't work if you view the page via file:// --> <!--[if lt IE 9]> <script src="https://oss.maxcdn.com/html5shiv/3.7.3/html5shiv.min.js"></script> <script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script> <![endif]--> </head> <body> <div class="panel panel-info"> <div class="panel-heading"> <h1>WebCodeGeeks - Bootstrap Breadcrumbs</h1> <ol class="breadcrumb"> <li class="active">Home</li> </ol> </div> <div class="panel-body"> <a href="/list.html">Navigate to the Listings page</a> </div> </div> <!-- jQuery (necessary for Bootstrap's JavaScript plugins) --> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <!-- Include all compiled plugins (below), or include individual files as needed --> <!-- Latest compiled and minified JavaScript --> <script src="./js/bootstrap.min.js"></script> </body> </html>
list.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- The above 3 meta tags *must* come first in the head; any other head content must come *after* these tags --> <title>Bootstrap Breadcrumbs Example - Web Code Geeks</title> <!-- Latest compiled and minified CSS --> <link rel="stylesheet" href="/css/bootstrap.min.css"> <!-- Optional theme --> <link rel="stylesheet" href="/css/bootstrap-theme.min.css"> <!-- HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries --> <!-- WARNING: Respond.js doesn't work if you view the page via file:// --> <!--[if lt IE 9]> <script src="https://oss.maxcdn.com/html5shiv/3.7.3/html5shiv.min.js"></script> <script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script> <![endif]--> </head> <body> <div class="panel panel-info"> <div class="panel-heading"> <h1>WebCodeGeeks - Bootstrap Breadcrumbs</h1> <ol class="breadcrumb"> <li><a href="/index.html">Home</a></li> <li class="active">List</li> </ol> </div> <div class="panel-body"> <a href="/details.html">Navigate to the Details Page</a> </div> </div> <!-- jQuery (necessary for Bootstrap's JavaScript plugins) --> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <!-- Include all compiled plugins (below), or include individual files as needed --> <!-- Latest compiled and minified JavaScript --> <script src="./js/bootstrap.min.js"></script> </body> </html>
details.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- The above 3 meta tags *must* come first in the head; any other head content must come *after* these tags --> <title>Bootstrap Breadcrumbs Example - Web Code Geeks</title> <!-- Latest compiled and minified CSS --> <link rel="stylesheet" href="/css/bootstrap.min.css"> <!-- Optional theme --> <link rel="stylesheet" href="/css/bootstrap-theme.min.css"> <!-- HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries --> <!-- WARNING: Respond.js doesn't work if you view the page via file:// --> <!--[if lt IE 9]> <script src="https://oss.maxcdn.com/html5shiv/3.7.3/html5shiv.min.js"></script> <script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script> <![endif]--> </head> <body> <div class="panel panel-info"> <div class="panel-heading"> <h1>WebCodeGeeks - Bootstrap Breadcrumbs</h1> <ol class="breadcrumb"> <li><a href="/index.html">Home</a></li> <li><a href="/list.html">List</a></li> <li class="active">Details</li> </ol> </div> <div class="panel-body"> </div> </div> <!-- jQuery (necessary for Bootstrap's JavaScript plugins) --> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <!-- Include all compiled plugins (below), or include individual files as needed --> <!-- Latest compiled and minified JavaScript --> <script src="./js/bootstrap.min.js"></script> </body> </html>
4. Run the example project
Now since we are done with the edit of our files, it’s time to run them and see them in the browser. To do that, we need to run the following commands at the root of the project:
> npm install
> node index.js
Now we need to navigate to http://localhost:3010
in a browser window. Once you do this, you should see the below. Try navigating back and forth using the breadcrumb and the anchor tag below on each page and you can see the breadcrumb navigation in action.
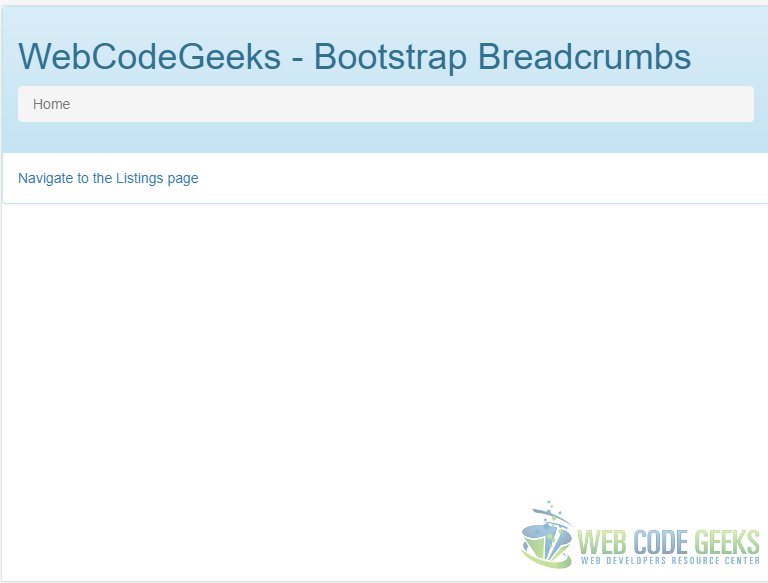
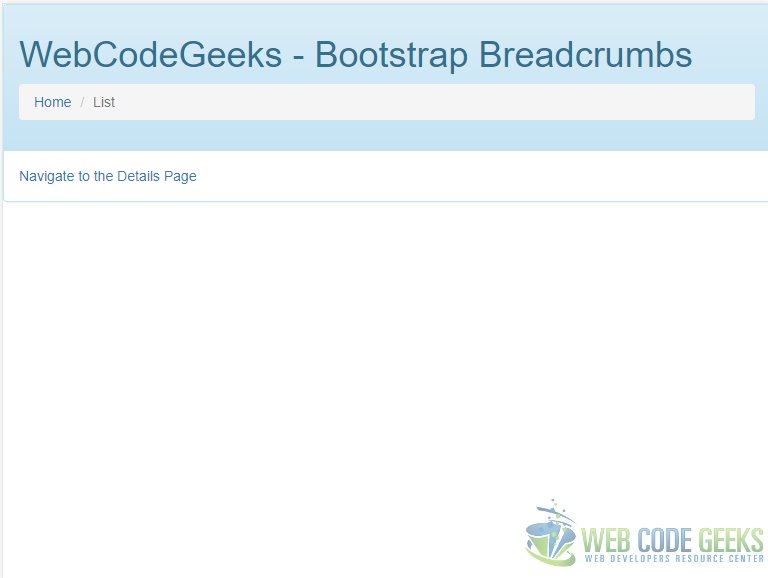
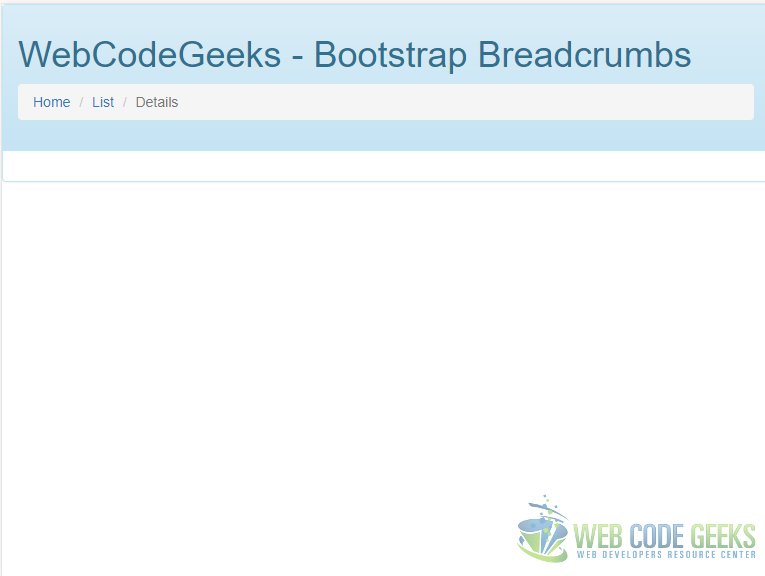
5. Download the Source Code
Download the source code for this tutorial using below link:
You can download the full source code of this example here: Bootstrap Breadcrumb Example