CSS Gradient Background Example
The aim of this example is to create and style gradient backgrounds.
Gradients are typically one color that fades into another, but in CSS you can control every aspect of how that happens, from the direction to the colors (as many as you want) to where those color changes happen.
CSS gradients are new types of image
added in the CSS3 Image Module. Using CSS gradients lets you display smooth transitions between two or more specified colors. This lets you avoid using images for these effects, reducing download time and bandwidth usage.
Browsers support two types of gradients: linear, defined with the linear-gradient()
function, and radial, defined with radial-gradient()
.
1. Basic Document Setup
Go ahead and create a new html document and add the basic sytnax in it like so:
<!DOCTYPE html> <html> <head> <title>CSS3 Gradient Background Example</title> </head> <body> <!-- STYLE SECTION --> <style type="text/css"> </style> <!-- HTML SECTION --> </body> </html>
To set up our HTML, lets just add a div with some text inside, there is nothing else we need for now.
<!-- HTML SECTION --> <div class="gradient_nr">Gradient Background</div>
Also, in your CSS code set up a box-like view of the current div.
<!-- STYLE SECTION --> <style type="text/css"> div { font-family: "Raleway"; margin: 5em; width: 25em; height: 8em; border: 1px solid #ccc; text-align: center; line-height: 8em; } </style>
For now, we’d have a box with a centered text, it is here inside the box that gradient backgrounds are going to be added.
2. Linear Gradients
Gradients of this type can be applied using the syntax below (you can replace background
with background-image
) background: linear-gradient(direction, color-stop1, color-stop2, ...);
.
Let’s see an example of a gradient with two color stops and declared to be compatible with all browsers:
<!-- STYLE SECTION --> <style type="text/css"> gradient1 { background: -webkit-linear-gradient(green, blue); /* For Safari 5.1 to 6.0 */ background: -o-linear-gradient(green, blue); /* For Opera 11.1 to 12.0 */ background: -moz-linear-gradient(green, blue); /* For Firefox 3.6 to 15 */ background: linear-gradient(green, blue); /* Standard syntax (must be last) */ } </style>
Notice we haven’t set a direction for the gradient, that means the default one is top to bottom as you can see.
Another example of a linear gradient would be a gradient with multiple color:
<!-- STYLE SECTION --> <style type="text/css"> .gradient2 { background: -webkit-linear-gradient(green, blue, red); /* For Safari 5.1 to 6.0 */ background: -o-linear-gradient(green, blue, red); /* For Opera 11.1 to 12.0 */ background: -moz-linear-gradient(green, blue, red); /* For Firefox 3.6 to 15 */ background: linear-gradient(green, blue, red); /* Standard syntax (must be last) */ } </style>
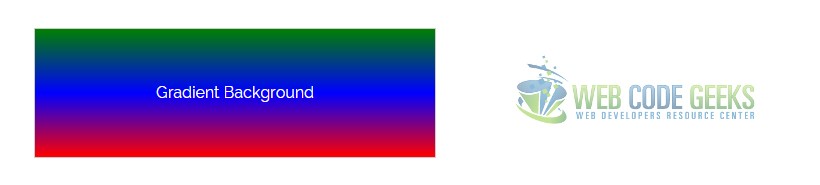
Enough with top to bottom directions. The following will show a left to right gradient, as easy as adding a left
keyword before colors.
<!-- STYLE SECTION --> <style type="text/css"> .gradient3 { background: -webkit-linear-gradient(left, darkorange, lightcoral, floralwhite); background: -o-linear-gradient(left, darkorange, lightcoral, floralwhite); background: -moz-linear-gradient(left, darkorange, lightcoral, floralwhite); background: linear-gradient(left, darkorange, lightcoral, floralwhite); } </style>
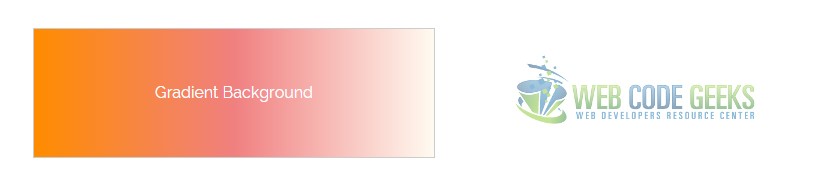
But the direction keyword in not limited to one, we can have somehting like the following to create a diagonal gradient:
<!-- STYLE SECTION --> <style type="text/css"> .gradient4 { background: -webkit-linear-gradient(left top, darkorange, lightcoral); background: -o-linear-gradient(left top, darkorange, lightcoral); background: -moz-linear-gradient(left top, darkorange, lightcoral); background: linear-gradient(left top, darkorange, lightcoral); } </style>
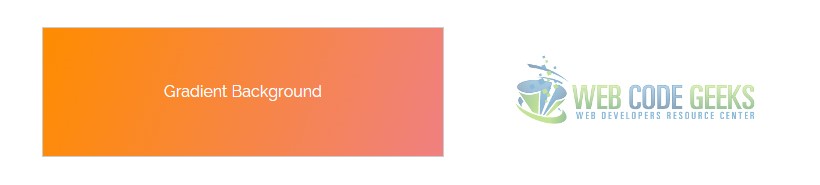
The last example for this section will be a gradient, the direction of which is declared in degrees and colors in HEX.
<!-- STYLE SECTION --> <style type="text/css"> .gradient5 { background: -webkit-linear-gradient(180deg, #b7e8f2, #90c7e6 ); background: -o-linear-gradient(180deg, #b7e8f2, #90c7e6 ); background: -moz-linear-gradient(180deg, #b7e8f2, #90c7e6 ); background: linear-gradient(180deg, #b7e8f2, #90c7e6); } </style>
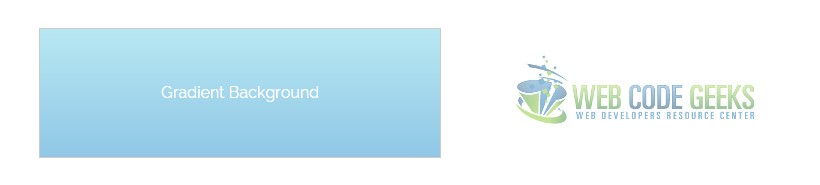
3. Radial Gradients
A radial gradient is defined by its center. You must declare at least two colors for it to work. The syntax would be:
background: radial-gradient(shape size at position, start-color, ..., last-color);
Let’s start with a radial gradient made of three colors set in hexadecimal.
<!-- STYLE SECTION --> <style type="text/css"> .gradient6 { background: -webkit-radial-gradient(#FF530D, #E82C0C, #E80C7A); /* Safari 5.1 to 6.0 */ background: -o-radial-gradient(#FF530D, #E82C0C, #E80C7A); /* For Opera 11.6 to 12.0 */ background: -moz-radial-gradient(#FF530D, #E82C0C, #E80C7A); /* For Firefox 3 to 15 */ background: radial-gradient(#FF530D, #E82C0C, #E80C7A); /* Standard syntax */ } </style>
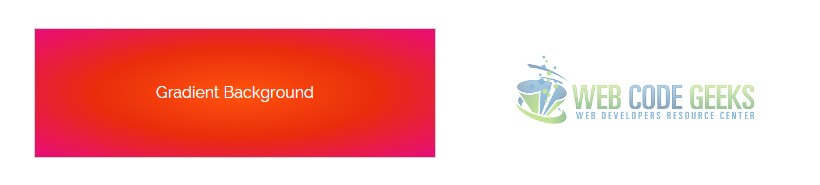
Next, we’ll see a gradient that doesn’t have equal space between color steps. Instead, we decide how much space each color uses.
<!-- STYLE SECTION --> <style type="text/css"> .gradient7 { background: -webkit-radial-gradient(#E8E37B 5%, #DBE899 15%, #62EEFF 80%); background: -o-radial-gradient(#E8E37B 5%, #DBE899 15%, #62EEFF 80%); background: -moz-radial-gradient(#E8E37B 5%, #DBE899 15%, #62EEFF 80%); background: radial-gradient(#E8E37B 5%, #DBE899 15%, #62EEFF 80%); } </style>
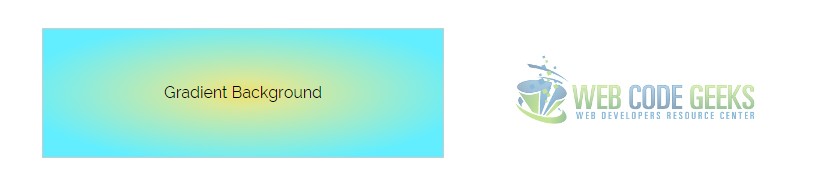
Last but not least, you can define shapes which will guide the gradient colors.
<!-- STYLE SECTION --> <style type="text/css"> .gradient8 { background: -webkit-radial-gradient(circle, #DEE8AE, #B097E8,#FF9134); background: -o-radial-gradient(circle, #DEE8AE, #B097E8,#FF9134); background: -moz-radial-gradient(circle, #DEE8AE, #B097E8,#FF9134); background: radial-gradient(circle, #DEE8AE, #B097E8,#FF9134); } </style>
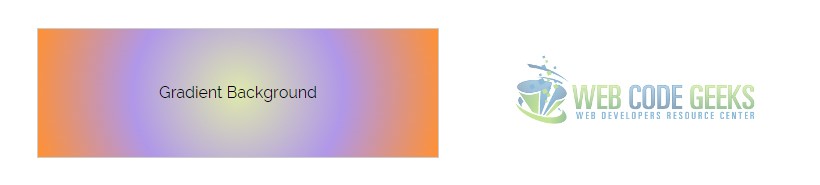
4. Conclusion
To conclude, it is clear that gradients are an easy and fancy way to style your backrounds around the page. There are even
more tools like gradient repeating that you can try. Additionally, there are dozens of websites that let you style color
based gradients in a graphical way and then give you the CSS for that.
A website I would recommend is CSS Matic. However, if you feel creative enough to style your own gradients, that is better and helps you understand.
Gradients are the best way to avoid images that show the same in terms of performance and readability.
5. Download
You can download the full source code of this example here: CSS3 Gradient Background