CSS Text Shadow Example
Ever felt chaotic about text going into unsuitable button colors, boxes or simply sitting inside a bad contrast in the page?
Well, it’s probably because you’ve used colors that won’t match to give a good view of the text, (e.g make it readable).
With CSS, it is very easy to solve this using the text-shadow
property to enhance the text readability and contrast.
In this example, we’re going to use text shadows in some really essential cases which will later enable you to explore more.
1. Basic Set Up
First, go ahead and create a new html file and add the basic html
and css
like this:
<!DOCTYPE html> <html> <head> <title>CSS Buttons</title> </head> <body> <!-- STYLE SECTION --> <style type="text/css"> </style> <!-- HTML SECTION --> </body> </html>
On the html section, add a <h1>
tag with a class of text
like so:
<!-- HTML SECTION --> <h1 class="text">Web Code Geeks</h1>
And this is the element we will be adding shadow on.
I have given this element some initial properties to make it look good on the screen:
<!-- STYLE SECTION --> <style type="text/css"> body{ font-family: "Arial", "sans-serif"; /* just a custom font */ } h1 { /* centered the text for a better view */ margin-left: 7em; margin-top: 5em; } </style>
Now let’s give the text the text-shadow attribute.
Before doing that, let us explain what are the inputs of this attribute:
text-shadow: 4px 4px 4px #ccc;
4px
– X (horizontal) offset
3px
– Y (vertical offset
2px
– blur amount
#ccc
– color
Basically, you can see it like this:
text-shadow: horizontal-offset vertical-offset blur color;
where the color can be either in hex
#ccc;
code or rgba(0,0,0,0.3);
code.
Applying the shadow to our text in css would look like this:
<!-- STYLE SECTION --> .text { font-size: 5em; /* just made text larger */ text-shadow: 4px 3px 2px #ccc; }
The view in the browser of this text with shadow would be:
2. Variations
From now on, the body
background color will be subject to constant change.
This is because certain shadows need specific backgrounds to be noticed.
Also the text is going to be uppercase for better results.
The following will show some great text-shadows you can apply.
2.1 Pressed Effect
Set your text color to a slightly darker shade than your background color.
Next, apply a slight white text-shadow with a reduced opacity.
body { background: #222; } .text { font-size: 5em; color: rgba(0,0,0,0.6); /* added text-color */ text-shadow: 2px 2px 3px rgba(255,255,255,0.1); /* added shadow */ }
Using the rgba
color code, you will be able to set the opacity
of the color applied.
Notice that the text-color has an opacity of 60% (0.6) and shadow 10% (0.1).
2.2 Hard Shadow Effect
Because of their retro nature, hard shadows don’t always need to have blur applied.
Take for example this hard text shadow:
body { background: #fff3cd; /* changed body background color */ } .text { font-size: 5em; color: white; /* changed text-color to white */ text-shadow: 6px 6px 0px rgba(0,0,0,0.2); /* added retro-feel shadow */ }
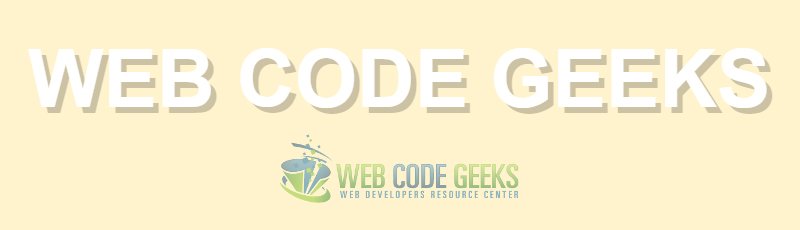
2.3 Double Shadow Effect
It is interesting to know that you are not limited to one single shadow application.
You can use more than one shadow like this: text-shadow: shadow1, shadow2, shadow3;
Let’s add two shadows, one of them with the color of the background, and the other one a slightly darker color than the background color:
.text { font-size: 5em; text-shadow: 4px 3px 0px #fff, 9px 8px 0px rgba(0,0,0,0.15); /* given two shadows */ }
In this case, the background is white, so we don’t need a custom color for it.
The view in the browser would be:
2.4 Distant Down Shadow Effect
This effect lies on the multi-shadow capability of css.
Below, you can see 4 shadows pointing down with various degrees.
body { background: #fff3cd; /* changed body background color */ } .text { font-size: 5em; color: white; text-shadow: 0px 3px 0px #b2a98f, 0px 14px 10px rgba(0,0,0,0.15), 0px 24px 2px rgba(0,0,0,0.1), 0px 34px 30px rgba(0,0,0,0.1); }
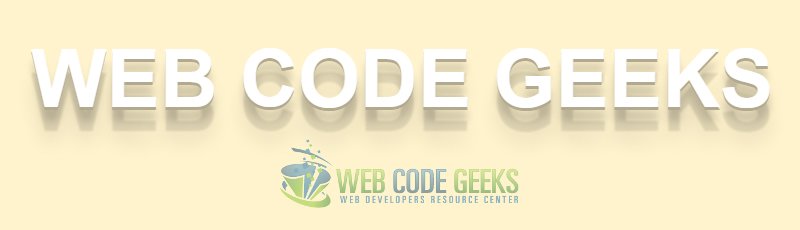
2.5 Mark Dotto’s 3D Text Effect
The following example is just as impressive as you might be wondering.
It comes from MarkDotto.com and utilizes an impressive 12 separate shadows to pull off a very believable 3D effect.
body { background: #3495c0; /* changed body background color */ } .text { font-size: 5em; color: white; text-shadow: 0 1px 0 #ccc, 0 2px 0 #c9c9c9, 0 3px 0 #bbb, 0 4px 0 #b9b9b9, 0 5px 0 #aaa, 0 6px 1px rgba(0,0,0,.1), 0 0 5px rgba(0,0,0,.1), 0 1px 3px rgba(0,0,0,.3), 0 3px 5px rgba(0,0,0,.2), 0 5px 10px rgba(0,0,0,.25), 0 10px 10px rgba(0,0,0,.2), 0 20px 20px rgba(0,0,0,.15); }
Now look at this:
2.6 Gordon Hall’s True Inset Text Effect
Gordon uses some serious CSS voodoo to pull off not only an outer shadow but a genuine inner shadow as well.
body { background: #cbcbcb; /* changed body background color */ } .text { font-size: 5em; color: transparent; background-color: #666666; -webkit-background-clip: text; -moz-background-clip: text; background-clip: text; text-shadow: rgba(255,255,255,0.5) 0px 3px 3px; }
And that gives and incredible true inset text effect.
2.7 Glowing Text Shadow Effect
body { background: #992d23; /* changed body background color */ } .text { font-size: 5em; color: white; text-shadow: 0px 0px 6px rgba(255,255,255,0.7); }
This shadow will create the effect of a glowing text.
2.8 Soft Emboss Shadow Effect
body { background: #629552; /* changed body background color */ } .text { font-size: 5em; color: rgba(0,0,0,0.6); text-shadow: 2px 8px 6px rgba(0,0,0,0.2), 0px -5px 35px rgba(255,255,255,0.3); }
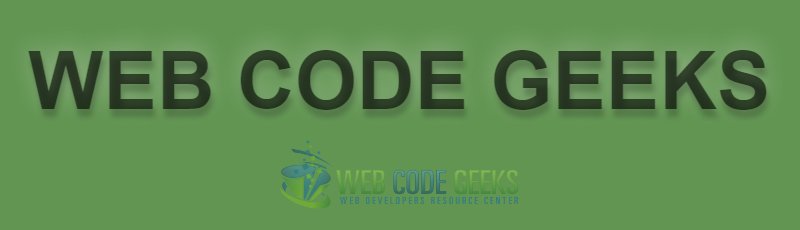
3. Conclusion
As you saw in this example, the text-shadow property is really easy to use.
What’s interesting, is that you can come up with creative effects if you put yourself into it.
These effects are used all over the web (I did not ‘invent’ them) so be sure you are using qualitative stuff.
You can also download the source html and just edit these examples that you saw above.
You can download the full source code of this example here: CSS Text Shadow
Useful tips , thanks )