HTML5 AutoComplete Example
In this article I will show you an example on how to create an autocomplete input using HTML5.
First I will present you what HTML5 added to create autocomplete fields.
Then we will create a real example, with data loaded from a server request (aJax).
1. Elements and attributes
1.1 The autocomplete attribute
IMPORTANT : This attribute is not used to create autocomplete field but to turn off the form autocompletion by the browser …
<form method="post" autocomplete="off" action="post.php"> <!-- Some form elements ...--> </form>
With the example above, the browser will not try to fill the form elements itself.
NOTE : This attribute was introduced in IE 5 ! So it’s not from HTML5 …
I presented this attribute just to remove any ambiguity.
1.2 The datalist element
HTML5 introduced the datalist
tag, this is “THE” tag to create autocomplete field.
The element can contain some option
.
The datalist
has no representation if it’s not linked to an input field, for example the code below will show an empty document :
<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title>HTML5 Autocomplete Example - First</title> </head> <body> <datalist> <option>Paris</option> <option>London</option> <option>Brussels</option> <option>Madrid</option> <option>Roma</option> <option>Dublin</option> <option>Berlin</option> <option>Bucharest</option> </datalist> </body> </html>
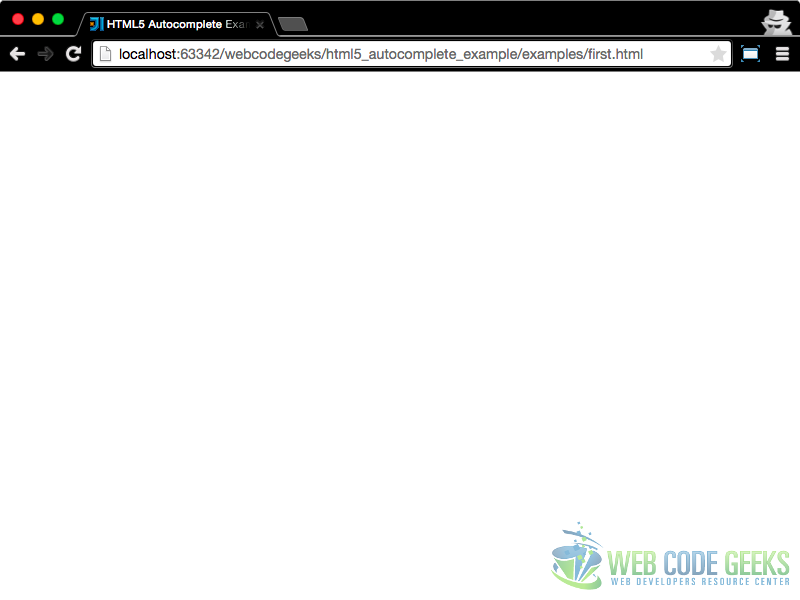
1.3 Link the datalist
Then if you link the datalist to an input field you will have an autocomplete field …
<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title>HTML5 Autocomplete Example - First</title> </head> <body> <input type="text" list="capitals" /> <datalist id="capitals"> <option>Paris</option> <option>London</option> <option>Brussels</option> <option>Madrid</option> <option>Roma</option> <option>Dublin</option> <option>Berlin</option> <option>Bucharest</option> </datalist> </body> </html>
Very simple no ?
Let see a full example.
2. Full Example
In this example we will create an autocomplete field with data retrieved from an Ajax Request.
2.1 Why jQuery
In this example we will use the jQuery library. I inject the library by passing it as a dependency of my code :
(function($){ 'use strict'; })(jQuery);
This allows me to isolate my code, and to show the reader that jQuery is needed, and that my code depends on it …
This library uses the $
symbol as a function name.
In the code I’ve used it 3 times :
- First as a shorthand to the
load
event :$( functionLoadHandler );
- Second in the
loadDatas
function. I’ve use the$.getJSON( urlToJSONData, callbackHandler);
. This function will make a request (AJAX) to theurlToJSONData
, parse the response in JSON, then pass it to thecallbackHandler
function. - Third in the
createList
function. There, I’ve used jQuery to manipulate the DOM, retrieve element (with idcapitallist
), create elements (option
) , and append the created element to the retrieved element.
2.2 The Base document
Here is the HTML Document :
<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title>HTML5 Autocomplete Example - </title> </head> <body> <h2>Enter the first letters of a city ...</h2> <input type="text" name="capitals" list="capitallist" id="capitals" placeholder="capital ..." /> <datalist id="capitallist"></datalist> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.4/jquery.min.js"></script> <script src="script.js"></script> </body> </html>
As you can see, I will use jQuery for the Ajax Request but also for the DOM manipulation.
I have created and empty datalist
that will contains the option
elements.
2.3 The JSON datas
I made a Google search to find a list of World capitals, and I’ve found this article, and this file :
http://techslides.com/demos/country-capitals.json.
The structure of a model is :
{ "CountryName":"France", "CapitalName":"Paris", "CapitalLatitude":"48.86666666666667", "CapitalLongitude":"2.333333", "CountryCode":"FR", "ContinentName":"Europe" }
As you can see there is more data than I need so I will have to extract capital names from the list.
2.4 The JavaScript code
We can create a JavaScript function to make the Ajax Request :
// The JSON list url var capitals = "country-capitals.json"; /** * Load data and call callback function * @param {Function} callback */ function loadDatas( callback ){ // Make the ajax call $.getJSON(capitals, function(list){ // create the Capitals array var capitals =[]; for(var i=0; i < list.length; i++){ capitals.push(list[i].CapitalName); } // Call the function that will create the options // But sort the array first (for better user experience) callback(capitals.sort()); }); }
Then a function that will create the options in the datalist
elements :
/** * Create the options from the capitals array * @param {Array} capitals */ function createList(capitals) { // get the datalist element var datalist = $("#capitallist"); // Fill it with the capitals array for(var i=0; i < capitals.length; i++){ $('<option>'+capitals[i]+'</option>').appendTo(datalist); } }
And then all together …
(function($){ 'use strict'; // The JSON list url var capitals = "country-capitals.json"; /** * Create the options from the capitals array * @param {Array} capitals */ function createList(capitals) { // get the datalist element var datalist = $("#capitallist"); // Fill it with the capitals array for(var i=0; i < capitals.length; i++){ $('<option>'+capitals[i]+'</option>').appendTo(datalist); } } /** * Load data and call callback function * @param {Function} callback */ function loadDatas( callback ){ // Make the ajax call $.getJSON(capitals, function(list){ // create the Capitals array var capitals =[]; for(var i=0; i < list.length; i++){ capitals.push(list[i].CapitalName); } // Call the function that will create the options // But sort the array first (for better user experience) callback(capitals.sort()); }); } // jQuery OnLoad ... $(function(){ loadDatas( createList ); }); })(jQuery);
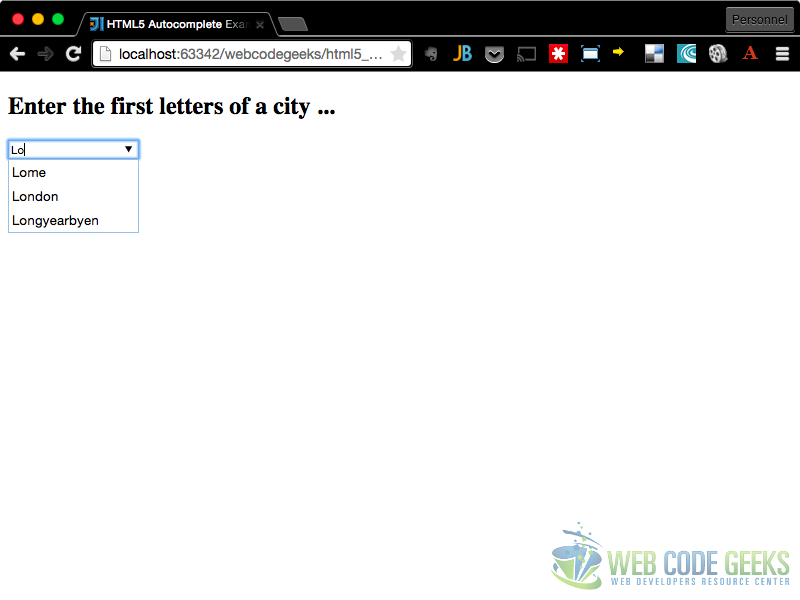
3. Download
You can download the full source code of this example here: HTML5 AutoComplete Example