Angular.js Controller Example
Hello! In today’s example we ‘ll see how do Angular’s controllers
work.
To get into this, I chose a simple form concept, where the user is prompt to insert his username. This updates an informative message (i.e. “Your username is “); I’ll also include a reset button, for demonstration purposes.
1. Required Background
Before getting into the technical part of this example, let me introduce you the $scope
element.
1.1 What are scopes?
AngularJS supports the MVC pattern, with the $scope
object associated with the application model. In fact, it’s the glue between View and Controller. In addition, they hold the Model data that we need to pass to View and use Angular’s two way data binding to bind model data to View.
Their responsibility is to initialize the data that the View needs to display. Scopes are arranged in hierarchical structure which mimic the DOM structure of the application.
1.2 What are controllers?
Generally, an Angular controller is a JavaScript constructor function that augments the $scope
object. It can be attached to the DOM through the ng-controller
directive, where Angular instantiates a new Controller object, using the specified controller’s constructor function. Using a controller, we can instantiate a new child scope as an injectable parameter to controller’s function. The injected parameter is accessible through $scope
.
1.2.1 How to initialize the state of a $scope
object
When creating an application, we need to set up the initial state for the Angular $scope
. This can be feasible by attaching properties to the $scope
object. These properties contain the View (the Model, which will be presented by the View). All the $scope
properties will be available to the template at the point in the DOM, where the controller is registered.
1.2.2 A small example
We here create a HelloController, which attaches a helloMessage
property containing the string “Hello World!” to the $scope
:
var myApp = angular.module('myApp',[]); myApp.controller('HelloController', ['$scope', function($scope) { $scope.helloMessage= 'Hello World!'; }]);
What is actually done, is an Angular module creation ( myApp
) for our app. We then add the controller’s constructor function to the module, using the .controller()
method. This keeps the controller’s constructor function out of the global scope.
Next, we attach our controller to the DOM using the ng-controller
directive. The helloMessage
property can now be data-bound to the template:
<div ng-controller="HelloController"> {{ helloMessage }} </div>
1.2.3 Controllers’ use case
Generally, we usually use controllers either to setup the initial state of the $scope
object or to add behavior to the $scope
object.
Cases that we cannot use controllers are to:
- Manipulate DOM (controllers should contain only business logic).
- Format input; use angular form controls instead.
- Filter output; use angular filters instead.
- Share code or state across controllers; use angular services instead.
- Manage the lifecycle of other components (i.e. to create service instances).
2. Our Example
As I fore-mentioned, a username text field, with a reset button and an updateable message, related to the controller’s logic.
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>AngularJS Controller Example</title> <link rel="stylesheet" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/2.3.2/css/bootstrap.min.css"> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.26/angular.min.js"></script> <script src="script.js"></script> <link rel="stylesheet" href="style.css" > </head> <body ng-app="myApp"> <h2>AngularJS Controller Example</h2> <div ng-controller="UserController"> <form> <label>Name:</label> <input type="text" class="form-control" name="username" ng-model="username"> <button class="btn btn-link" ng-click="reset()">Reset</button> <p>Your username is {{username}}</p> </form> </div> </body> </html>
As line 9 clarifies, our controller’s functionality will be defined into the script.js
file. In line 13, we declare our Angular application in the name of myApp
. Lines 15-23 contain the important div
which interacts with UserController
(this, in conjunction with line’s 9 included script, means that our controller’s logic is contained int the javascript file.
What else is important here, from Angular’s perspective, is the ng-model
attribute of the text field, in line 18. This is actually a directive that binds our text field to the username property on the scope, with the help of UserController, which is created and exposed by this directive.
That is, the text field’s value can be changed inside the controller, by accessing the username property from the scope object (i.e. $scope.username
).
What about line 19 and ng-click
? Well, using the ng-controller
directive, we bind the div
element including its children to the context of UserController
controller. The ng-click
directive will call the reset()
function of our controller, when the reset button is clicked.
In line 21, there is the message that we earlier discussed, where, in general, the double curly braces notation ( {{ }}
) binds expressions to elements.
Enough said for our View’s implementation. Let’s now code the Controller and then explain the necessary details.
script.js
var myApp = angular.module('myApp', []); myApp.controller('UserController', ['$scope', function($scope) { $scope.username = 'unknown'; $scope.reset = function() { $scope.username = ''; }; }]);
At first, we have to define that this script is about an Angular module; especially the one defined in our index.html
, the one named as myApp
application. We then define the controller’s name and initialize the username
with the value of ‘unknown’. That is, when the application is loaded to the browser, the text field will contain a predefined value, but on user change, ng-model
interferes with the controller and updates the username’s value (the curly braces variable binded in the View).
Obviously, the reset function is used to empty the text fiel’s content.
3. Demo
Let’s now display a quick demo. This is the initial view:
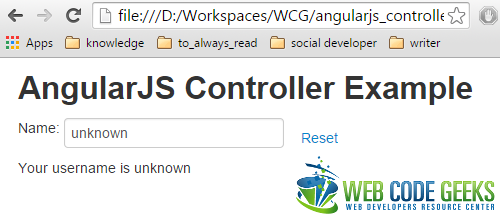
If we hit the reset button, our text field gets empty:
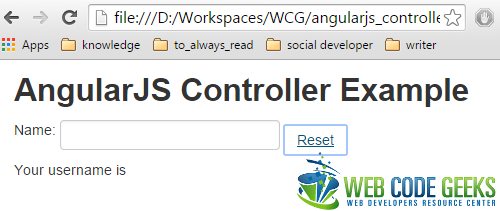
Finally, here is the updated message, which, in conjuction with the user input, result to a dynamic view in our application:
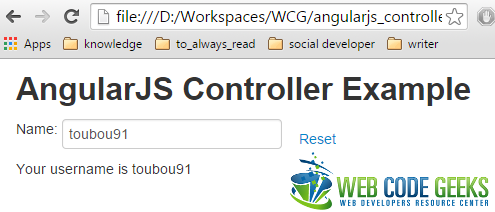
4. Download the project
This was an example of an AngularJS Controller.
You can download the full source code of this example here : angularjs_controller.zip
I think using the “controller as” is not the recommended approach.
I mean: I think using the “controller as” is the recommended approach.
Dear Erron,
thank you for comment.
I cannot claim that the presented-here approach is worse than the “controller as” one, as each one has separate features that developers has to firstly think about and then consider which one would fit better to the specified needs.
Nevertheless, I plan writing a “controller as” example, too, as it seems to be used in Angular.js 2.0.