Angular.js Data Binding Example
Hey! Today we ‘ll see how we can easily update our angular views, using ngModel. To begin with, according to angular applications, data-binding is the automatic synchronization of data between the model and view components (for those that didn’t get this at all, please take a look over the MVC pattern).
Regarding Angular’s implementation, the view is a projection of the model at all times. When the model changes, the view reflects the change, and vice versa.
For the purpose either of a getting a better understanding of the fore-mentioned or the data-binding comparison between classical template systems and Angular’s, please refer to the official documentation.
1. Introduction
1.1 The concept
Today’s concept will demonstrate the implementation of data-binding by displaying the user’s input into the View, according to the Model. Especially, there are two text fields, one that matches a user’s first name, while the second matches the last name and a greeting message, depending on the user’s input.
1.2 What you need to know
1.2.1 Templates
In Angular, templates are written with HTML that contains Angular-specific elements and attributes. Angular combines the template with information from the model and controller to render the dynamic view that a user sees in the browser.
These are the types of Angular elements and attributes you can use:
- Directive — An attribute or element that augments an existing DOM element or represents a reusable DOM component.
- Markup — The double curly brace notation {{ }} to bind expressions to elements is built-in Angular markup.
- Filter — Formats data for display.
- Form controls — Validates user input.
For further details, according to angular templating, please refer to the official documentation.
1.2.2 ngModel
The ngModel
directive binds an input,select, textarea (or custom form control) to a property on the scope using NgModelController, which is created and exposed by this directive.
ngModel has several responsibilities (you can read all of them in the official documentation, as well), but for the purpose of this example, the important one is the responsibility for binding the view into the model, which other directives such as input, textarea or select require.
What is important to know, before diving into the source code of this example, is that ngModel
will try to bind to the property given by evaluating the expression on the current scope. This means that if the property doesn’t already exist on this scope, it will be created implicitly and added to the scope.
2. The Example
One file is enough!
index.html
<!DOCTYPE html> <html ng-app> <head> <meta charset="utf-8"> <title>Angular.js Data Binding Example</title> <script src="//cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.15/angular.min.js"></script> </head> <body> <h2>Angular.js Data Binding Example</h2> <table> <tr> <td>First name:</td> <td><input ng-model="firstName" type="text"/></td> </tr> <tr> <td>Last name:</td> <td><input ng-model="lastName" type="text"/></td> </tr> </table> <br/> Hello {{firstName}} {{lastName}} </body> </html>
First of all, we obviously have to define that this is about an angular application (line 2). Secondly, we build our table view, according to our needs: two rows (first and last name), each one with two columns (the corresponding text field for first and last name). Finally, we display our message in line 21.
The whole process is based on the assignable variables to the ng-model
. That is, this works as a nested angular controller, binding data to our view. This kind of data can be accessed/displayed using the curly brackets angular templating markup.
Generally, HTML’s input
tag, when used together with ng-model
, provides data-binding, input state control, and validation.
3. Demo
Firstly, please take a look at this post, just to understand why you should deploy this app in a local server rather than just executing it in a browser.
Access the web app from your local server:
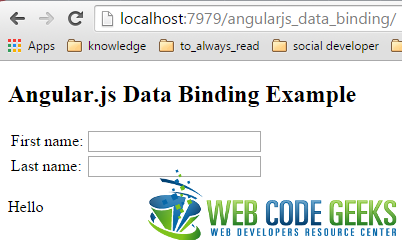
Now, let’s insert some values:
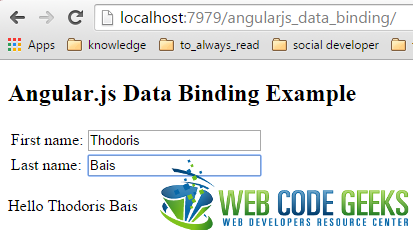
Hooray, it’s giving feedback!
4. Download
This was an example of Angular.js Data Binding.
You can download the full source code of this example here : angularjs_data_binding.zip