Angular.js Form Validation Example
EDITORIAL NOTE: In this post, we feature a comprehensive Angular.js Form Validation Example.
One of AngularJS advantages is the simplicity it provides for validating forms. Today’s example demonstrates a simple way to implement client-side validation using the AngularJS form properties.
Suppose a form with two fields, username and email and two buttons, one for reset and the other with a submit role. Our example’s concept is that both of the form’s fields are required, so the form cannot be submitted if either of these is empty or has invalid format (i.e. the email).
1. View Page
The best way to take advantage of Angular’s abilities, according to form validation, is to attach a controller to our form.
index.html
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/2.3.2/css/bootstrap.min.css"> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.26/angular.min.js"></script> <script src="script.js"></script> <link rel="stylesheet" href="style.css" > </head> <body> <h2>AngularJS Form Validation Example</h2> <form ng-controller="validationCtrl" name="sampleForm" novalidate> <p> <label>Username:</label> <input type="text" class="form-control" name="username" ng-model="username" required > <span ng-show="sampleForm.username.$error.required">Username is required.</span> </p> <p> <label>Email:</label> <input type="email" class="form-control" name="email" ng-model="email" required> <span ng-show="sampleForm.email.$error.required">Email is required.</span> <span ng-show="sampleForm.email.$error.email">Invalid email address.</span> </p> <p> <button class="btn btn-link" ng-click="reset()">Reset</button> <input type="submit" class="btn btn-primary" ng-disabled="sampleForm.$invalid" ng-click="checkData()"> </p> </form> </body> </html>
As you make clear from the head
‘s definition, I decided to separate HTML from Javascript and CSS.
Line 12 declares clarifies that our form is attached to validationCtrl
and yes, your guess is right, the script.js
will contain the controller’s logic, which will be nothing more than a handler for our form’s buttons.
A typical client-side validation process contains error messages, for the cases of invalid (email) or blank input fields.
In Angular, there are discrete form states, such as $dirty
or $invalid
, for user-interaction check with the form and for invalid input fields check, respectively. To read more about form properties, refer to the official documentation.
Generally, this means that we partially want extra messages to be displayed in our webapp. AngularJS provides the ng-show
directive for this purpose, which, in conjunction with the form’s states, results to the client-side validation. Lines 16 and 21-22 implement the validation in the AngularJS way.
We want our submit button to be disabled, while the form is invalid (line 26). AngularJS provides ng-disabled
directive to make this feasible.
Finally, we clarify that we want our controller to run a specific function for each of the form’s buttons, using ng-click
directive.
2. Controller’s Functionality
When our form has a valid state, the submit buttons gets enabled. Suppose there is a predefined acceptable username and email from the webapp and that this the purpose of the reset button: to turn our form to its acceptable format. On the other hand, the submit has to check if the form contains the correct data.
According to AngularJS, the glue between the application controller and the view, is the $scope
object.
script.js
function validationCtrl($scope) { var validUsername = "Thodoris Bais"; var validEmail = "thodoris.bais@gmail.com"; $scope.reset = function(){ $scope.username = validUsername; $scope.email = validEmail; } $scope.checkData = function() { if ($scope.username != validUsername || $scope.email != validEmail) { alert("The data provided do not match with the default owner"); } else { alert("Seems to be ok!"); } } }
3. Demo
Let’s run our app:
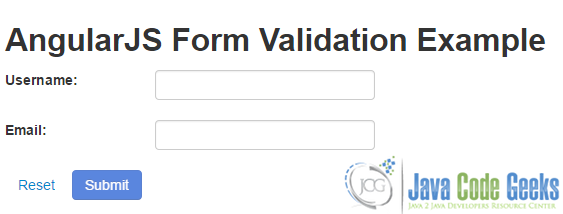
After leaving the email field blank, here’s the job of ng-show
:
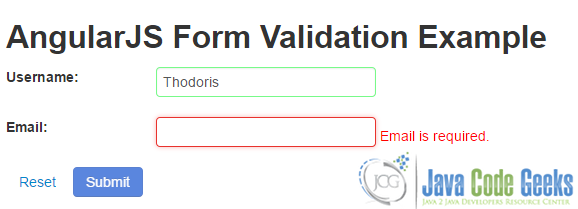
Having provided the valid input data, we firstly notice that the submit button get enabled (both fields have now a green/valid border) :
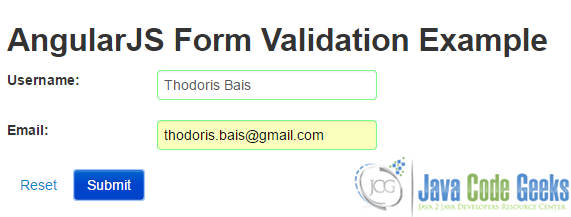
4. Download the project
This was an example of AngularJS Form Validation.
You can download the full source code of this example here : angularjs_form_validation.zip
Thank you..!!
This article is so usefull and very easy to understand at basic level.
I like this.