Angular.js Table Example
Hi there! Today we ‘ll examine a simple table solution, using the Angular.js
framework. Suppose we want to display a list of persons, accompanied with their hobbies, in the gentle packaging of an HTML table
. Let’s see the way over it!
1. Introduction
If you ‘re not enough experienced with Angular, you should first take a glance at a previous example of mine, where the required background for this example, is provided (paragraphs 1.1 and 1.2).
Now that you have a basic understanding of scopes
and cotrollers
, let’s solve our problem!
2. Our Example
To begin with, let’s again separate our View from our Controller (MVC pattern). That is, we ‘ll provide our view in index.html
, whereas the controller’s functionality will remain in script.js
file.
Having understood the MVC pattern
, too, we need our controller to pull the necessary data from the Model
and “feed” the view with them.
I here want to demonstrate a sample table implementation, not an MVC example, so, to make it easier for you, the model’s data is created into the controller, instead of being pulled from the model.
Besides the basic functionality that the $scope
object provides, we can also use it to handle an array with it. We can define an array of persons (with their names and hobbies), into the $scope
object, like below:
$scope.persons = [ {"name": "Thodoris", "hobby": "Gym"}, {"name": "George", "hobby": "Fishing"}, {"name": "John", "hobby": "Basketball"}, {"name": "Nick", "hobby": "Football"}, {"name": "Paul", "hobby": "Snooker"} ];
Ok, now that we know what our controller’s definition will contain, let’s see our view file and we ‘ll get back to the definition of our script, after it:
index.html
<html ng-app="tableApp"> <head> <meta charset="utf-8"> <title>Angular.js Table Example</title> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.26/angular.min.js"></script> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.26/angular-route.min.js"></script> <script src="script.js"></script> </head> <body ng-controller="HobbyCtrl"> <table> <tr> <th>Name</th> <th>Hobby</th> </tr> <tr ng-repeat="person in persons"> <td>{{person.name}}</td> <td>{{person.hobby}}</td> </tr> </table> </body> </html>
We here defined an Angular app (line 1), in the name of tableApp
and attached a controller to it (line 9). This means that our view will search for the controller’s definition/functionality in the script.js
file.
Lines 12 and 13 declare our table’s headers, inside a table row:
<tr> <th>Name</th> <th>Hobby</th> </tr>
At this point, we have to find a way to repeatedly parse all the data from the controller’s persons
array to our table, as we obviously don’t want to exercise our HTML typing.
This results to an extra requirement, too: we want to divide each person’s data, into name and hobby, in order to display each person in the corresponding table’s column.
In order to meet the fore-mentioned requirements, we ‘ll use Angular’s ngRepeat directive, which instantiates a template once per item from a collection. Each template instance gets its own scope, where the given loop variable is set to the current location item, and $index is set to the item index or key.
In our case, to repeatedly loop over each person, we have to assume that each person is a table row:
<tr ng-repeat="person in persons"> </tr>
Now, we can access the person that is processed each time, using the person
variable:
<tr ng-repeat="person in persons"> <td>{{person.name}}</td> <td>{{person.hobby}}</td> </tr>
We ‘ve analyzed our view page, so after a small introduction to the controller, here’s its final structure:
script.js
angular.module('tableApp', []) .controller('HobbyCtrl', function ($scope){ $scope.persons = [ {"name": "Thodoris", "hobby": "Gym"}, {"name": "George", "hobby": "Fishing"}, {"name": "John", "hobby": "Basketball"}, {"name": "Nick", "hobby": "Football"}, {"name": "Paul", "hobby": "Snooker"} ]; });
3. Demo
Here’s a quick demo of the app:
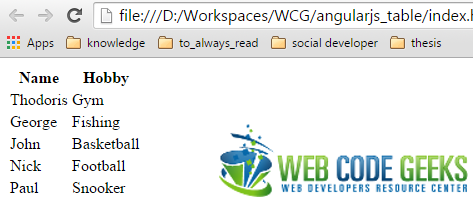
4. Download the project
This was an example of Angular.js Table.
You can download the full source code of this example here : angularjs_table.zip