Angular.js JSON Fetching Example
Hello there! Today’s example’s about displaying data from a JSON file to an Angular.js application.
1. Introduction
1.1 What is JSON
JSON is a lightweight data-interchange format. It is easy for humans to read and write. It is easy for machines to parse and generate. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers.
It is built on two structures:
- A collection of name/value pairs. In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
- An ordered list of values. In most languages, this is realized as an array, vector, list, or sequence.
1.2 Example’s concept
In this example we will demonstrate a simple way to retrieve a JSON
file and display the respective information to our Angular application, in a user-friendly way. Specifically, we ‘ll here deal with countries’ population around the world.
1.2.1 The JSON file
I have already prepared a countries json file, which provides codenames and population data for several countries around the world, like this:
1.2.2 The app itself
So, what’s the plan?
- Fetch the
JSON
. - Display
JSON
‘s information in a more human-readable way.
Fetching the JSON
at first, means that this has to be done each time the app is being loaded to the browser. That is, the corresponding action will take place into the head
‘s script.
Ok, that was the easy part, what about the difficult one? The fore-mentioned “action” is actually a call to the $http
service, a core Angular service that facilitates communication with the remote HTTP servers via the browser’s XMLHttpRequest
object or via JSONP
.
Practically, this means that you have to deploy your app in a web server, rather than executing it in a browser. For further details about this fact, please consult this post.
The general usage of the $http
service is a single argument (configuration object) that is used to generate an HTTP request. It returns a promise with two $http
methods: success
and error
.
Here’s how a simple GET
request looks like:
// Simple GET request example : $http.get('/someUrl'). success(function(data, status, headers, config) { // this callback will be called asynchronously // when the response is available }). error(function(data, status, headers, config) { // called asynchronously if an error occurs // or server returns response with an error status. });
We’ll only make use of the success
and guess what?! On service’s successful call/execution, we want to load our JSON
file into a global variable, in order to be accessible all over the app and yes, your guess was right, $scope
is the global variable that we ‘re searching for!
In addition, the $http
core service, provides shortcut methods, where the only requirement is the URL that has to be processed, whereas the request data must be passed in for POST/PUT requests:
$http.get('/someUrl').success(successCallback);
2. The Example
Time for action!
2.1 Loading JSON into $scope
According to the fore-mentioned notes, we have to load our JSON file into a $scope
variable, let’s say “countries”:
$http.get('countries.json').success(function(data) { $scope.countries = data; });
Obviously, this service call has to be a part of an Angular’s app controller definition, so, assuming that we named our angular app as “countryApp”, here’s the updated format of our service call:
countryApp.controller('CountryCtrl', function ($scope, $http) { $http.get('countries.json').success(function(data) { $scope.countries = data; }); });
If you need further assistance according to Angular Controllers, please take a look at this post.
2.2 Displaying JSON data into a table
Now that we ‘ve loaded all the JSON’s data into $scope.countries
, let’s display them to a table with three columns: code, name, population. This fact is translated into two requirements:
- We have to find a way to repeatedly parse all the data from
$scope.variables
(as we obviously don’t want to exercise our handwriting for more than 70 separate countries). - We want to divide each country’s data, into code, name and population, in order to display each one in the corresponding table’s column
Defining the table’s headers is very easy:
<tr> <th>Code</th> <th>Country</th> <th>Population</th> </tr>
Now, to fullfil the first requirement, we ‘ll use Angular’s ngRepeat
directive, which instantiates a template once per item from a collection. Each template instance gets its own scope, where the given loop vriable is set to the current location item, and $index
is set to the item index or key.
In our case, to repeatedly loop over each country, we have to assume that each country is a table row:
<tr ng-repeat="country in countries"> </tr>
Fullfilling the second requirement is now easier, that the country processed each time can be accessed from the country
variable:
<tr ng-repeat="country in countries | orderBy: 'code' "> <td>{{country.code}}</td> <td>{{country.name}}</td> <td>{{country.population}}</td> </tr>
Here is the final structure of our app:
index.html
<html ng-app="countryApp"> <head> <meta charset="utf-8"> <title>Angular.js JSON Fetching Example</title> <link rel="stylesheet" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/3.3.2/css/bootstrap.min.css"> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.26/angular.min.js"></script> <script> var countryApp = angular.module('countryApp', []); countryApp.controller('CountryCtrl', function ($scope, $http){ $http.get('countries.json').success(function(data) { $scope.countries = data; }); }); </script> </head> <body ng-controller="CountryCtrl"> <h2>Angular.js JSON Fetching Example</h2> <table> <tr> <th>Code</th> <th>Country</th> <th>Population</th> </tr> <tr ng-repeat="country in countries | orderBy: 'code' "> <td>{{country.code}}</td> <td>{{country.name}}</td> <td>{{country.population}}</td> </tr> </table> </body> </html>
3. Demo
Let’s run it in a local server.
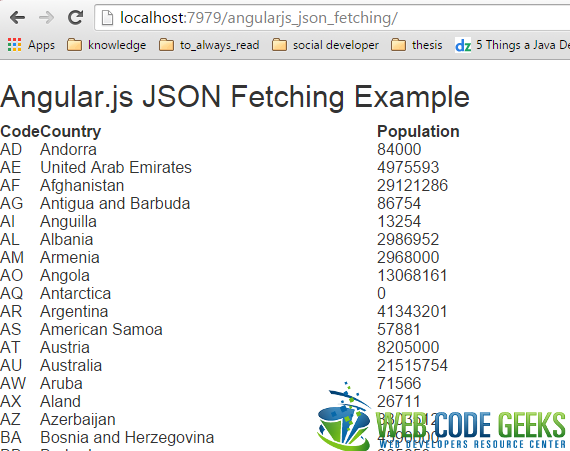
4. Download
This was an example of Angular.js JSON Fetching.
You can download the full source code of this example here : angularjs_json_fetching.zip
The above code works perfectly in firefox but not work in Internet explorer and chrome.
Please provide solution for IE and chrome.
Not working in Latest version of chrome and IE.
Maybe you have no web server. When you were trying to run this code in and your json file path is file://C:…… it will not show the output.
@Asif
i don’t agree with you. The Code works fine in the latest Versions of IE, Chrome and also Firefox.
Hey, how do I provide these JSON inputs, when paginating AngularJS?
Hi, check this link for pagination.
http://www.michaelbromley.co.uk/blog/108/paginate-almost-anything-in-angularjs
this its solve my problem. thnx Thodoris Bais
Its very Useful.very Simple code to execute in JSON file in Angular js….Thanks…great Hoping to all
Hai your tutorial is nice and when i use this method with url its fallback in error section only .what is the reason for that could you please help me??.Thanks :)
thanks for the example
i want to get data from this URL
but when i put this URL i didn’t get any data instead when i put any local file’s URL i got data can u plz tell me why so?
if possible ..mail me the solution.
please give some innovative work of angular js where above example just the hard code.
many are confusing in how to manipulate a JSON data , there are many ways to represent json data and its manipulations.
so many gets confused when they enter IT world without proper training
One of the solution that I found in order to run the code correctly is to copy the folder in the htdocs folder if you are using xampp and rename the file index.html into index.php it will run the code without any problem in internet explorer, google chrome and mozilla firefox as I tested. The issue is that some web browsers disable because of their cross browser file permission. The solution is to run the code in the server will solve all the problem in xampp it uses apache as it’s web server.