Angular.js ng-src Example
Hi there! Today we ‘re gonna see how to include images in our Angular applications.
1. Introduction
In plain HTML, we use the <img>
tag to insert an image to our site. For example, this could be a sample image insertion:
<img src="image.png" alt="A sample image" height="64" width="64">
Now, in terms of Angular.js and image insertion, one could easily claim, that we can similarly refer to an image, by using one of the standard Angular.js templates. I suppose, everyone would go for the “markup” one, with which, we can use the double curly brace notation {{ }}
to bind expressions to elements.
However, using Angular markup like {{hash}}
in the fore-mentioned form, within an HTML src
attribute doesn’t work well, as the browser will fetch from the URL with the literal text {{hash}}
, until Angular replaces the expression inside {{hash}}
.
Angular’s ngSrc
directive solves this problem.
The fore-mentioned “buggy” way to implement is, seems like:
<img src="http://www.gravatar.com/avatar/{{hash}}" alt="Sample avatar"/>
But the correct way to write it, is by using the ngSrc
directive:
<img ng-src="http://www.gravatar.com/avatar/{{hash}}" alt="Sample avatar" />
2. Example’s concept
For our convenience, we ‘ll make use of an existing example concept, the one where I demonstrated the Angular.js JSON Fetching. That is, assuming that we have to display some countries’ data in our page, we may also want to let the user view the country’s flag, too. So, we ‘ll use the ngSrc
directive in conjuction with a JSON file. This is not so close to the introduction section, but I ‘ll cover that usage, too.
3. The Example
My previously linked example, according to JSON fetching, describes in depth how to load and “consume” a json file, into an Angular.js application, so I’ll quickly get into this.
3.1 The JSON file
Here’s a minified version of our JSON file:
countries.json
[ { "name": "Greece", "population": 11000000, "flagURL": "//upload.wikimedia.org/wikipedia/commons/5/5c/Flag_of_Greece.svg" }, { "name": "United Kingdom", "population": 62348447, "flagURL": "//upload.wikimedia.org/wikipedia/en/a/ae/Flag_of_the_United_Kingdom.svg" }, { "name": "United States of America", "population": 312247000, "flagURL": "//upload.wikimedia.org/wikipedia/en/a/a4/Flag_of_the_United_States.svg" } ]
That is, it includes one additional key, compared to Angular.js JSON Fetching‘s one: the flagURL
.
3.2 Fetching the JSON file
We can load a JSON file to an Angular app into the $scope
variable, by calling the $http
service. a core Angular service that facilitates communication with the remote HTTP servers via browser’s XMLHttpRequest
or via JSONP
.
Practically, this means that you have to deploy your app in a web server, rather than executing it in a browser. For further details about this fact, please consult this post.
To do so, we ‘ll reuse the following code snippet from the existing example (JSON fetching), assuming that:
- The Angular app is named as
contryApp
. - Our controller is named as
CountryCtrl
. - Our JSON file is named as
countries.json
and is placed in app’s root folder.
$http.get('countries.json').success(function(data) { $scope.countries = data; });
3.3 Displaying the JSON file
Keeping a similar concept as in the Angular.js JSON Fetching Example let’s see how to display the country’s flag, using the ngSrc
directive:
index.html
<html ng-app="countryApp"> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <link rel="stylesheet" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/3.3.4/css/bootstrap.min.css"> <script src="//cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.0/angular.min.js"></script> <script> var countryApp = angular.module('countryApp', []); countryApp.controller('CountryCtrl', function ($scope, $http){ $http.get('countries.json').success(function(data) { $scope.countries = data; }); }); </script> </head> <body ng-controller="CountryCtrl"> <div class="container"> <h2>Angular.js ng-src Example</h2> <table class="table table-striped"> <tr> <th>Country</th> <th>Population</th> <th>Flag</th> </tr> <tr ng-repeat="country in countries"> <td>{{country.name}}</td> <td>{{country.population}}</td> <td> <img ng-src="{{country.flagURL}}" width="100"> </td> </tr> </table> </div> </body> </html>
Lines 1, 7-14 and 16, fulfill the assumptions made in JSON fetching section. Let’s use an HTML table to group the JSON’s data properly. Lines 21-23 define the table’s headers for the country name, population and flag.
That is, we want to divide each country’s data, into name, population and flag, in order to display each one in the corresponding table’s column. For this purpose, we ‘ll use the ngRepeat
directive, which instantiates a template once per item from a collection. Each template instance gets its own scope, where the given loop variable is set to the current location item, and $index
is set to the item index or key.
When it comes to our case, in order to repeatedly loop over each country, we have to assume that each country is a table row:
<tr ng-repeat="country in countries> </tr>
So, now that the country processed each time can be accessed from the country
variable, it’s easier to understand that lines 26-30 parse each country’s name, population and flag to the corresponding table column.
In addition to what is mentioned in the Introduction section of this example, the official documentation for the ngSrc
, states that the directive can accept any argument of type template
. That is, it accepts any string which can contain {{ }}
markup.
4. Demo
Let’s run it in a local server. For this example, the app can be accessed from a browser in following url: http://localhost:8080/angularjs_ngsrc/
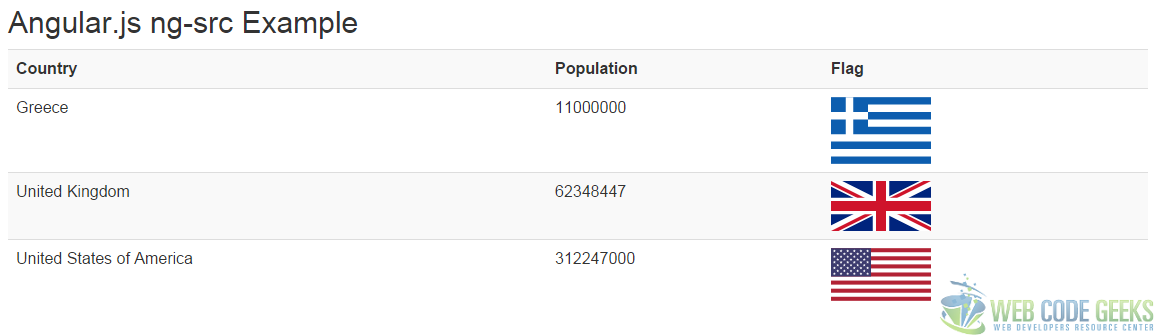
5. Download
This was an example of Angular.js ng-src.
You can download the full source code of this example here : angularjs_ngsrc.zip
try to learn this website
Hey Very Short and Good Example it is..Can you please do one favour for me by implementing the same logic but for many images using lazy load or load more button option??? or give me a hint of it..
Very well explained tutorial about loading images with ng-repeat.It really helps me put but I want to know why people are using Lazy loading script for loading images?