AngularJS Navigation Example
By now you’ve surely heard of the superheroic Javascript framework named Angular, and if not, then you’ll hear about it very soon if web programming is a part of your world, so you had better take a look here! Angular is by far the best in handling dynamic views in HTML, and it appears that the trend will continue. Now that we have established it’s superiority in quite a lot of aspects but mainly in the creation of SPA‘s, let’s take a look at other factors that we would include in our website except for the partial views. The first that comes to mind is navigation!
1. Navigation through routing
When discussing SPA’s it’s unavoidable the discussion on navigation. Let’s suppose we want to create an SPA, and after we create all the necessary code in the main HTML file of our app, we go on creating the partial views we want to show. The next step would be the creation of the controllers for each section and of course, the routing of these partial views, as the Single Page App wouldn’t work without it. However, even though it looks like by conquering all these steps we would be all cool and dandy, we actually wouldn’t. Can you find where we went wrong?
Yes, navigation! We need to have some buttons or triggers of some kind to make it possible for us to pass from one page to the other and back again, or else what we’re looking to build won’t be functional. All the same, the code for that would be so very basic, that even this code snippet would suffice:
index.html
<a href="#/"><button class="btn btn-primary">Homepage</button></a> <a href="#/about"><button class="btn btn-info">About</button></a> <a href="#/date"><button class="btn">Date</button></a>
While you can simply use random links or buttons, as we have done in this example, the best way to go about it is to create proper navbars. Let’s see how we go about it in AngularJS.
2. Navigation in Angular
Even though you could very easily use some make-do triggers instead of proper navbars, AngularJS will not let you suffer through bad code AND bad user experience (and all of the other stuff that comes with anything not thought through). It offers a solution in order to make it possible for you to have proper navigation in your app, and not any navigation, but one that is simple to code, looks good, and is pretty functional. How? Well let’s see it for ourselves.
2.1 The setup
Say we want to create a navigation bar that gives us three navigation options: we could go to Home, to About or to Contact. So we would need three options to click, and to make our choice visible we will print the option we chose for the user.
The first logical step in creating this would be the setup. Except AngularJS, we will also use some minimal Twitter’s Bootstrap to give our app some quick styling, so all this should go in the setup of our app. The code would go like below:
index.html
<!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <link href="style.css" rel="stylesheet"/> <script src="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/3.3.6/js/bootstrap.min.js"></script> <link href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/3.3.6/css/bootstrap.min.css" rel="stylesheet"/> <script src="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9hamF4Lmdvb2dsZWFwaXMuY29tL2FqYXgvlibs/jquery/3.1.0/jquery.min.js"></script> <script src="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9hamF4Lmdvb2dsZWFwaXMuY29tL2FqYXgvlibs/angularjs/1.0.7/angular.min.js"></script> </head> <body ng-app> </body> </html>
What we’ve done here is simply the main structure of an HTML document, all we’ve added is the linking of a custom CSS file which will contain some customization of the styling provided mainly by Bootstrap. Next up is Bootstrap itself: the linking of it’s main CSS file and the scripting of it’s Javascript file.
After that we have to script the Angular file, since it’s what our app will be based on, and also jQuery, since it’s instrumental in the proper functioning of Bootstrap.
Then we have included the ng-app
directive in the <body>
tag, which tells Angular that the code below should be evaluated as an Angular app, and it will be the one containing all our code.
2.2 Creating the navigation bar
Now comes what I like to call “the real part”, the one where we would actually be creating the navigation bar. Take a look at the code snippet below, and then we’ll analyze every line of it:
index.html
<nav class="{{active}}" ng-click="$event.preventDefault()"> //links here </nav>
First we create a section using the <nav>
tag that will contain the entirety of our navbar. Note that this tag is new for HTML5. You will notice that this tag has been awarded two attributes: class="{{active}}"
which means that the navigation menu will get the value of the “active” variable as a class, and ng-click="$event.preventDefault()"
. ng-click
is an Angular directive that let’s you specify a custom behavior when an element is clicked. in our case, this directive having the value $event.preventDefault()
means the $event.preventDefault()
stops the page from jumping when a link is clicked.
2.3 The triggers
After creating the outer shell of the navigation bar, we have to create all the triggers of the different locations we want our navigation to take us. The code for that would be pretty simple:
index.html
<button type="button" class="btn btn-warning btn-lg" href="#" ng-click="active='home'">Home</button> <button type="button" class="btn btn-success btn-lg" href="#" ng-click="active='about'">About</button> <button type="button" class="btn btn-info btn-lg" href="#" ng-click="active='contact'">Contact</button>
What you see here are three simple buttons, that are supposed to take you either to Home, to About or to Contact. As seen, the <button>
tag holds quite the number of attributes, and each of them has it’s importance in making it function just as we want it to. However, ng-click
is arguably the most important, at least to put the proper focus on what this button is supposed to do. When a link in the menu is clicked, we set the active variable, which is then used to display our choice.
The type="button"
and href="#"
are pretty explanatory. The one that might trip you up a bit is class
. The values awarded to it are related to Twitter’s Bootstrap, which means that they’re strictly styling. You can take them as is and see what they’re for by yourself, but we will explain them just in case!
They are all related to buttons, and obviously btn
represents just that. The btn-lg
means that we want our buttons to be large. It can either be lg
, md
, sm
and xs
, depending on what size you want the button to be. The rest are colors: btn-warning
is a shade of orange, btn-success
is green, and lastly btn-info
is blue. Nice, right? This is what we use Twitter’s bootstrap for: roughly 6 code words and you have all your styling set up for you!
With that, your navigation bar should be working fine and looking something similar to this:
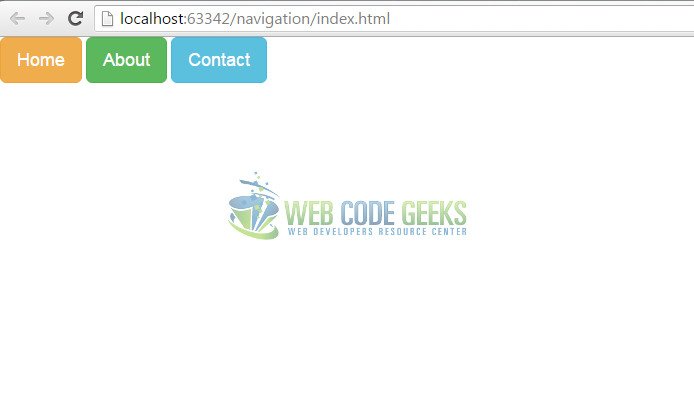
3. Fixing it up
3.1 The display
Even though our navigation bar is done and working, it’s difficult for a beginner to see that. Which is why we will build a display telling us what our choice was. The code would go like below:
index.html
<p ng-show="active">You chose <button type="button" class="btn btn-lg"><b >{{active}}</b></button> </p>
What we have here is a paragraph containing the custom Angular directive ng-show
whose main function is to show or hide the given HTML element based on the expression provided to the it. In our case, it will show the value given to active
, which as we mentioned above, will change every time we click one of the buttons. This value is styled into another button, this time gray, and whose content will be defined by the Angular expression {{active}}
. The rest is just par for the course.
3.2 Styling
While most of the styling is done using Twitter’s Bootstrap, every good app has some modifications of it’s own properties. In our case the most pressing one is the alignment of the button, as it’s be more visually appealing if we’d put them in the center, and also some other small modifications such as background colors and similar things. The code would look like below:
style.css
a:hover{ text-decoration:none; } nav{ display:inline-block; margin:60px auto 45px; background-color:#4d656e; box-shadow:0 1px 1px #ccc; border-radius:2px; } body{ font:15px/1.3 'Open Sans', sans-serif; color: #5e5b64; text-align:center; }
With that our “navigation app” would be complete and looking like below:
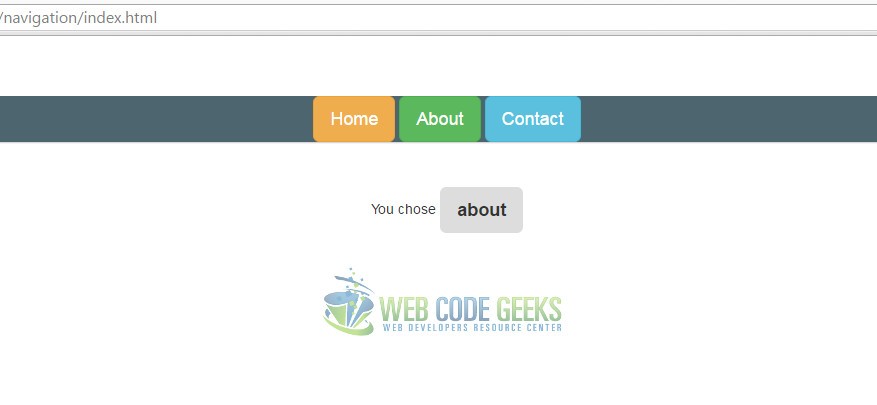
4. Download the source code
This was an example of navigation using AngularJS.
You can download the full source code of this example here: navigation